How to Disable Button in iOS SwiftUI
In this blog post, we will explore an integral aspect of user interaction in mobile apps – disabling buttons in SwiftUI. Effectively managing user interactions, such as disabling a button under certain conditions, is crucial for creating intuitive and user-friendly applications.
Basics: Disable a Button
In SwiftUI, a button can be disabled using the .disabled()
modifier, which accepts a Boolean value:
Button(action: {
// your action code here
}) {
Text("Click me")
}
.disabled(true)
If disabled
is true, the button will be disabled; otherwise, it will be enabled.
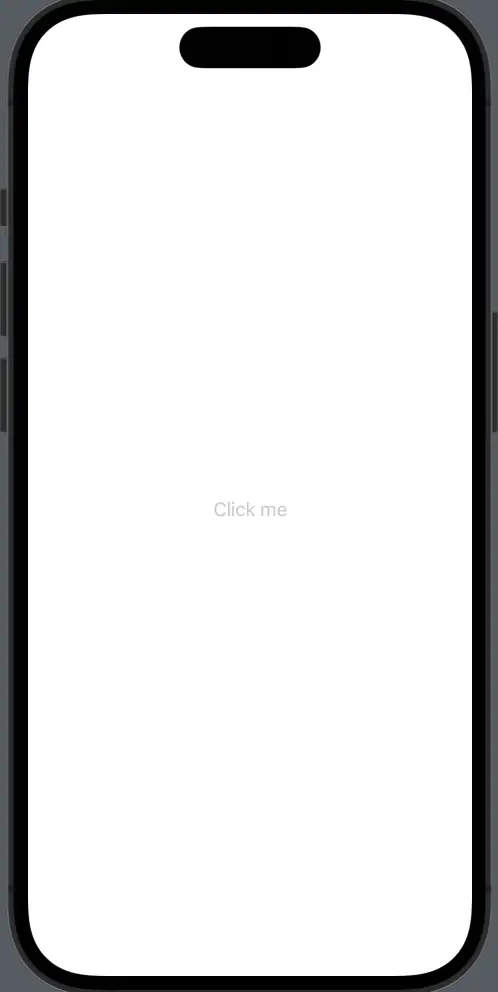
Change Button Appearance When Disabled
When a button is disabled, it’s often desirable to change its appearance to communicate its state to the user. SwiftUI automatically applies a lowered opacity effect to disabled buttons. However, you can further customize the button’s appearance based on its enabled state:
Button(action: {
// your action code here
}) {
Text("Click me")
.padding()
.background(isButtonDisabled ? Color.gray : Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
.disabled(isButtonDisabled)
Here, the button’s background color changes based on the isButtonDisabled
Boolean. If the button is disabled, the background color is gray; otherwise, it is blue.
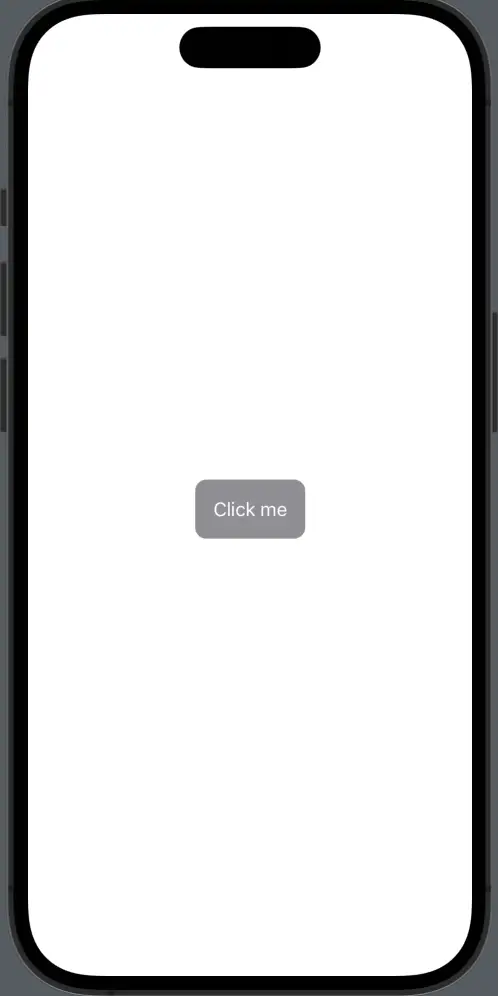
Dynamically Disable a Button
Often, you’ll want to disable a button based on the logic of your app. For instance, a “Submit” button might be disabled until all fields in a form are correctly filled. SwiftUI makes this easy:
@State private var textFieldInput = ""
Button(action: {
// your action code here
}) {
Text("Submit")
}
.disabled(textFieldInput.isEmpty)
In this case, the “Submit” button is disabled as long as the textFieldInput
string is empty, indicating that the user has not yet input any text.
Understanding how to disable buttons in SwiftUI and alter their appearance based on their enabled state is a key skill for creating smooth, intuitive user experiences. By leveraging SwiftUI’s powerful and straightforward modifiers, you can effectively manage and control user interactions in your app.