How to Make HStack Scrollable in iOS SwiftUI
SwiftUI’s HStack is a go-to tool for many developers who want to group and align views horizontally. But what happens when you have more elements than what can fit on the screen? In this blog post, we’ll walk through making a SwiftUI HStack scrollable.
HStack Overview
An HStack is a simple, straightforward SwiftUI structure that positions child views on a horizontal line. However, without additional modifications, an HStack doesn’t automatically scroll if it contains too many views to fit on the screen. That’s where the ScrollView comes into play.
Make HStack Scrollable with ScrollView
ScrollView is a SwiftUI view that scrolls its contents. Wrapping an HStack within a ScrollView makes it possible to scroll through all the views inside the HStack.
Step 1: Create an HStack
Start by creating an HStack. Let’s add several Color views for demonstration purposes.
HStack {
ForEach(0..<20) { index in
Color.red
.frame(width: 50, height: 50)
.overlay(Text("\(index)").foregroundColor(.white))
}
}
Step 2: Wrap HStack in a ScrollView
Next, enclose your HStack inside a ScrollView. This will make the HStack scrollable.
ScrollView(.horizontal) {
HStack {
ForEach(0..<20) { index in
Color.red
.frame(width: 50, height: 50)
.overlay(Text("\(index)").foregroundColor(.white))
}
}
}
In the above code, we added .horizontal
to the ScrollView initializer, which means the content will scroll from left to right.
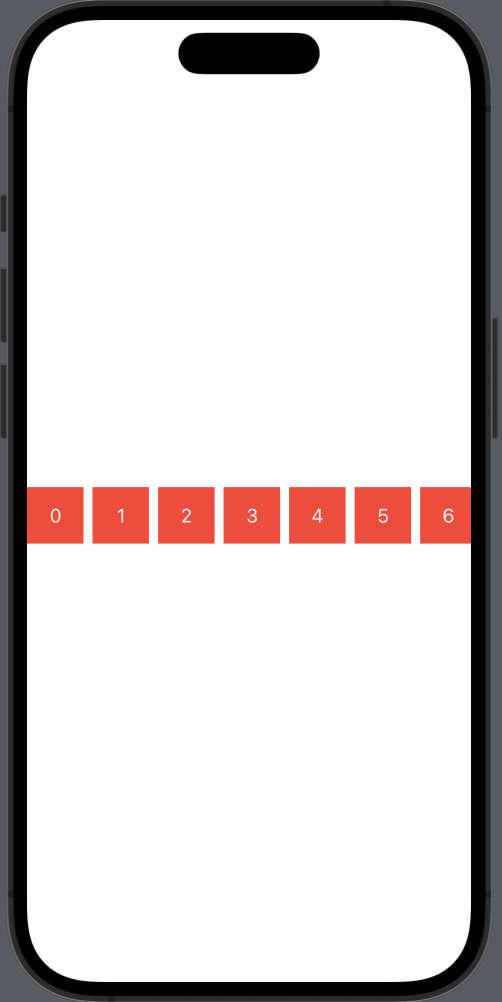
Now you know how to make an HStack scrollable using ScrollView in SwiftUI. This feature can enhance user experience by offering better navigation when dealing with many child views.