How to Add ZStack in iOS SwiftUI
SwiftUI, Apple’s innovative UI toolkit, has dramatically simplified app development. One feature at the forefront of this tool is ZStack. This blog post explores the concept and usage of ZStack in SwiftUI.
What is a ZStack?
ZStack is a SwiftUI container that stacks its child views along the z-axis. This means it overlays its children, with each new child view layered on top of the previous ones. ZStack allows us to create layered interfaces with relative ease.
Basic ZStack Example
To illustrate, let’s create a simple ZStack with three Circle views, each with a different color.
ZStack {
Circle().fill(Color.red).frame(width: 200, height: 200)
Circle().fill(Color.yellow).frame(width: 150, height: 150)
Circle().fill(Color.green).frame(width: 100, height: 100)
}
This code places three circles on top of each other, with the green circle on top, followed by the yellow and then the red.
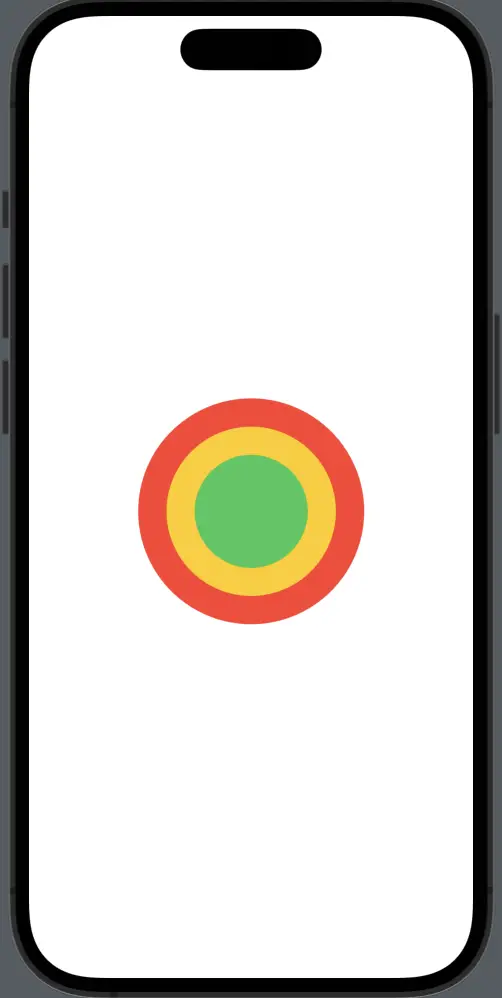
Positioning with ZStack
ZStack is not just for overlaying views. Combined with the .offset
modifier, it allows for powerful positioning. Each child view can be offset from the center of the ZStack, enabling complex layouts.
For example:
ZStack {
Circle().fill(Color.red).frame(width: 200, height: 200)
Circle().fill(Color.yellow).offset(x: 20, y: 20).frame(width: 150, height: 150)
Circle().fill(Color.green).offset(x: 40, y: 40).frame(width: 100, height: 100)
}
In this code, the yellow circle is offset by 20 points in both directions from the center, and the green circle is offset by 40 points.
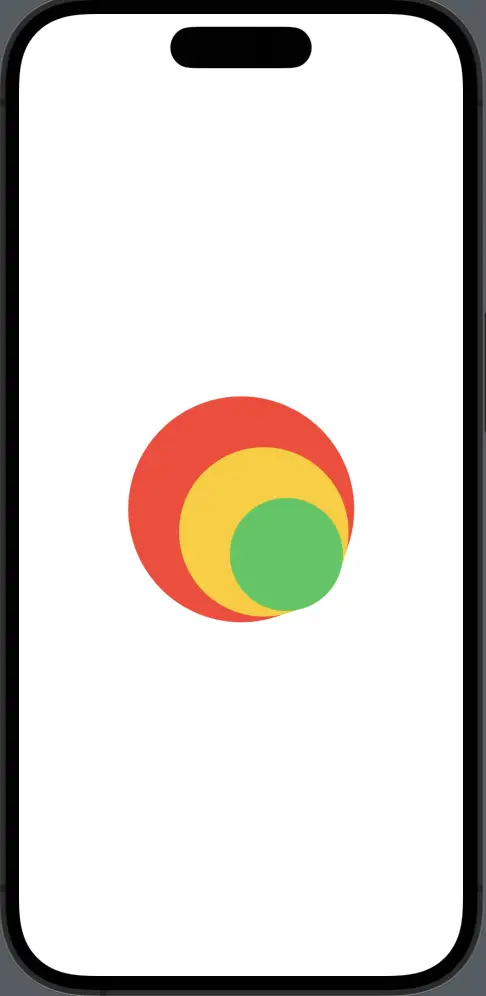
Implement Background Images using ZStack
ZStack is also incredibly handy when you need to set a full-screen background image. For instance:
ZStack {
Image("clouds")
.resizable()
.edgesIgnoringSafeArea(.all)
Text("Hello, World!")
}
In this code snippet, the background image expands to fill the screen, with the text “Hello, World!” overlaid on top.
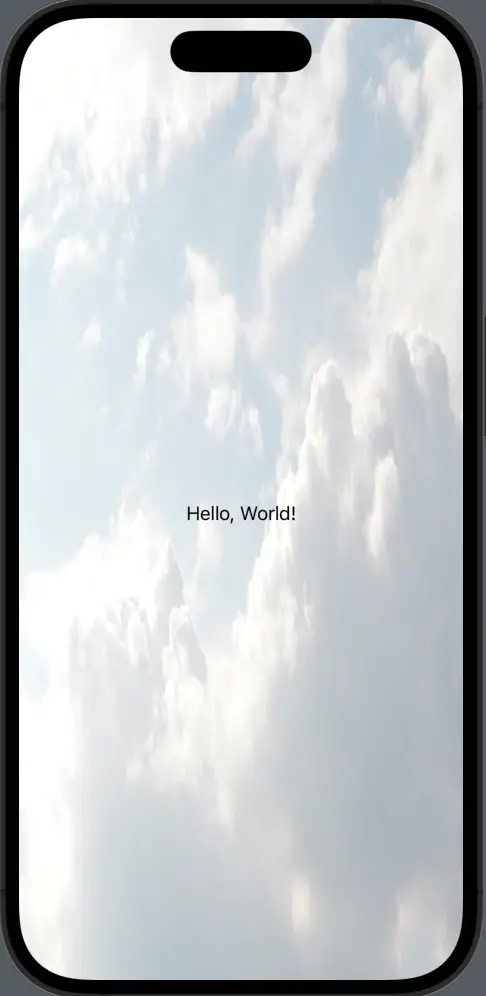
The ZStack in SwiftUI is a powerful tool for developers, offering options for complex, layered designs. Combined with other SwiftUI views and modifiers, ZStack becomes an indispensable part of any SwiftUI developer’s toolkit.