How to Add Title to ConfirmationDialog in iOS SwiftUI
Confirmation dialogs are essential UI elements that help capture user decisions, especially for critical actions like deleting a file. In SwiftUI, creating a confirmation dialog is straightforward, but what if you want to make sure the title is always visible?
In this blog post, we’ll focus on how to add a title to a ConfirmationDialog
in iOS SwiftUI.
What is a ConfirmationDialog?
A ConfirmationDialog
in SwiftUI is a dialog box that appears to confirm an action. It usually contains a title, a message, and one or more buttons to accept or cancel the action.
Add a Title to ConfirmationDialog
The Code
Here’s a simple example that demonstrates how to add a title to a ConfirmationDialog
.
import SwiftUI
struct ContentView: View {
@State private var showConfirmation = false
var body: some View {
Button("Delete File") {
showConfirmation = true
}
.confirmationDialog("Are you sure?", isPresented: $showConfirmation, titleVisibility: Visibility.visible, actions: {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}){
Text("You are about to delete a file. This action cannot be undone.")
}
}
}
Explanation of the Code
Let’s break down the code to understand it better.
State Variable
@State private var showConfirmation = false
We declare a state variable showConfirmation
to control the visibility of the ConfirmationDialog
.
Trigger Button
Button("Delete File") {
showConfirmation = true
}
We have a button labeled “Delete File.” When this button is clicked, it sets showConfirmation
to true, which triggers the dialog to appear.
The ConfirmationDialog
.confirmationDialog("Are you sure?", isPresented: $showConfirmation, titleVisibility: Visibility.visible, actions: {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}){
Text("You are about to delete a file. This action cannot be undone.")
}
The confirmationDialog
is attached to the button using a modifier. The titleVisibility: Visibility.visible
parameter ensures that the title is always visible. Inside the dialog, we have two buttons for “Yes, Delete it” and “Cancel,” along with a message text.
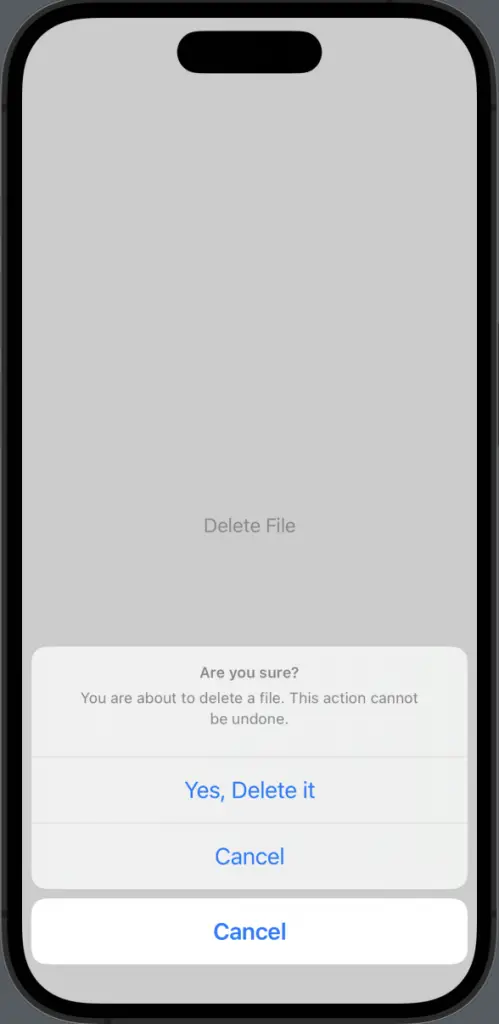
Adding a title to a ConfirmationDialog
in SwiftUI is as simple as using the titleVisibility: Visibility.visible
parameter. This ensures that the title is always visible, providing a clearer context for the user action.