How to Add LazyVStack with Section in iOS SwiftUI
SwiftUI is a declarative framework that allows developers to build user interfaces across all Apple platforms. In this blog post, we’ll be exploring one of its unique constructs: the combination of LazyVStack and Section.
By understanding and applying these concepts, you can enhance the structure and performance of your SwiftUI apps.
SwiftUI LazyVStack
LazyVStack is a SwiftUI view that arranges its child views in a vertical line. Similar to LazyVGrid, LazyVStack also loads its content lazily. This means it only creates views as they become visible, making it highly efficient for large collections of data.
Here’s a basic LazyVStack example:
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
ForEach(1...1000, id: \.self) { number in
Text("Row \(number)")
}
}
}
}
}
This LazyVStack generates a thousand rows of text, but due to its lazy nature, only the views visible on screen are created.
Combine LazyVStack with Section
Section is another SwiftUI view used to group content, usually within a list or another container. Sections make it easier to organize and manage code, especially when dealing with complex user interfaces. A Section can also have a header and footer.
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
Section(header: Text("Section 1").font(.largeTitle)) {
ForEach(1...20, id: \.self) { number in
Text("Row \(number)")
}
}
Section(header: Text("Section 2").font(.largeTitle)) {
ForEach(21...40, id: \.self) { number in
Text("Row \(number)")
}
}
}
}
}
}
In this example, we use a LazyVStack within a ScrollView to create two sections. Each section header is labeled, and each section contains a specific range of rows. This helps organize the data in a logical, easily readable format.
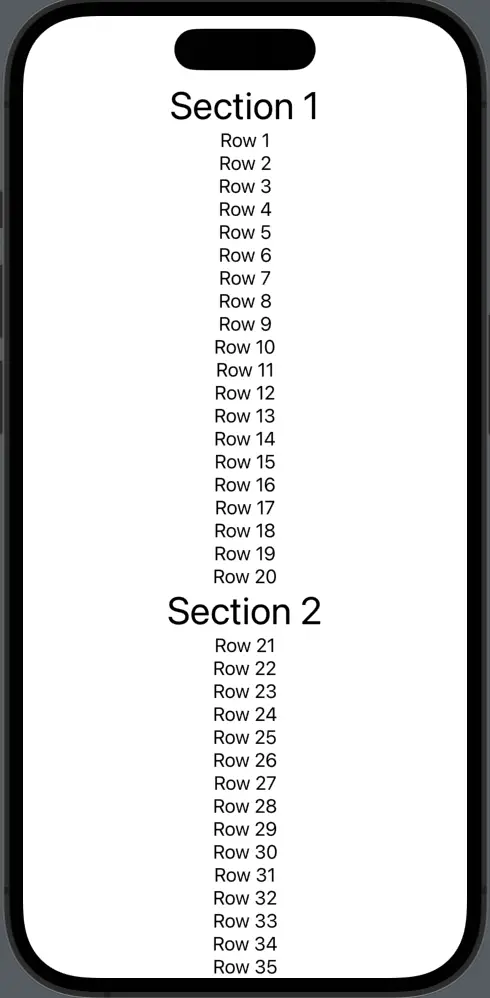
Benefits of Using LazyVStack with Section
The main benefit of using LazyVStack with Section is that it provides a structured and organized way to present data. This combination lets you segment large sets of data into logical chunks, making it easier for users to understand and navigate through the content.
Moreover, because LazyVStack only loads views as they become visible, it’s a great tool for enhancing performance in SwiftUI apps, especially when working with large amounts of data.
Mastering the use of LazyVStack and Section views in SwiftUI can significantly enhance your app’s structure and performance. These tools provide a great way to create organized, efficient, and highly readable interfaces.