How to Rotate Image in iOS SwiftUI
In this blog post, we will venture into the realm of image manipulation in SwiftUI, focusing on one of the most widely used image transformations – rotation. Whether it’s for an engaging UI animation or merely enhancing the aesthetic appeal of your app, understanding how to rotate images will certainly come in handy.
Basics: Rotate an Image
SwiftUI provides a simple yet powerful modifier – .rotationEffect()
, which you can use to rotate an image. Here’s an example:
Image("yourImage")
.resizable()
.frame(width: 300,height: 200)
.rotationEffect(.degrees(45))
In this code snippet, an image is rotated by 45 degrees clockwise. The .degrees(_:)
function is used to specify the angle of rotation in degrees.
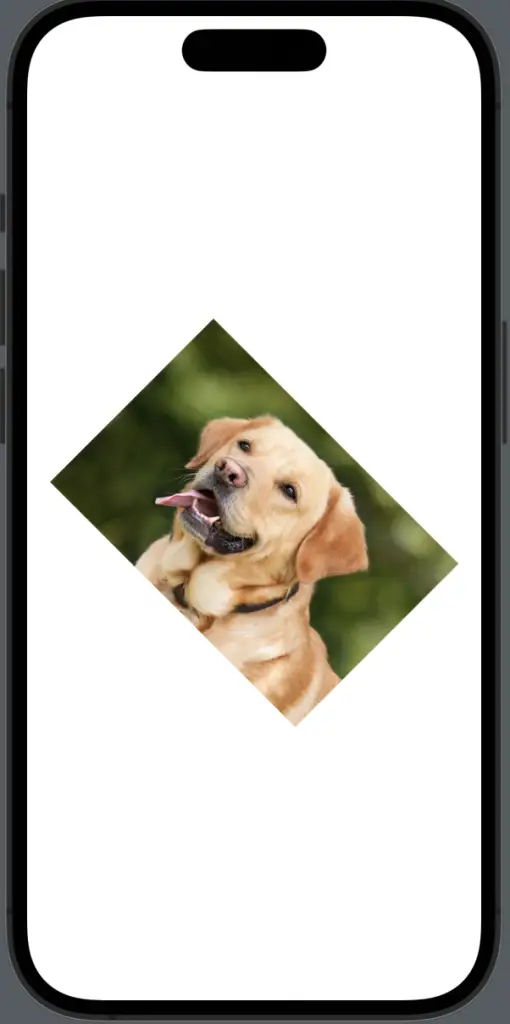
Rotate Around a Point
By default, SwiftUI rotates views around their center point. However, you can specify a different point of rotation using the anchor
parameter of the .rotationEffect()
modifier:
Image("dog")
.resizable()
.frame(width: 300,height: 200)
.rotationEffect(.degrees(45), anchor: .topLeading)
Here, the image rotates 45 degrees around its top leading corner.
Animate Image Rotation
SwiftUI makes it effortless to animate image rotation:
struct ContentView: View {
@State private var isRotated = false
var body: some View {
Image("dog")
.resizable()
.scaledToFit()
.rotationEffect(.degrees(isRotated ? 180 : 0))
.onTapGesture {
withAnimation(.easeInOut(duration: 1)) {
self.isRotated.toggle()
}
}
}
}
In this example, the image rotates 180 degrees when tapped, with the rotation animated over a duration of one second. The withAnimation
function is used to apply an animation to the rotation.
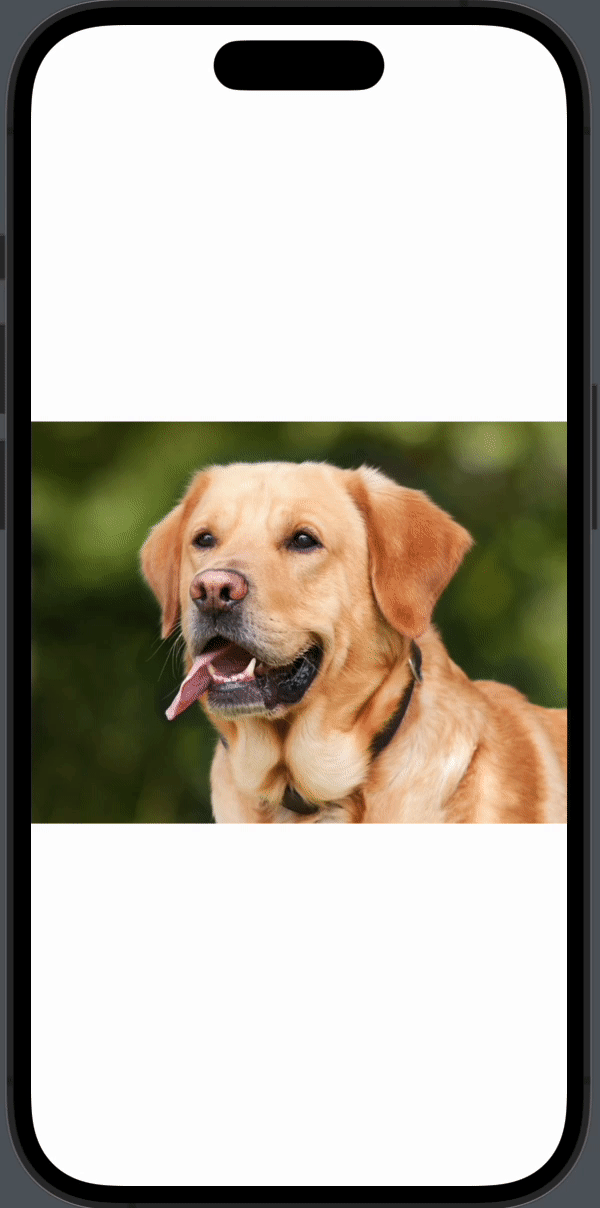
Being able to rotate images in SwiftUI is an invaluable tool in your arsenal to create visually captivating and interactive user interfaces. From basic rotations to animations, SwiftUI offers a powerful and simple way to manipulate images in your application.