How to Add Picker to List in iOS SwiftUI
SwiftUI has revolutionized the way we build user interfaces for iOS and macOS. One of the most useful elements in SwiftUI is the Picker, and it becomes even more powerful when used within a List.
In this blog post, we’ll explore how to effectively use a SwiftUI Picker within a List to create dynamic and interactive apps.
What is a SwiftUI Picker?
A Picker in SwiftUI is a UI element that allows users to select an option from a list of choices. It can be displayed in various styles, such as a wheel, a segmented control, or even a dropdown menu.
What is a SwiftUI List?
A List in SwiftUI is similar to a UITableView in UIKit. It’s used to display a collection of data in a single-column layout. You can populate it with static or dynamic data.
Why Combine Picker with List?
Combining a Picker with a List allows you to create more complex and interactive UIs. For example, you can let users choose from a list of options for each item in the List.
How to Use Picker in List
Create State Variables
First, create state variables to hold the selected values.
@State private var selectedFruits = ["Apple", "Banana", "Cherry"]
Create the List
Next, add a List to your SwiftUI view.
List {
// List content goes here
}
Add Picker to List
Inside the List, add a Picker.
List {
Picker("Select a fruit", selection: $selectedFruits) {
Text("Apple").tag("Apple")
Text("Banana").tag("Banana")
Text("Cherry").tag("Cherry")
}
}
Complete Example
Here’s how everything comes together:
import SwiftUI
struct ContentView: View {
@State private var selectedFruits = ["Apple", "Banana", "Cherry"]
var body: some View {
List {
Picker("Select a fruit", selection: $selectedFruits) {
Text("Apple").tag("Apple")
Text("Banana").tag("Banana")
Text("Cherry").tag("Cherry")
}
}
}
}
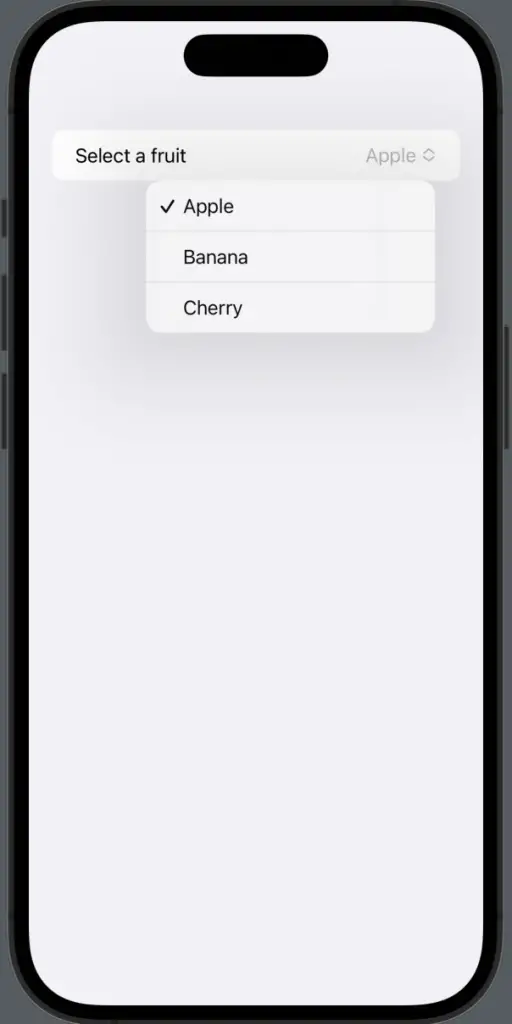
Advanced Tips
- Dynamic Data: You can use dynamic data to populate the Picker options.
- Custom Rows: Customize the List rows to include additional elements like images or text.
- User Interaction: Use other SwiftUI elements like Button or Toggle to interact with the Picker.
Using a Picker within a List in SwiftUI opens up a world of possibilities for creating interactive and dynamic user interfaces. By following the steps outlined in this guide, you can easily integrate these powerful UI elements into your apps.