How to Make ZStack Fill Entire Screen in iOS SwiftUI
In SwiftUI, ZStack is a vital tool for creating layered interfaces. In this guide, we’ll learn how to make a ZStack fill the entire screen and use a background color to visualize the results.
What is ZStack?
ZStack is a SwiftUI container view that overlays its child views along the z-axis. It is incredibly versatile, but to fully leverage it, understanding how to manipulate its dimensions is critical.
Fill the Screen with ZStack
To fill the entire screen with a ZStack, we use the .frame
modifier, specifying .infinity
for maxWidth
and maxHeight
.
Step 1: Create a ZStack
Firstly, define your ZStack. To illustrate, we will include a Text view.
ZStack {
Text("This is ZStack")
}
Step 2: Apply .frame Modifier
Next, apply the .frame
modifier to your ZStack. Here, you set maxWidth
and maxHeight
to .infinity
.
ZStack {
Text("This is ZStack")
}.frame(maxWidth: .infinity, maxHeight: .infinity)
This tells SwiftUI that the ZStack should take up as much space as possible, thus filling the entire screen.
Step 3: Set a Background Color
To ensure your ZStack is truly occupying the full screen, you can set a background color. We will use .edgesIgnoringSafeArea(.all)
to allow the background to extend into the safe area.
ZStack {
Text("This is ZStack")
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(Color.yellow.edgesIgnoringSafeArea(.all))
The screen should now be completely filled with the red color, thus indicating the ZStack is also filling the entire screen.
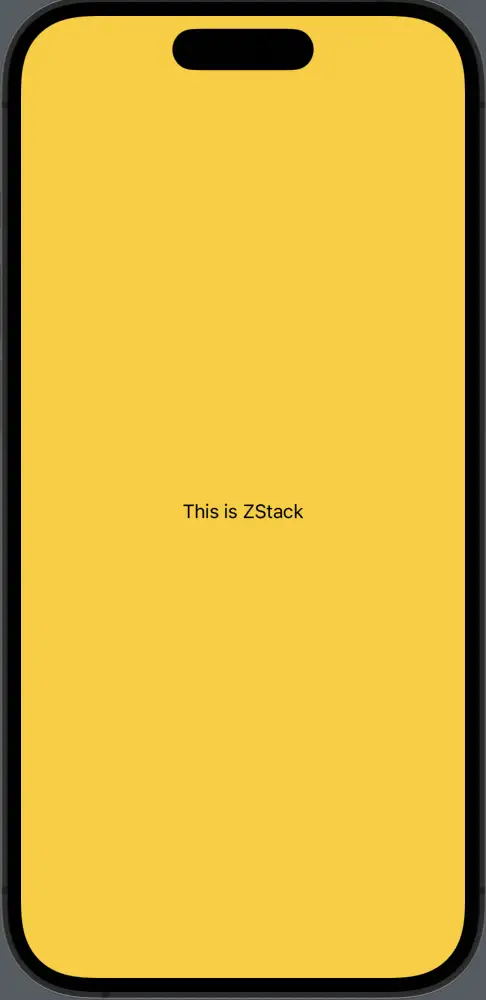
Following is the complete code for reference.
import SwiftUI
struct ContentView: View {
var body: some View {
ZStack {
Text("This is ZStack")
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(Color.yellow.edgesIgnoringSafeArea(.all))
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
The ZStack in SwiftUI provides a powerful means to design layered user interfaces. By using the .frame
modifier and specifying .infinity
for maxWidth
and maxHeight
, you can make a ZStack fill the entire screen. The addition of a background color helps confirm the results visually.