How to Add Text Border in React Native
Borders are an essential part of UI/UX design, particularly when it comes to text elements. They can add structure, emphasis, and aesthetic appeal to your React Native app. This blog post aims to be your go-to guide for understanding how to add and customize borders around text elements in React Native.
What is Text Border?
In design, a border is essentially an outline around a graphical element. When we talk about text borders in React Native, we’re referring to adding an outline around the Text
component. This helps in setting the text apart from other elements.
Add Basic Text Border
Single-line Borders
For a simple, single-line text element, adding a border is straightforward. The basic properties you’ll need are borderWidth
and borderColor
.
import React from 'react';
import {View, Text, StyleSheet} from 'react-native';
const App = () => (
<View>
<Text style={styles.borderText}>This is a bordered text.</Text>
</View>
);
const styles = StyleSheet.create({
borderText: {
borderWidth: 1,
borderColor: 'red',
},
});
export default App;
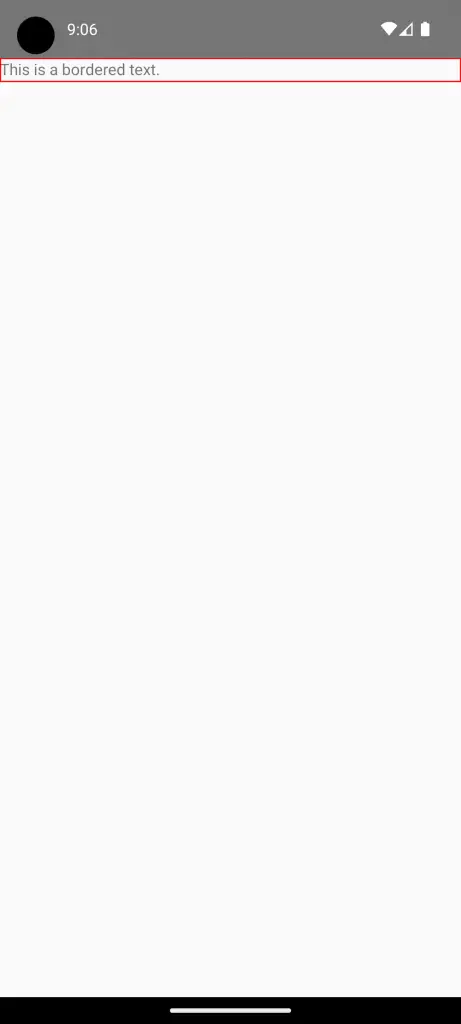
Multi-line Text Borders
For multi-line text, you can use the same border properties. Just ensure to also use the numberOfLines
property on the Text
component.
import React from 'react';
import {View, Text, StyleSheet} from 'react-native';
const App = () => (
<View>
<Text numberOfLines={3} style={styles.borderText}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</Text>
</View>
);
const styles = StyleSheet.create({
borderText: {
borderWidth: 1,
borderColor: 'red',
},
});
export default App;
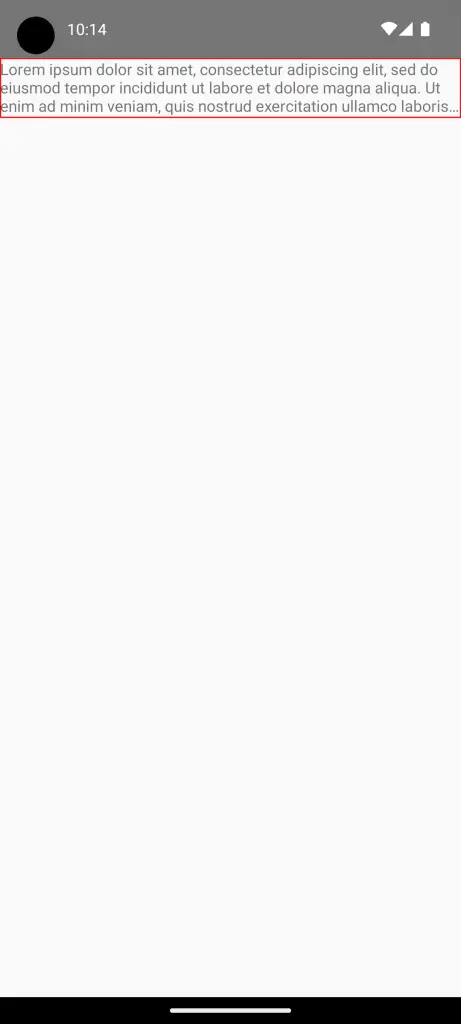
Customize Text Borders
Rounded Corners
To add rounded corners, you can use the borderRadius
property.
<Text style={{ borderWidth: 1, borderColor: 'red', borderRadius: 5 }}>
Rounded border text
</Text>
Different Borders for Each Side
You can set different borders for each side of the text using borderLeftWidth
, borderRightWidth
, borderTopWidth
, and borderBottomWidth
.
<Text
style={{
borderTopWidth: 1,
borderBottomWidth: 2,
borderLeftWidth: 3,
borderRightWidth: 4,
borderColor: 'black',
}}
>
Different borders on each side
</Text>
Adding Padding
Borders hug the text tightly by default. To add some space between the text and its border, use the padding
property.
<Text style={{ borderWidth: 1, borderColor: 'black', padding: 10 }}>
Padded border text
</Text>
Borders are an incredibly versatile tool in your React Native design toolkit. They can help improve readability and draw attention to specific text elements. With a mix of different styles and properties, you can achieve the perfect look for your app.