How to Create a Form in iOS SwiftUI
Forms are integral components of many applications, allowing users to input information through a structured and intuitive interface. SwiftUI provides a simple yet powerful way to create forms on iOS, macOS, and other Apple platforms.
This blog post will walk you through the fundamental aspects of building a form using SwiftUI.
Create a Basic Form: Example
Here’s a basic example of a form that includes various input fields:
struct ContentView: View {
@State private var username = ""
@State private var password = ""
@State private var rememberMe = false
var body: some View {
Form {
Section(header: Text("User Information")) {
TextField("Username", text: $username)
SecureField("Password", text: $password)
}
Toggle("Remember Me", isOn: $rememberMe)
Button("Login") {
// Handle login action
}
}
}
}
Key Components of the Code
1. Form
The Form
view is the container for all the form’s elements, like text fields, buttons, toggles, etc.
2. Section
Sections are used to group related controls together. Here, the Section
is used to group the username and password fields.
3. TextField
and SecureField
TextField
is used for standard text input, while SecureField
is used for sensitive inputs like passwords.
4. Toggle
A Toggle
is used to represent an on/off switch, as in the “Remember Me” option.
5. Button
A Button
is used to trigger actions, like handling the login process.
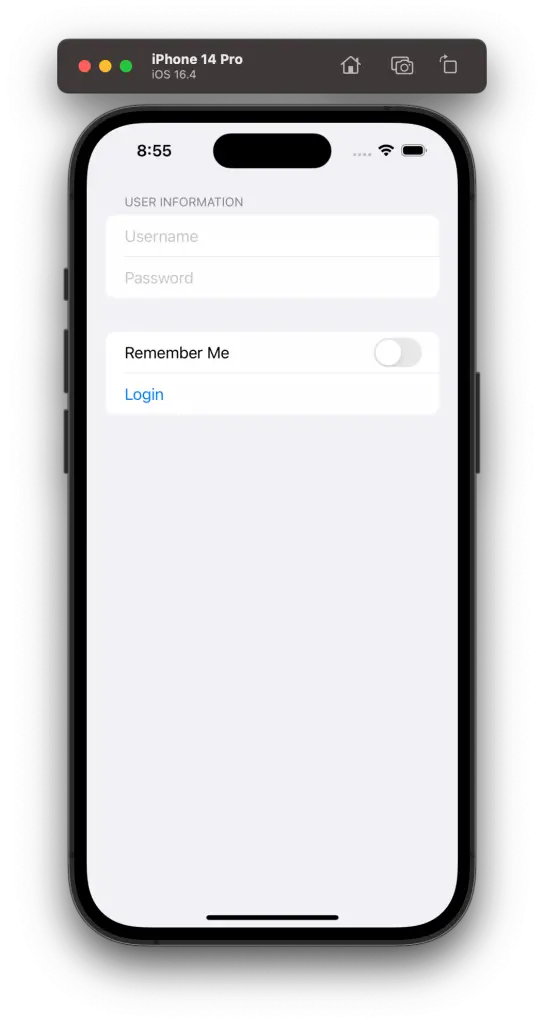
Advanced Customizations
SwiftUI allows for extensive customization of forms. Here are some options:
- Picker Controls: Integrate picker controls for options like date selection or drop-down lists.
- Validation: Add validation to ensure that the data entered meets specific criteria.
- Styling: Use modifiers to apply custom styling to each form element.
Handle Form Data
The form data can be handled through various SwiftUI mechanisms, such as @State
, @Binding
, @EnvironmentObject
, or even custom view models using the Combine framework. You can choose an approach that best fits your application’s architecture and complexity.
SwiftUI’s form component offers a powerful and straightforward way to collect user input in a structured and visually consistent manner. With various view types and modifiers at your disposal, you can create sophisticated forms that provide an intuitive user experience.
The example in this blog post is a foundational guide to building and customizing forms according to your app’s needs and design preferences.