How to Add Toggle Without Label in iOS SwiftUI
Toggles are a common UI element used to switch between two states, typically on and off. In SwiftUI, adding a toggle with a label is straightforward. But what if you want a toggle without a label? In this blog post, we’ll explore how to achieve this using the labelsHidden()
modifier.
The Basic Toggle with Label
First, let’s look at the default way to create a toggle with a label in SwiftUI:
import SwiftUI
struct ContentView: View {
@State private var isOn = true
var body: some View {
Toggle("Switch Me", isOn: $isOn)
}
}
This will display a toggle with the label “Switch Me” next to it.
Use labelsHidden()
Modifier
To remove the label, you can use the labelsHidden()
modifier. Here’s the example code:
import SwiftUI
struct ContentView: View {
@State private var isOn = true
var body: some View {
Toggle("Switch Me", isOn: $isOn).labelsHidden()
}
}
By adding .labelsHidden()
at the end, the label “Switch Me” will be hidden, leaving only the toggle switch.
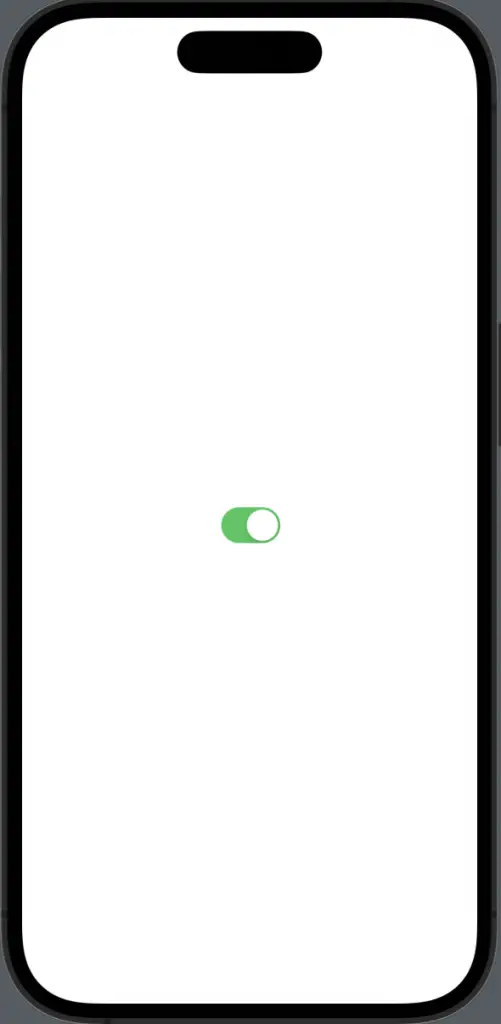
Why Use a Toggle Without a Label?
There are several scenarios where a label-free toggle is useful:
- To save screen space.
- When the toggle’s function is obvious.
- When the UI design calls for minimalism.
Removing the label from a toggle in SwiftUI is as simple as adding the labelsHidden()
modifier. This can be useful for various design needs and to make the UI cleaner or more intuitive.