How to Align ZStack Views at the Bottom in iOS SwiftUI
SwiftUI is an innovative and user-friendly framework for building user interfaces across all Apple platforms. One of its robust tools is the ZStack, which allows views to be layered on top of each other. In this post, we’ll delve into aligning views at the bottom of a ZStack.
ZStack Overview
ZStack is a simple yet potent tool in SwiftUI that organizes views along the Z-axis, stacking them on top of each other. But the real magic comes into play when we start aligning these views.
Align Views at the Bottom of a ZStack
Let’s explore how to align views at the bottom of a ZStack.
Step 1: Create a ZStack
First off, you’ll need to define a ZStack.
ZStack {
Text("This is a ZStack")
}
Step 2: Align Text at the Bottom
To align the text at the bottom of the ZStack, you need to use the .frame
and .alignment
modifiers.
ZStack {
Text("This is a ZStack")
.frame(maxWidth: .infinity, maxHeight: .infinity, alignment: .bottom)
}
The maxWidth: .infinity
and maxHeight: .infinity
make the ZStack span the whole screen. Meanwhile, alignment: .bottom
pushes the Text to the bottom of the ZStack.
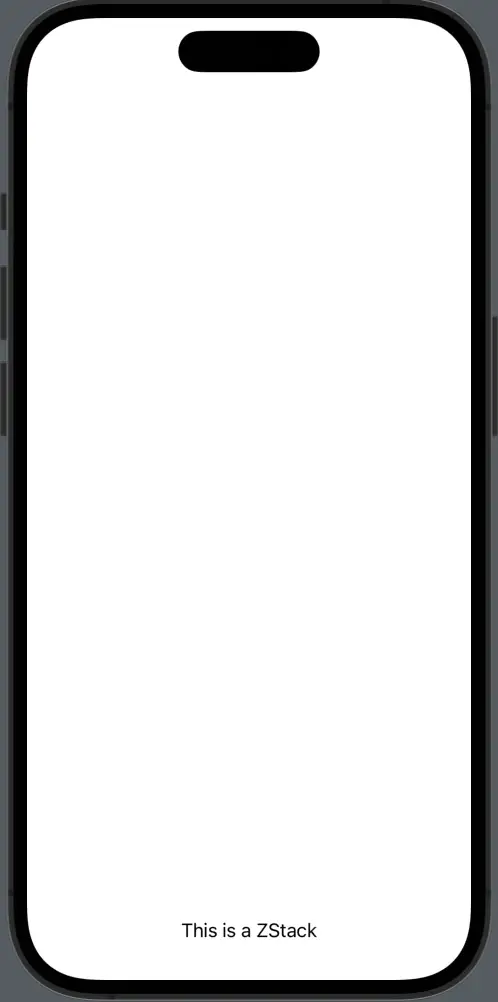
Following is the complete code for reference.
import SwiftUI
struct ContentView: View {
var body: some View {
ZStack {
Text("This is a ZStack")
.frame(maxWidth: .infinity, maxHeight: .infinity, alignment: .bottom)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
SwiftUI’s ZStack is a versatile tool that allows developers to layer views in a stack, offering flexible customization, such as aligning views to the bottom. By simply using the .frame
and .alignment
modifiers, you can efficiently organize your SwiftUI views in no time.