How to Scale Shapes in SwiftUI
In SwiftUI, scaling shapes is an essential part of creating dynamic and responsive interfaces. Whether you’re adjusting the size of a button on different devices or creating a zoomable graphic, understanding how to scale shapes in SwiftUI is crucial.
This blog post will guide you through the process of scaling shapes, including maintaining aspect ratios, animating scale changes, and more.
Scaling a shape in SwiftUI is performed using the .scaleEffect
modifier. This modifier can scale a shape uniformly in both dimensions or scale it disproportionately to stretch it in a particular direction. Below, we’ll cover various scenarios where you might want to scale a shape and how to do it properly.
Uniform Scaling of Shapes
To scale a shape uniformly, maintaining its aspect ratio, you apply the .scaleEffect
modifier with a single scale factor.
import SwiftUI
struct ContentView: View {
var body: some View {
Circle()
.fill(Color.blue)
.scaleEffect(0.5)
.frame(width: 200, height: 200)
}
}
In this example, a Circle
shape is scaled down to half its size while maintaining its circular form. The frame
sets the space allocated for the view, and the circle scales to fit within this space.
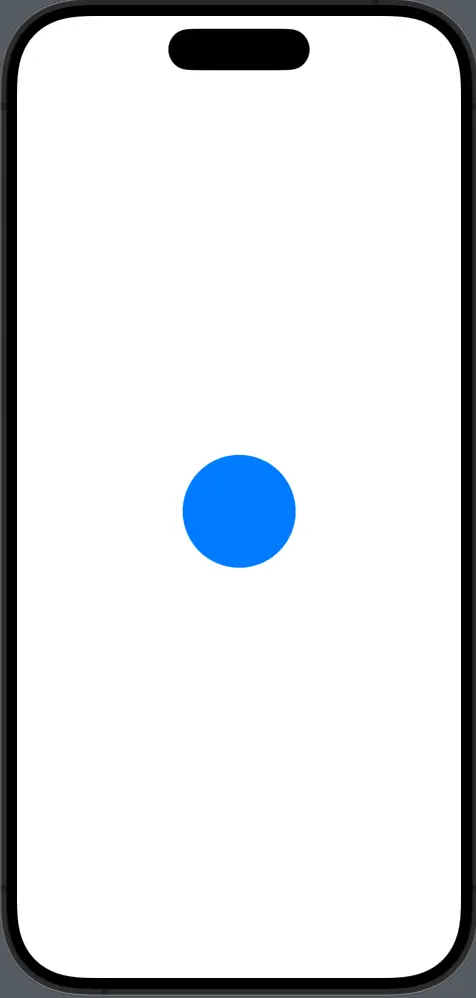
Non-Uniform Scaling
There are times when you might need to scale a shape’s width and height by different amounts. You can do this by passing separate x and y scale factors to the .scaleEffect
modifier.
Rectangle()
.fill(Color.green)
.scaleEffect(x: 1, y: 0.5)
.frame(width: 200, height: 200)
Here, a Rectangle
is scaled to its original width but half its height, resulting in a stretched effect.
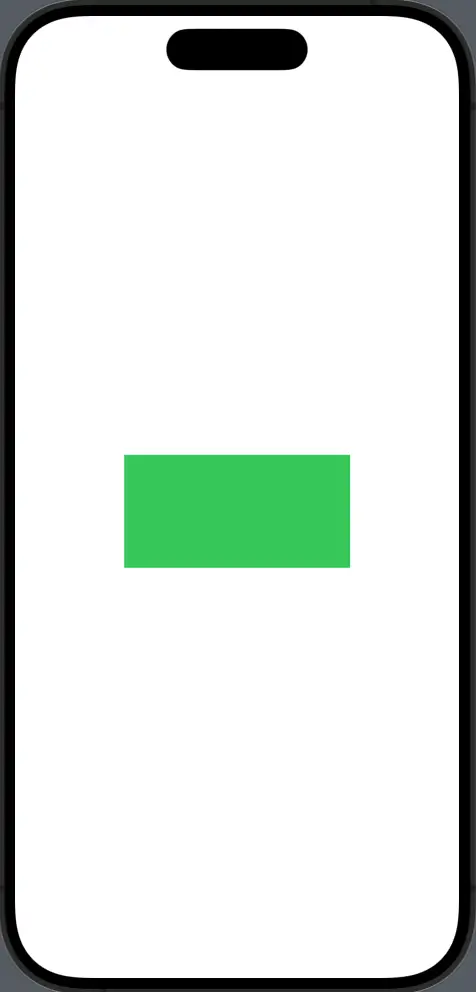
Animating Scale Changes
Animating a shape’s scaling can add a layer of interactivity to your UI. Here’s how you can animate the scaling of a shape when a user taps on it.
struct ContentView: View {
@State private var scaleFactor: CGFloat = 1
var body: some View {
Circle()
.fill(Color.red)
.scaleEffect(scaleFactor)
.frame(width: 200, height: 200)
.onTapGesture {
withAnimation {
scaleFactor = scaleFactor == 1 ? 2 : 1
}
}
}
}
Tapping on the Circle
toggles its scale between its original size and double its size with a smooth animation.
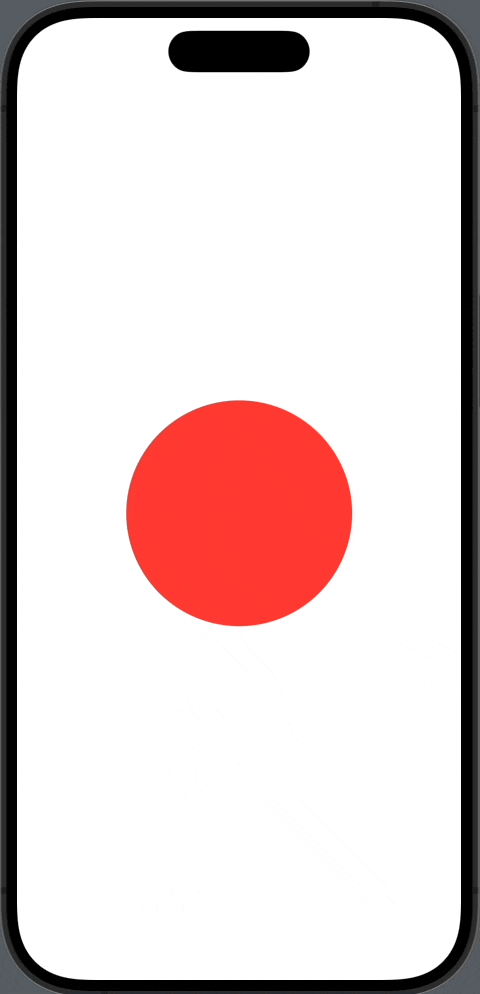
Scaling shapes in SwiftUI is a powerful feature that can help you build a responsive and engaging UI. Whether you need uniform scaling for icons, non-uniform scaling for creative design, or responsive scaling for different device sizes, SwiftUI provides the tools to achieve this with minimal effort.
Remember to always test your scaling on various devices and consider the usability implications of your design choices. With thoughtful application, scaling can enhance the user experience by providing visual feedback, drawing attention, and adapting the interface to different environments.