How to Show Image from URL in iOS SwiftUI
The importance of image as a UI element is significant. In this beginner iOS app development tutorial, let’s learn how to load an image from a URL in iOS.
You should choose Image to load an image from assets. Instead, here you have to choose AsyncImage to show an image from the URL. AsyncImage is basically a View that loads synchronously to display an image.
Let’s jump into the tutorial by creating a new iOS app project using Xcode. Open ContentView.swift and clear the existing code. Then add the following code.
import SwiftUI
struct ContentView: View {
var body: some View {
AsyncImage(url: URL(string: "https://cdn.pixabay.com/photo/2013/07/13/13/14/tiger-160601_1280.png")) { image in
image.resizable()
} placeholder: {
ProgressView()
}
.frame(width: 300, height: 300)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
I am showing an image from Pixabay using AsyncImage. The image is made resizable and it is restricted to the frame width and height. The ProgressView is set as the placeholder which is shown before loading the image.
You will get the following output.
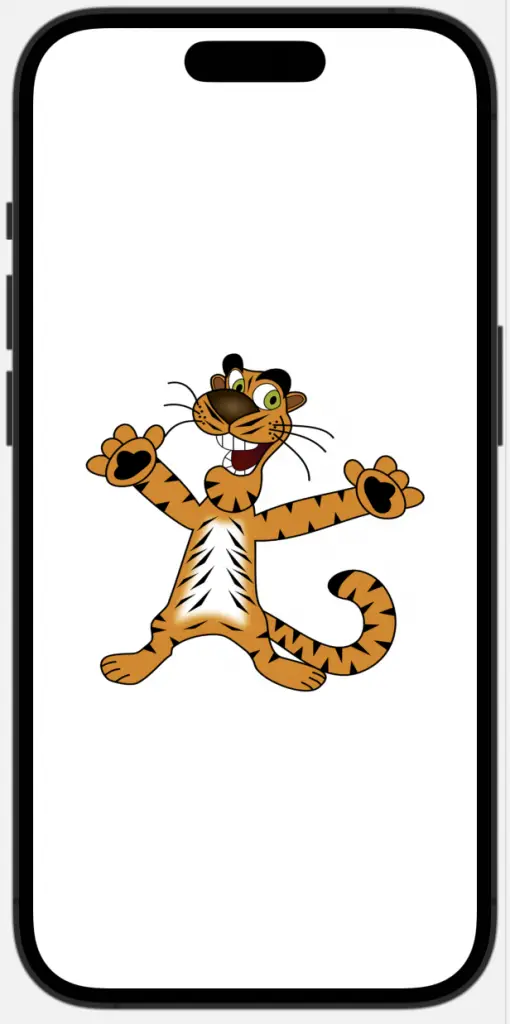
This is how you display an image from a URL in iOS using SwiftUI.