How to Create Different Shapes in SwiftUI
Creating shapes in SwiftUI can add a lot of visual interest and a professional look to your iOS apps. In this post, I’m going to guide you through the basics of shape drawing in SwiftUI.
We will explore the built-in shapes, how to customize them, and how to combine them to create complex designs.
Built-In Shapes
SwiftUI comes with a number of built-in shapes such as Rectangle, Circle, Ellipse, Capsule, and more. They are easy to use and can be drawn by simply initializing them and adding them to your view hierarchy.
Rectangle()
.fill(Color.blue)
.frame(width: 100, height: 100)
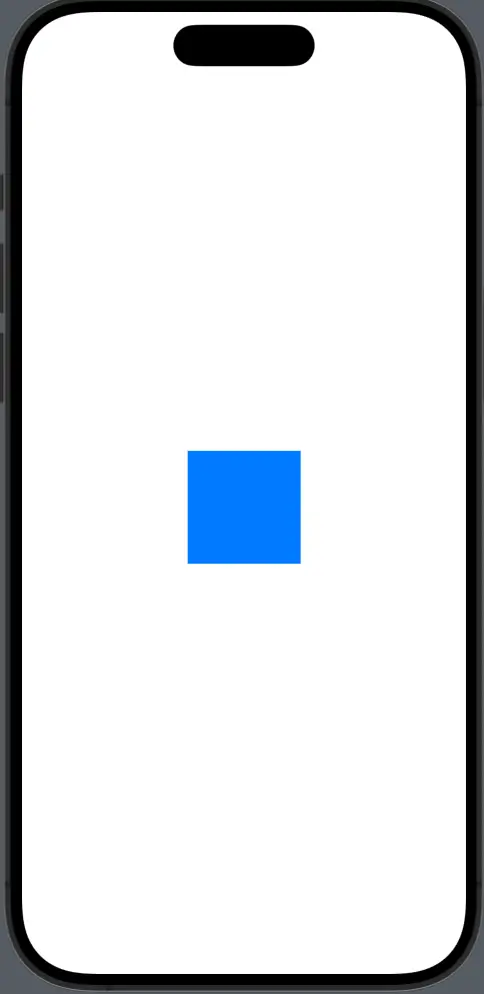
Customize Shapes
You can easily customize these shapes by applying modifiers. For example, you can change the color, size, and add borders.
Circle()
.stroke(Color.red, lineWidth: 5)
.frame(width: 50, height: 50)
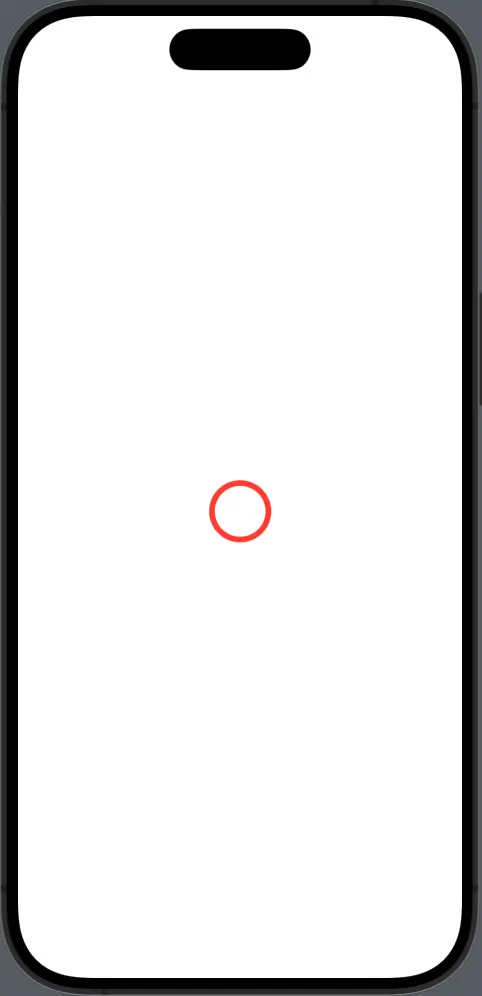
Combine Shapes
You can also combine multiple shapes to create more complex designs. By using the ZStack
layout, you can layer shapes on top of each other.
