How to Add Snackbar Messages in React Native
If you are a regular Android user then chances are high that you come across snackbars. The Snackbars are part of the material design and are used to inform users about an action performed or about to perform.
Even though snackbar has some similarities to Android toast, it has its own identity. Usually, Snackbar appears briefly at the bottom of a screen and does not interrupt the user interface.
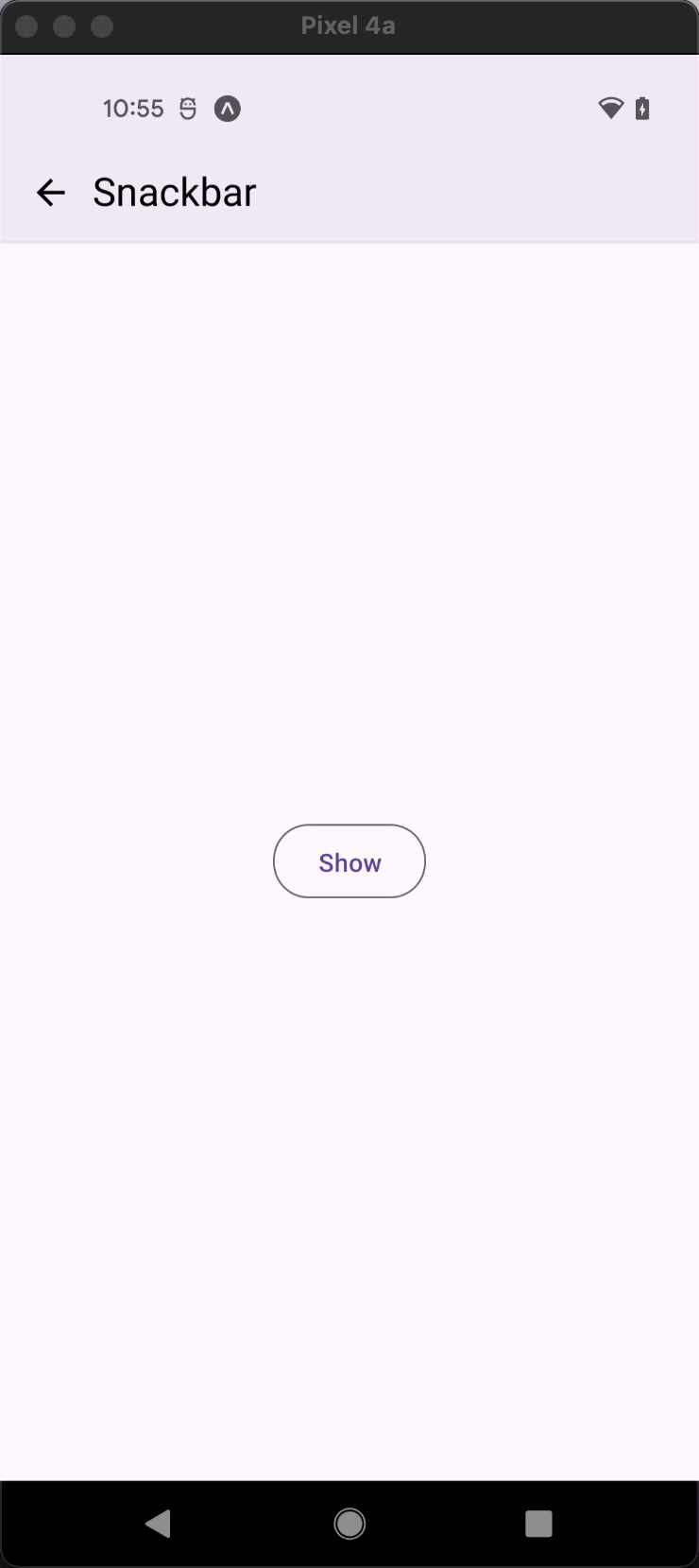
So how to add snackbar in react native which works both on android and ios platforms?
There are some third-party libraries out there with snackbar component. In this react native snackbar example, I am going with react native snackbar library.
First of all, add the library using any of the following commands.
npm install react-native-snackbar --save
or
yarn add react-native-snackbar
If you have any installation problems then you can follow the manual steps given here.
In the following react native example, I show snackbar when the touchableOpacity is pressed. The duration is given indefinitely and the snackbar gets dismissed once the undo is pressed.
import React from 'react';
import {View, Text, TouchableOpacity} from 'react-native';
import Snackbar from 'react-native-snackbar';
const App = () => {
const showSnackbar = () => {
Snackbar.show({
text: 'Hello world',
//You can also give duration- Snackbar.LENGTH_SHORT, Snackbar.LENGTH_LONG
duration: Snackbar.LENGTH_INDEFINITE,
backgroundColor: 'black',
textColor: 'white',
action: {
text: 'UNDO',
textColor: 'green',
onPress: () => {
console.log('clicked');
},
},
});
};
return (
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<TouchableOpacity onPress={() => showSnackbar()}>
<Text>Touch Here To Make Snackbar Appear</Text>
</TouchableOpacity>
</View>
);
};
export default App;
The output will be as given below:

If you are looking for alternative libraries with snackbar component then have a look at react native paper library.
That’s how you add snackbar in react native.