How to Add Button with Image and Text in iOS SwiftUI
In this blog post, we delve into creating interactive buttons featuring both images and text in SwiftUI. Buttons are pivotal for user interactivity, and the ability to incorporate both text and images within them makes your application more dynamic and visually appealing.
Basics: SwiftUI Button Structure
A SwiftUI button is made up of two main components: a display (what the user sees) and an action (what happens when the user taps the button). Here’s a basic SwiftUI button:
Button(action: {
// What should happen when the button is tapped
print("Button tapped!")
}) {
// What the button looks like
Text("Tap me!")
}
Adding an Image to a Button
To add an image to a button in SwiftUI, you can use the Image
view in place of (or in addition to) the Text
view:
Button(action: {
print("Button tapped!")
}) {
Image(systemName: "star")
}
In the above example, instead of a text label, the button displays a star icon. systemName
indicates we’re using one of Apple’s built-in SF Symbols. You can replace "star"
with the name of any SF Symbol to use that icon.
Create a Button with Image and Text
To display both an image and text within a button, you can combine Image
and Text
views inside an HStack
:
Button(action: {
print("Button tapped!")
}) {
HStack {
Image(systemName: "star")
Text("Tap me!")
}
}
In this code snippet, the button will display a star icon next to the text “Tap me!”.
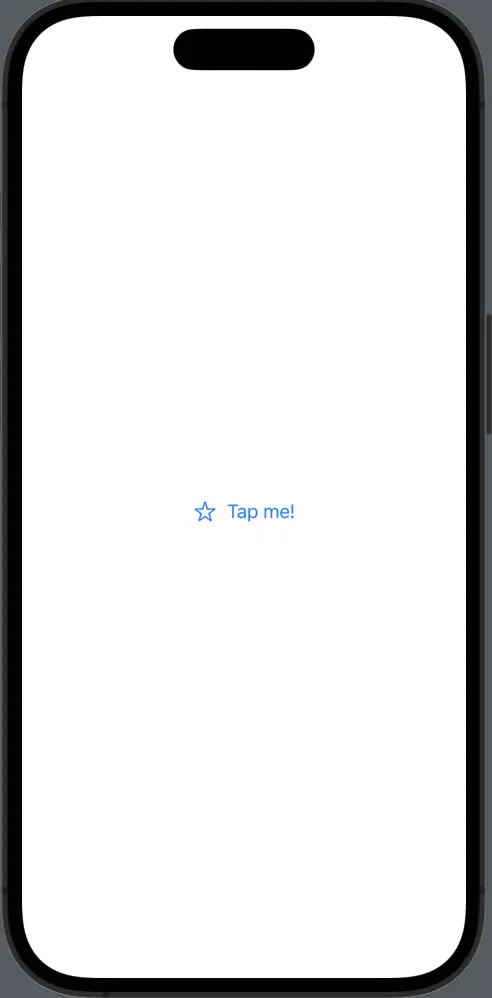
Customize the Button’s Appearance
SwiftUI allows you to modify the button’s appearance using various view modifiers:
Button(action: {
print("Button tapped!")
}) {
HStack {
Image(systemName: "star")
.foregroundColor(.yellow)
Text("Tap me!")
.fontWeight(.bold)
.font(.title)
}
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
In this more advanced example, we’ve added foreground color to the image and text, made the text bold and larger, added padding around the button content, set a background color for the button, changed the button text color to white, and made the button corners rounded.
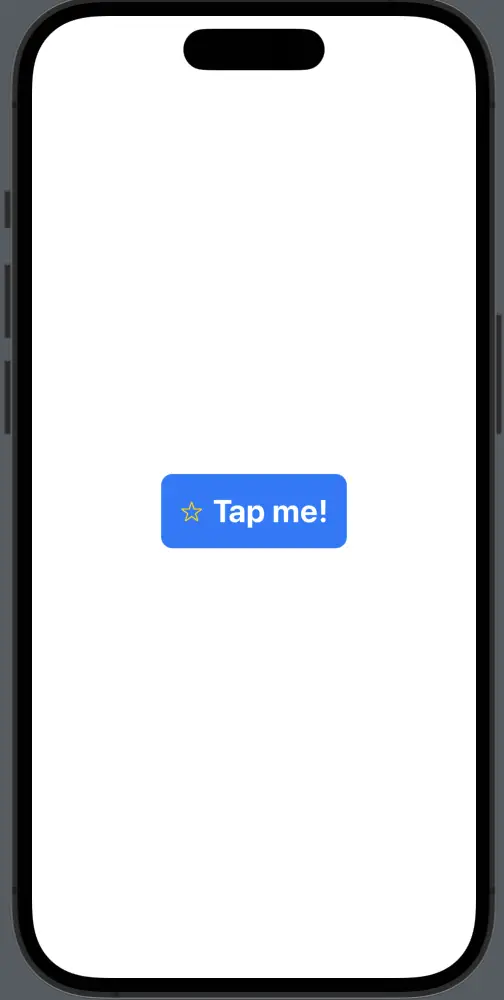
Conclusion
Incorporating both text and images into your SwiftUI buttons is a great way to create visually appealing and intuitive interfaces. With SwiftUI’s composability and easy-to-use view modifiers, you can create beautiful, interactive buttons with just a few lines of code. We hope this blog post inspires you to experiment with and add your creative touch to your SwiftUI buttons.