How to Add TextField in iOS SwiftUI
The SwiftUI TextField
is an essential control for gathering text input from users. From customization to actions, it offers various functionalities. In this guide, we’ll take an in-depth look at the different ways you can use SwiftUI TextField
, explaining each example in detail.
Basic TextField Usage
Simple TextField
A fundamental usage of TextField
is gathering plain text input. The placeholder provides a hint to the user:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter your text here", text: $text)
.padding()
}
}
In this example, the text entered by the user will be stored in the text
variable, which is bound to the TextField
.
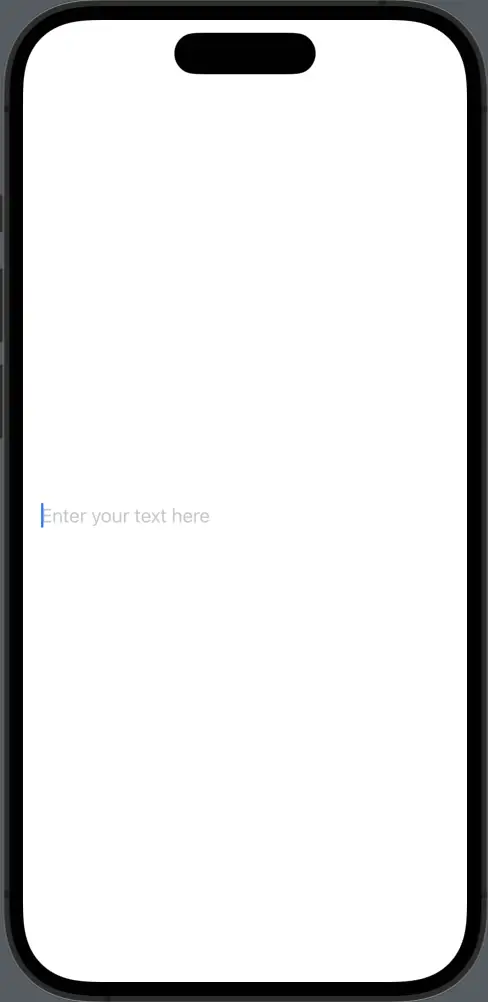
Customize TextField Appearance
TextField with Border
You can add a rounded border to make the text field more visually appealing:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter your text here", text: $text)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
}
}
Here, the RoundedBorderTextFieldStyle
modifier adds the rounded border.
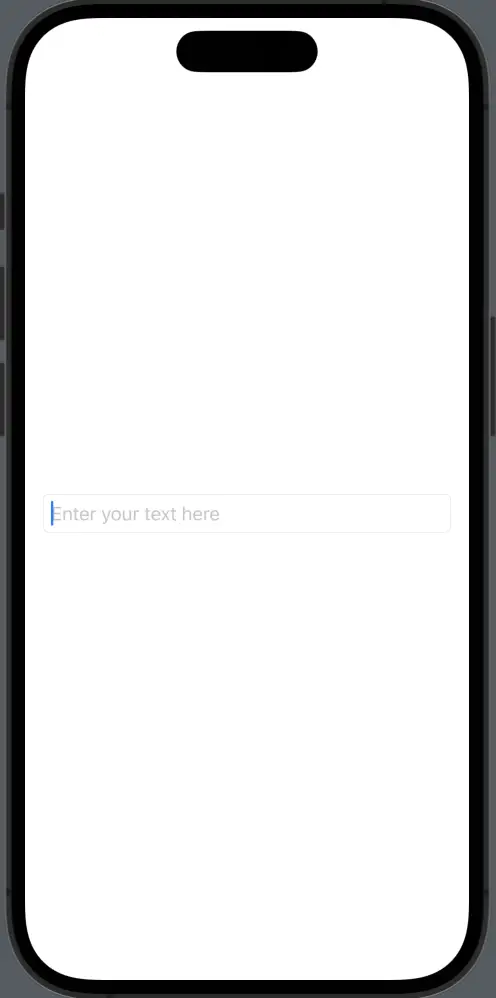
TextField with Custom Font and Color
Customizing the font and text color gives you control over the appearance:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter your text here", text: $text)
.font(.title)
.foregroundColor(.purple)
.padding()
}
}
In this case, the font
and foregroundColor
modifiers are used to change the text appearance.
