How to Implement LazyHStack Alignment in iOS SwiftUI
SwiftUI has brought simplicity and flexibility to app development. Among its many features, LazyHStack provides developers with the ability to create horizontally-scrolling stacks efficiently. This post will focus on the alignment options available in SwiftUI’s LazyHStack.
LazyHStack Overview
LazyHStack is a SwiftUI view that arranges child views horizontally, only rendering the views that are currently on-screen. It allows you to present a list of elements horizontally while optimizing performance. The alignment feature lets you control how the children are positioned vertically within the stack.
Alignment in LazyHStack
The alignment parameter in LazyHStack determines the vertical alignment of child views. There are several predefined alignment options available, such as:
top
center
bottom
firstTextBaseline
lastTextBaseline
You can also create custom alignments based on your specific needs.
Top Alignment
Aligning children to the top of the LazyHStack:
LazyHStack(alignment: .top) {
// Child views
}
Center Alignment
Aligning children to the center of the LazyHStack:
LazyHStack(alignment: .center) {
// Child views
}
Bottom Alignment
Aligning children to the bottom of the LazyHStack:
LazyHStack(alignment: .bottom) {
// Child views
}
Complete Example
Below is a complete example using LazyHStack with different alignments. This example showcases four text views with different font sizes within a LazyHStack, and how the alignment affects their vertical positioning.
struct ContentView: View {
var body: some View {
VStack(spacing: 20) {
LazyHStack(alignment: .top) {
Text("Top").font(.largeTitle)
Text("Alignment").font(.headline)
Text("In").font(.subheadline)
Text("LazyHStack").font(.footnote)
}
.background(Color.gray.opacity(0.2))
LazyHStack(alignment: .center) {
Text("Center").font(.largeTitle)
Text("Alignment").font(.headline)
Text("In").font(.subheadline)
Text("LazyHStack").font(.footnote)
}
.background(Color.gray.opacity(0.2))
LazyHStack(alignment: .bottom) {
Text("Bottom").font(.largeTitle)
Text("Alignment").font(.headline)
Text("In").font(.subheadline)
Text("LazyHStack").font(.footnote)
}
.background(Color.gray.opacity(0.2))
}
.padding()
}
}
Output:
- Top Alignment: All text views align to the top of the largest text view.
- Center Alignment: The texts are vertically centered within the LazyHStack.
- Bottom Alignment: The texts align to the bottom of the largest text view.
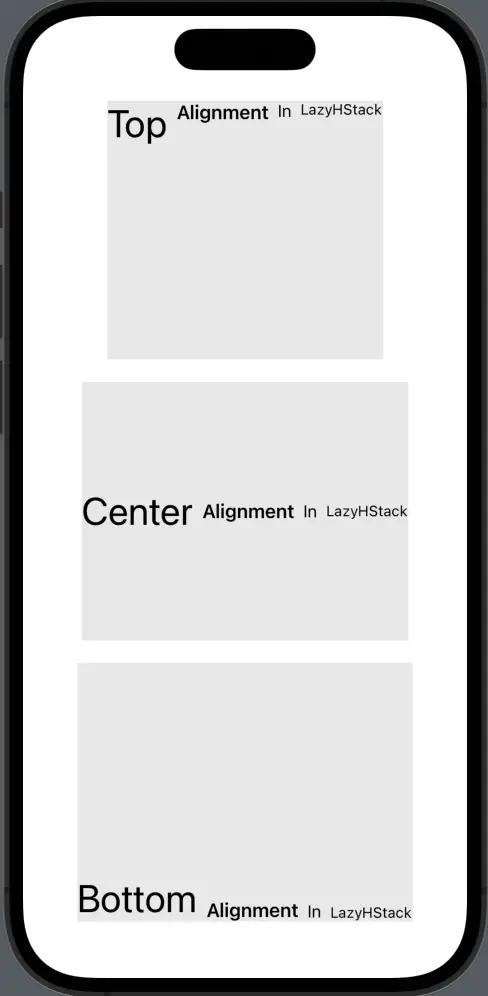
Conclusion
SwiftUI’s LazyHStack alignment options bring powerful control to horizontal layouts. The various predefined alignments provide developers with the tools to create visually appealing and well-organized designs.