How to Add LazyVStack in iOS SwiftUI
In the world of SwiftUI, the LazyVStack view plays an essential role in creating flexible, efficient layouts. This blog post will provide a detailed explanation of this view and guide you through its usage, along with examples.
What is LazyVStack?
LazyVStack is a SwiftUI container view that arranges its child views in a vertical line, similar to VStack. The “Lazy” part refers to its optimization strategy.
SwiftUI will only render the views currently visible on the screen, which saves resources and boosts performance, especially when you have a large number of child views.
When to Use LazyVStack?
Use LazyVStack when you need a vertical stack of views that might be too large to fit into memory all at once. For instance, if you’re creating a list-like structure with custom views and you don’t know how many views it might contain, a LazyVStack can handle rendering those views efficiently as the user scrolls.
How to Use LazyVStack?
The implementation of LazyVStack is straightforward. Here’s a simple example:
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack(spacing: 20) {
ForEach(1...100, id: \.self) { index in
Text("Row \(index)")
.padding()
.frame(height: 50)
.background(Color.green)
.cornerRadius(10)
}
}
.padding()
}
}
}
In this example, the LazyVStack contains 100 Text views displaying the row numbers, each with a height of 50 and a background color of green. As you scroll through the views, SwiftUI only renders the ones that are currently visible on screen.
The ScrollView is required because LazyVStack itself doesn’t scroll. Inside the ScrollView, the LazyVStack has a spacing of 20 points between each of its children.
The ForEach loop creates the 100 Text views. The frame modifier sets the height for each view, and the background modifier gives it a green color. Lastly, the cornerRadius modifier rounds the corners of each view.
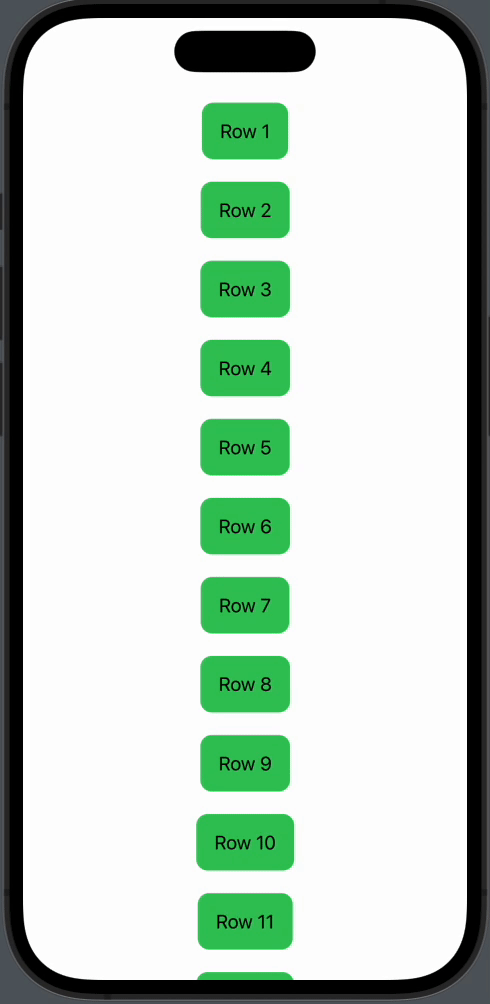
LazyVStack vs VStack
While VStack and LazyVStack both arrange child views vertically, the key difference lies in the “laziness” of the rendering. VStack will try to render all of its children immediately, which might lead to memory issues if you have a large number of views.
On the other hand, LazyVStack only creates the views that are visible, which can be a great advantage for performance when dealing with a large number of views.
LazyVStack is a powerful tool in SwiftUI, ideal for creating efficient vertical arrangements of views, especially when dealing with a large number of views. Understanding and utilizing this component effectively can greatly enhance your SwiftUI apps’ performance and efficiency.