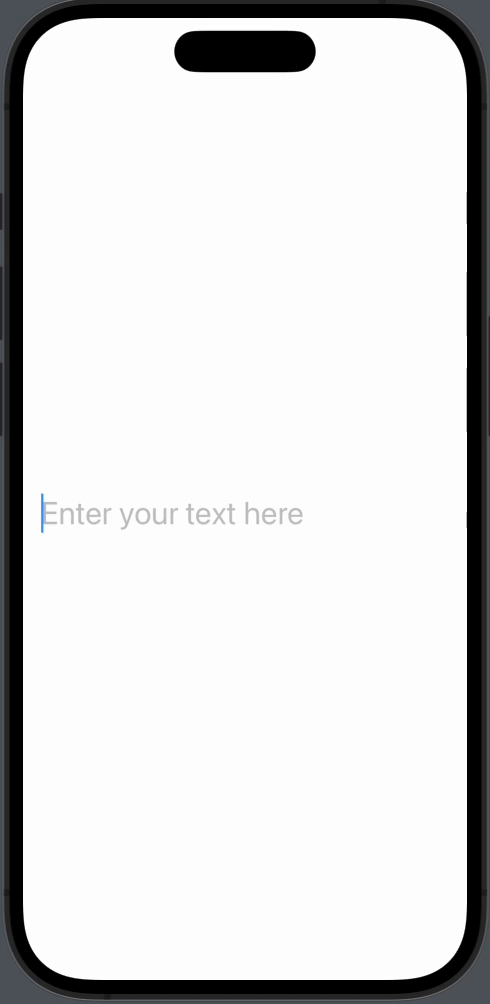
TextField with Actions
TextField with On-Commit Action
Actions can be triggered when the user presses the return key:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter your text here", text: $text, onCommit: {
print("User pressed return key!")
})
.padding()
}
}
The onCommit
closure is called when the return key is pressed, allowing you to perform a specific action.
Secure TextField
Secure Input
For confidential information like passwords, you can use SecureField
:
struct ContentView: View {
@State private var password = ""
var body: some View {
SecureField("Enter your password", text: $password)
.padding()
}
}
The SecureField
ensures the input is masked, keeping sensitive information secure.
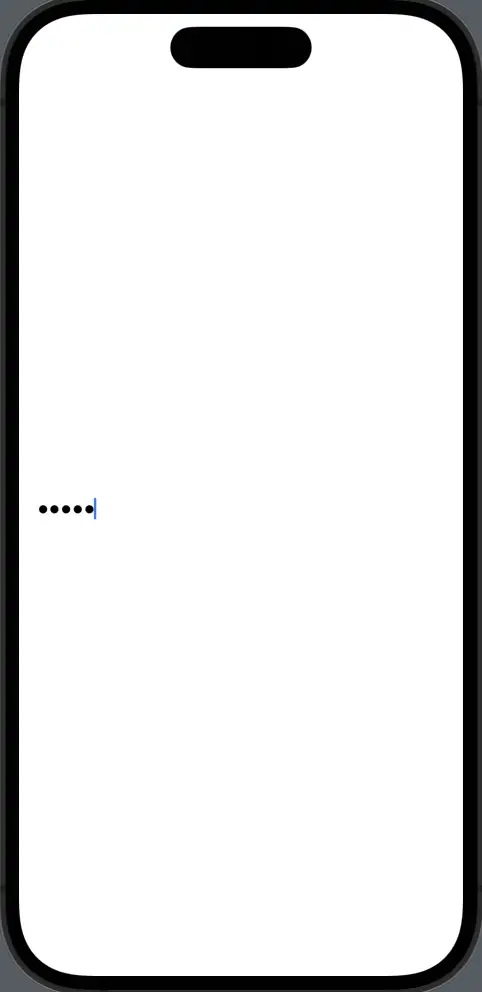
SwiftUI’s TextField
offers various options to gather and handle text input in an elegant and functional manner. From basic usage to specialized styles and actions, this guide provides the understanding needed to effectively use this essential component in your SwiftUI applications.