How to Manage LazyVStack Spacing in iOS SwiftUI
Welcome to another SwiftUI tutorial where we dive deep into a specific feature. In this blog post, our focus is on the SwiftUI view called LazyVStack, particularly its spacing attribute. If you’re a mobile app developer looking to enhance your SwiftUI knowledge, this post is for you!
LazyVStack Overview
LazyVStack is a SwiftUI view that arranges its children in a vertical line, creating its contents just before they are displayed on screen. This “lazy” rendering method is a performance optimization, as it reduces the processing load when dealing with a large number of items.
Spacing in LazyVStack
One key attribute of a LazyVStack is its ‘spacing’ parameter. This value determines the vertical space between the items within the stack. The spacing is set to nil by default, which means the system will choose an appropriate spacing based on the context.
However, you can specify a custom value to get the exact spacing you desire.
LazyVStack Spacing Example
Let’s dive into a simple SwiftUI example that uses LazyVStack and a custom spacing.
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack(spacing: 20) {
ForEach(1...100, id: \.self) { index in
Text("Row \(index)")
.frame(maxWidth: .infinity, minHeight: 50)
.background(Color.yellow)
.border(Color.orange, width: 1)
}
}
}
}
}
In this example, we’re creating a vertical list of 100 rows with LazyVStack. Each row contains a Text view displaying its row number. We’ve set the ‘spacing’ parameter to 20, so there will be a 20-point gap between each row.
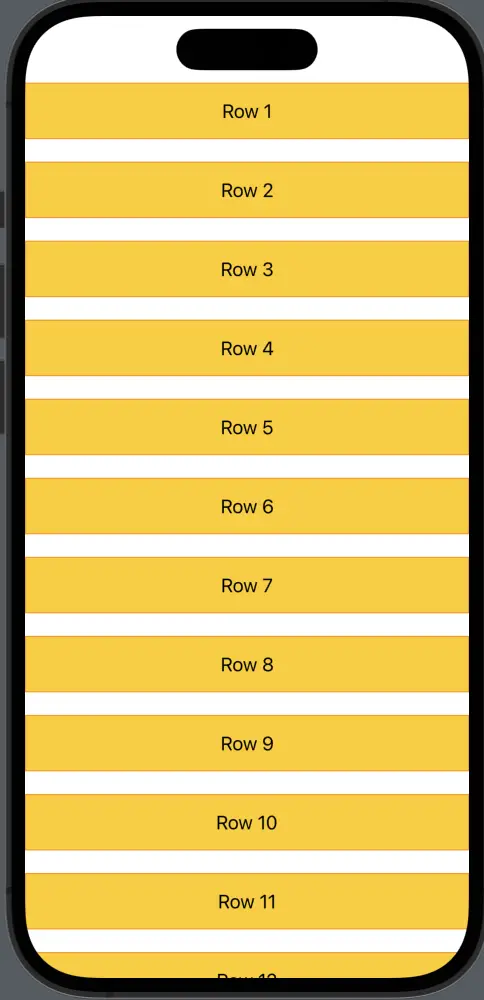
Tips for Using Spacing in LazyVStack
Here are a few tips to keep in mind when working with spacing in LazyVStack:
- Mind the Default Spacing: If you don’t provide a specific spacing, SwiftUI applies a default spacing based on the context. This might not always be what you want, so it’s often best to specify your own spacing value.
- Consider the Context: The spacing should fit the overall design of your app. Make sure the spacing value complements other elements on the screen.
- Performance Optimization: Remember, LazyVStack creates views as they become visible. This is efficient when dealing with large data sets.
LazyVStack and its spacing attribute are crucial to creating efficient and well-designed SwiftUI applications. By understanding how to use this feature, you can create dynamic vertical lists with customized spacing.