ZStack {
Rectangle()
.fill(Color.green)
.frame(width: 200, height: 100)
Circle()
.fill(Color.blue)
.frame(width: 100, height: 100)
}
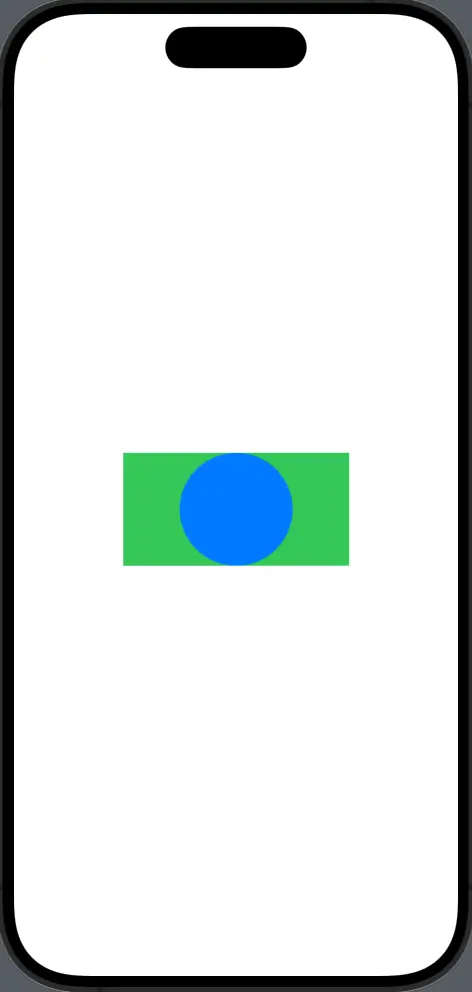
Create Custom Shapes
For more unique designs, you can create custom shapes by conforming to the Shape
protocol. You define your own path within the path(in:)
method.
import SwiftUI
struct ContentView: View {
var body: some View {
Triangle()
.fill(Color.purple)
}
}
struct Triangle: Shape {
func path(in rect: CGRect) -> Path {
var path = Path()
path.move(to: CGPoint(x: rect.midX, y: rect.minY))
path.addLine(to: CGPoint(x: rect.minX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY))
path.closeSubpath()
return path
}
}
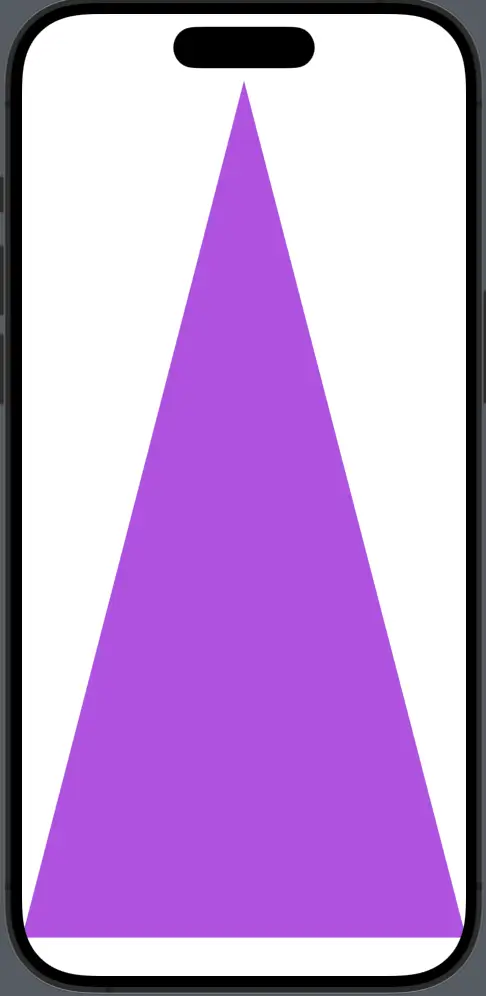
Drawing shapes in SwiftUI is both fun and powerful. Start with built-in shapes, customize them with modifiers, combine them to make more complex structures and create custom shapes with the Shape
protocol.
By following this guide, you can integrate shapes into your SwiftUI apps to enhance the user interface. Experiment with different combinations and animations to see what creative designs you can come up with!