How to Place Button at the Bottom of the Screen in iOS SwiftUI
In this blog post, we will explore one of the more common design paradigms seen in mobile applications – positioning a button at the bottom of the screen in SwiftUI. This layout is often used to host primary actions such as “Save”, “Next”, “Confirm” or “Submit”, allowing easy access for users and thus enhancing user experience.
Let’s delve into how SwiftUI can help us achieve this layout with its intuitive, declarative syntax.
Basics: Place a Button
First, let’s understand how to create a basic button in SwiftUI. Here’s a simple example:
Button(action: {
// Handle button tap here
}) {
Text("Tap me!")
}
The Button
view in SwiftUI takes two primary arguments: an action closure that defines what happens when the button is tapped, and a label view builder closure that defines how the button looks.
VStack and Spacer
To place this button at the bottom of the screen, you need to use a VStack
in combination with a Spacer
:
VStack {
Spacer()
Button(action: {
// Handle button tap here
}) {
Text("Tap me!")
}
.padding()
}
The Spacer
view expands to occupy as much space as possible, pushing the button to the bottom. The .padding()
modifier is added to give some space around the button.
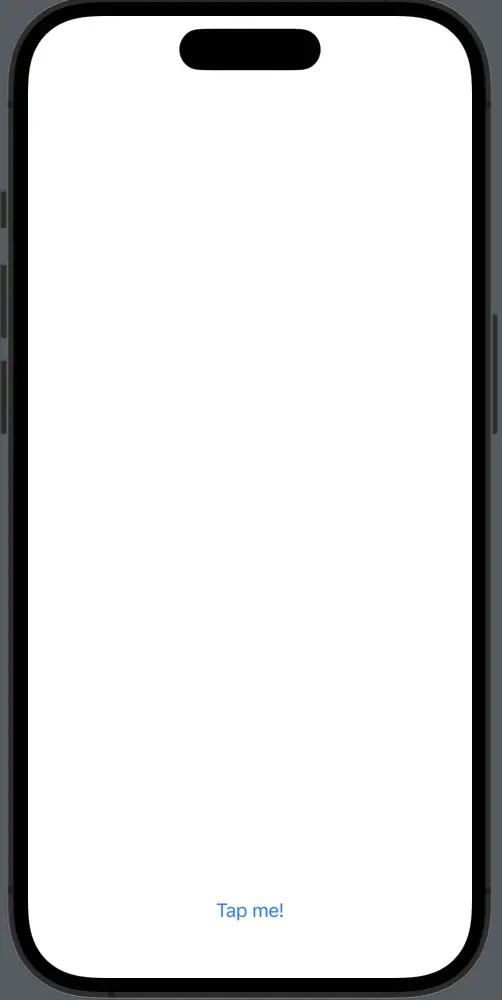
Safe Area Considerations
When placing a button at the bottom of the screen, you must consider the device’s safe area, particularly on devices with a home indicator at the bottom of the screen. SwiftUI makes this easy with the .edgesIgnoringSafeArea()
modifier:
VStack {
Spacer()
Button(action: {
// Handle button tap here
}) {
Text("Tap me!")
}
.padding()
}
.edgesIgnoringSafeArea(.bottom)
This will ensure that the button extends to the very bottom edge of the screen, including the area under the home indicator, if present.
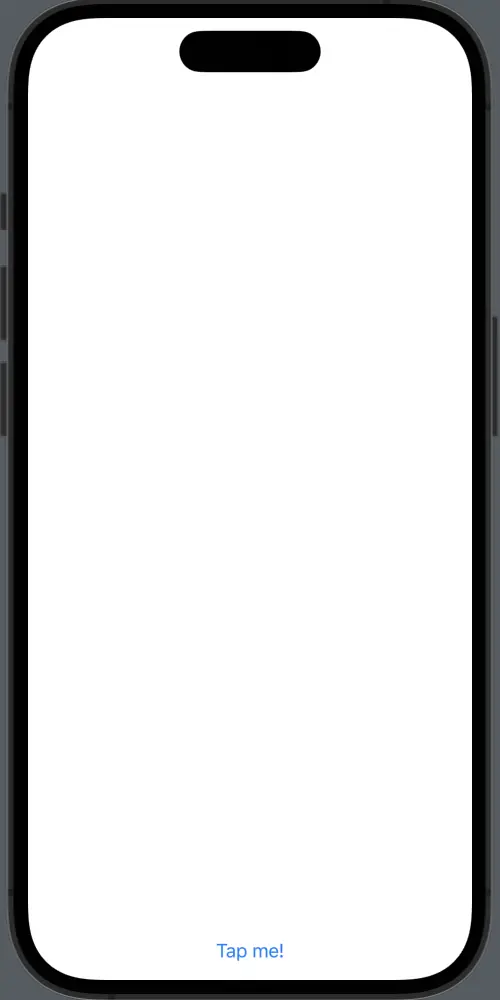
Example with Text and Button
Here’s an example of a SwiftUI view with a text element centered on the screen and a button placed at the bottom.
VStack {
Spacer()
Text("Hello, SwiftUI!")
.font(.title)
.multilineTextAlignment(.center)
Spacer()
Button(action: {
// Handle button tap here
}) {
Text("Tap me!")
.padding(10)
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
.padding(.bottom)
}
.edgesIgnoringSafeArea(.bottom)
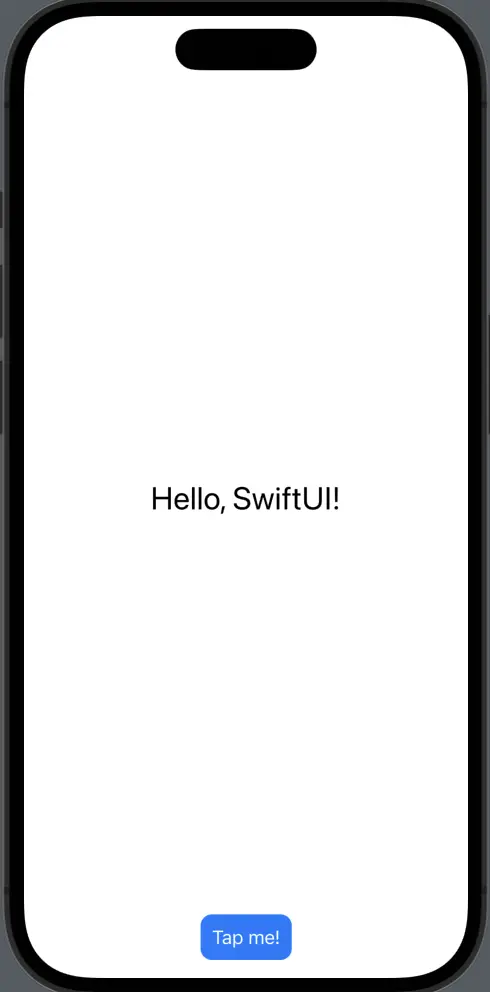
Placing a button at the bottom of the screen is a common UI design seen in many apps. SwiftUI, with its powerful and flexible layout system, allows us to implement this design with ease. By using a VStack
and Spacer
, you can position a button at the bottom of any screen, ensuring that it remains accessible and easy to use for all your app’s users.