How to Rotate Shapes in SwiftUI
SwiftUI is a robust framework that enables developers to craft fluid and dynamic interfaces with ease. One of the essential aspects of creating an engaging UI is the ability to control the rotation of shapes.
This blog post will delve into the mechanics of rotating shapes in SwiftUI, providing a comprehensive guide to implementing this in your app designs.
Rotation in SwiftUI can be applied to any view, including shapes, which allows you to create animated, interactive, and dynamic designs. The .rotationEffect
modifier is typically used to rotate shapes around a specified anchor point.
Basic Shape Rotation
To begin, let’s rotate a simple square shape:
struct ContentView: View {
var body: some View {
Rectangle()
.frame(width: 100, height: 100)
.rotationEffect(.degrees(45))
}
}
In this example, a Rectangle
is rotated 45 degrees using the .rotationEffect
modifier, which takes an Angle
as a parameter. The rotation is applied around the center of the rectangle by default.
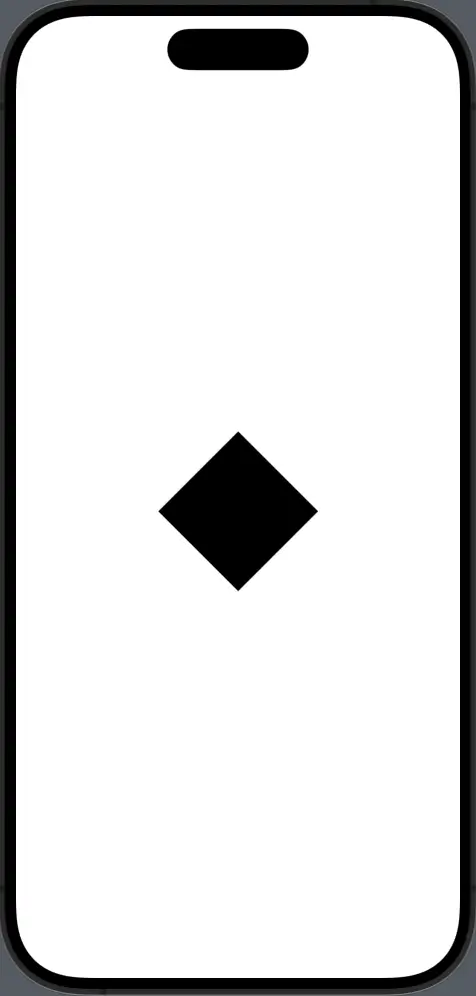
Rotate with a Specified Anchor
You might want to rotate a shape around a different point, such as one of its corners. SwiftUI allows you to specify an anchor for the rotation:
Rectangle()
.frame(width: 100, height: 100)
.rotationEffect(.degrees(45), anchor: .topLeading)
Now, the rectangle is rotated around its top-leading corner (the top-left corner), creating a different visual effect.
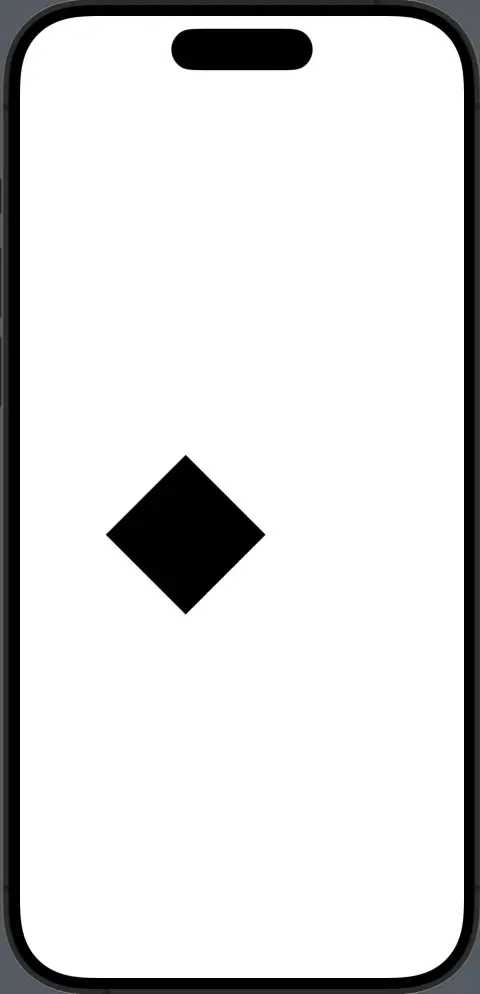
Animate Rotations
Animations add a layer of polish to your app. SwiftUI makes animating rotations straightforward:
@State private var rotationAngle: Angle = .degrees(0)
var body: some View {
Rectangle()
.frame(width: 100, height: 100)
.rotationEffect(rotationAngle)
.onTapGesture {
withAnimation {
rotationAngle += .degrees(90)
}
}
}
Here, the rotationAngle
state variable controls the rotation of the rectangle. When the rectangle is tapped, it animates a rotation by 90 degrees.
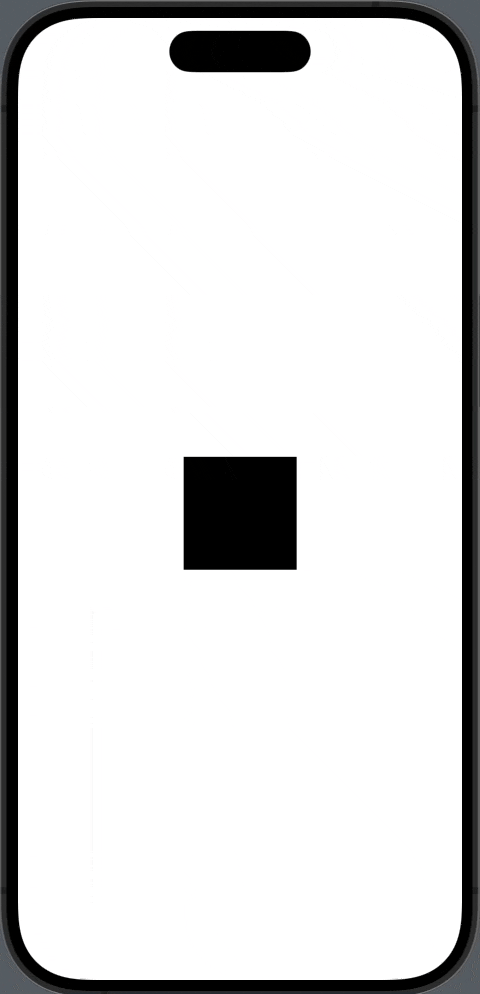
Shape rotation in SwiftUI is a powerful tool that can help create an interactive and dynamic user interface. Whether you need a simple rotation, a precise anchor point rotation, an animated or gesture-controlled rotation, SwiftUI provides the functionality to achieve this with minimal code.
By understanding these concepts and experimenting with the various rotation effects, you can add an extra layer of interactivity and polish to your app’s design. So go ahead, give your shapes a spin, and watch your app come to life!