How to Add Shadows to Shapes in SwiftUI
In UI design, shadows are not just decorative elements; they provide context and depth, helping to distinguish interface layers and improving user focus. SwiftUI offers a simple yet powerful system for adding shadows to shapes, which can greatly enhance the visual aesthetics of an app.
In this detailed blog post, we’ll explore how to apply shadows to shapes in SwiftUI and fine-tune them to achieve the desired effect.
SwiftUI’s .shadow
modifier applies a drop shadow to a view, which can create a raised or layered effect. Shadows can be applied to any view, but they are particularly effective when used with shapes to emphasize their borders and provide a sense of elevation.
Apply a Basic Shadow
Let’s start by applying a basic shadow to a circle:
struct ContentView: View {
var body: some View {
Circle()
.fill(Color.blue)
.frame(width: 100, height: 100)
.shadow(radius: 10)
}
}
In this code, the Circle
is given a shadow with a radius of 10 points. This shadow extends outward from the circle, creating a blurred effect that gives the impression of the circle floating above the background.
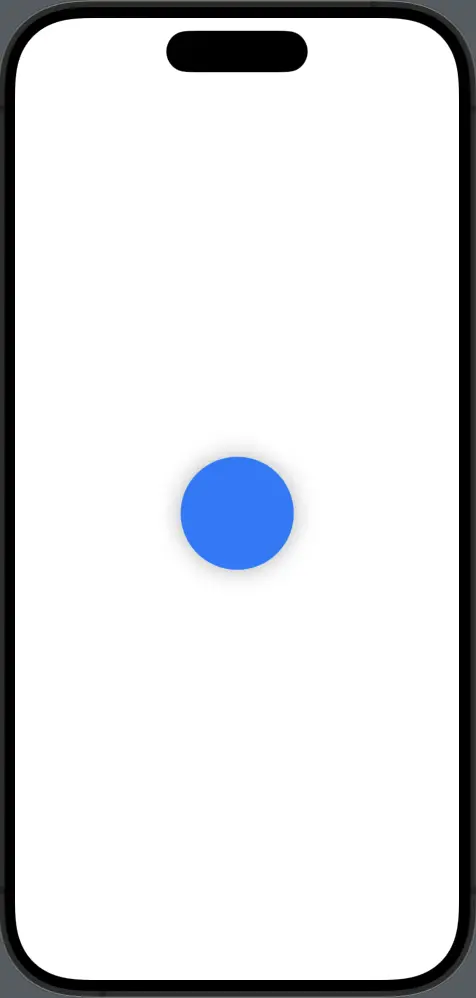
Customize Shadow Properties
The .shadow
modifier can be customized further by specifying the color, radius, and x/y offsets:
Rectangle()
.fill(Color.green)
.frame(width: 200, height: 100)
.shadow(color: .gray, radius: 5, x: 10, y: 10)
Here, we apply a shadow to a Rectangle
with a specific color (gray) and set the blur radius to 5 points. The offsets (x: 10, y: 10) shift the shadow to the right and downwards, enhancing the depth effect.
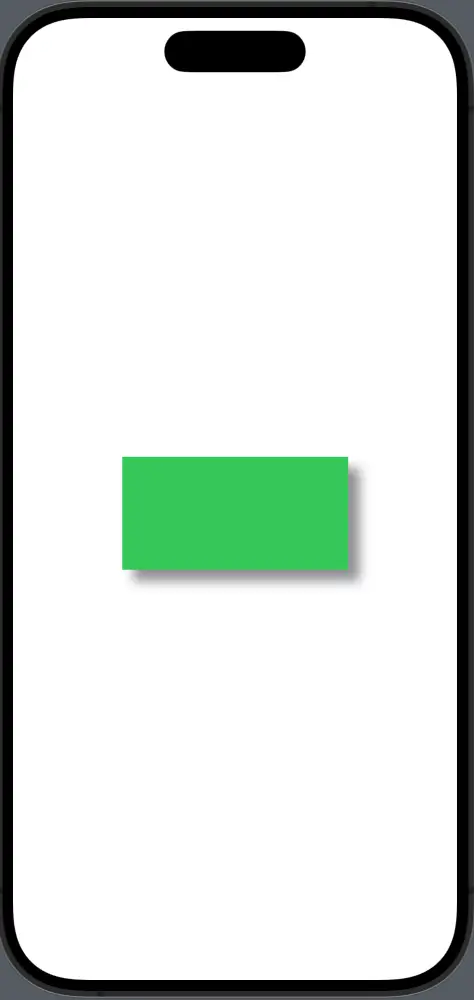
Multiple Shadows
SwiftUI allows you to apply multiple shadows to a view by adding several .shadow
modifiers. This can create a more complex and nuanced shadow effect:
Circle()
.fill(Color.red)
.frame(width: 100, height: 100)
.shadow(color: .black.opacity(0.5), radius: 10, x: 0, y: 5)
.shadow(color: .black.opacity(0.2), radius: 20, x: 10, y: 10)
In this example, two shadows with different opacities and offsets are added to a Circle
, creating a more dramatic and realistic shadow.
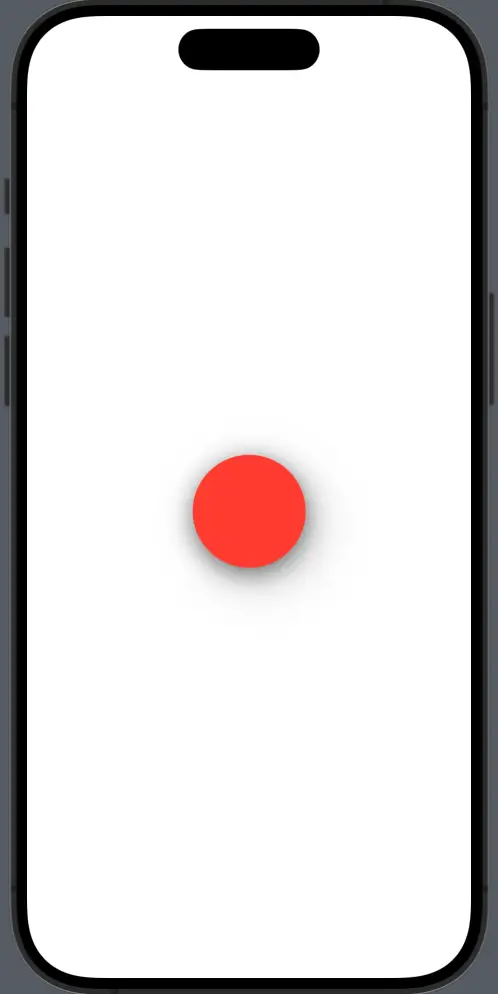
Shadows are a vital part of UI design, and SwiftUI’s .shadow
modifier provides a simple yet flexible way to add shadows to your shapes. Whether you’re looking for subtle depth, a realistic elevation effect, or dynamic, SwiftUI’s shadow capabilities can help you achieve your design goals.
Remember, while shadows can greatly enhance the look of your app, they should be used judiciously to avoid overwhelming the user interface. With the right balance, shadows can make your UI elements pop and provide a more intuitive experience.