How to Set VStack Background Color in iOS SwiftUI
Creating beautiful and interactive user interfaces is an important aspect of iOS app development. SwiftUI, Apple’s declarative UI framework, offers a variety of layout structures and styling options that make this task easier and more efficient.
One such styling feature is the ability to change the background color of a VStack. In this post, we’ll take a deep dive into how to set the SwiftUI VStack background color.
VStack in SwiftUI
VStack, short for Vertical Stack, is a type of layout in SwiftUI. As the name suggests, VStack stacks its children views vertically. By default, VStack occupies the minimum space required to display its content, but it can also expand to fill the available space when required.
Set the Background Color of a VStack
Styling is a crucial aspect of building appealing user interfaces. SwiftUI provides simple and intuitive ways to apply styles to our views. Adding a background color to VStack involves the use of the background
modifier. Here’s an example:
struct ContentView: View {
var body: some View {
VStack() {
Text("Hello")
Text("World")
}.frame(maxWidth: .infinity, maxHeight: .infinity)
.background(.green)
}
}
In this example, “Hello” and “World” are stacked vertically, and a green background is applied to the VStack.
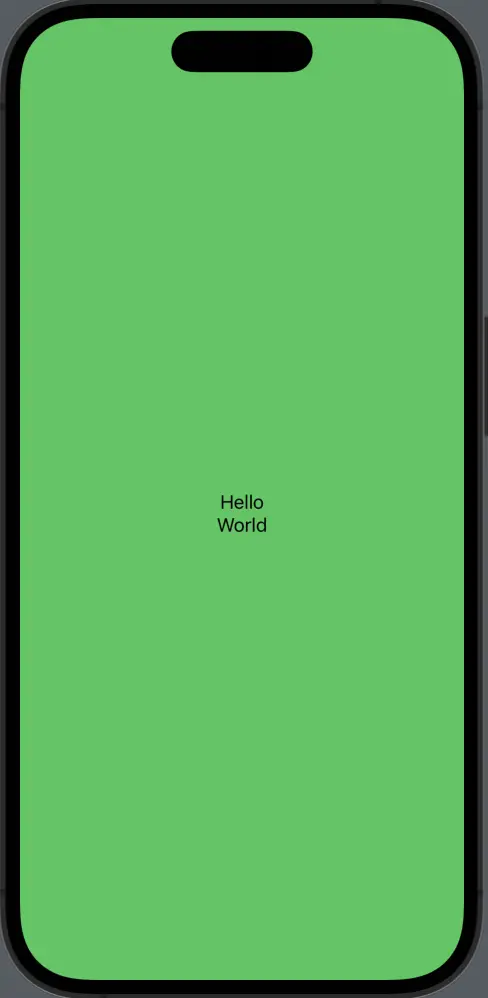
The .background Modifier
The .background
modifier is used to set the background of a view. In our case, we use it to set the background color of the VStack. You can pass various arguments to this modifier, but for setting a simple color, you pass a Color
value.
Customize SwiftUI VStack Background
SwiftUI allows you to create custom colors if the predefined ones do not fit your needs. You can define a color using RGB values as follows:
VStack {
Text("Hello")
Text("SwiftUI")
}
.background(Color(red: 0.2, green: 0.8, blue: 0.6))
In this example, the VStack has a custom color background created using RGB values.
Setting the background color of a VStack in SwiftUI allows you to create more appealing and visually distinct user interfaces. It’s a simple yet powerful way to enhance the aesthetic of your app.
Remember, while colors can make your app more attractive, it’s essential to maintain a balance and ensure that the user experience is not hampered. Always keep readability and user experience in mind when styling your app.
2 Comments