How to Change Focus Border Color in React Native TextInput
In React Native, changing the visual cues for focused input fields can enhance user interaction. The border color of a TextInput
component is one such visual cue. This blog post delves into methods for changing the focus border color of TextInput
in React Native.
Prerequisites
- Familiarity with React Native and JavaScript
- A functional React Native setup
Basic TextInput Component
Firstly, let’s review how to add a simple TextInput
component in your React Native application.
import React from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
return (
<View>
<TextInput placeholder="Enter text here" />
</View>
);
};
Static Border Color Change
You can set the initial border color using the style
prop.
<TextInput
placeholder="Enter text here"
style={{ borderColor: 'blue', borderWidth: 1 }}
/>
The borderColor
sets the color of the border. The borderWidth
sets the width of the border.
Dynamic Focus Border Color
To change the border color when the TextInput
is focused, you can use component state and event handlers.
import React, { useState } from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
const [isFocused, setFocused] = useState(false);
return (
<View>
<TextInput
placeholder="Enter text here"
style={{
borderColor: isFocused ? 'green' : 'blue',
borderWidth: 1,
}}
onFocus={() => setFocused(true)}
onBlur={() => setFocused(false)}
/>
</View>
);
};
export default App;
Explanation
useState
is used to manage whether theTextInput
is focused.onFocus
andonBlur
event handlers change the state when the input is focused or blurred.- The
borderColor
is conditionally set based on theisFocused
state.
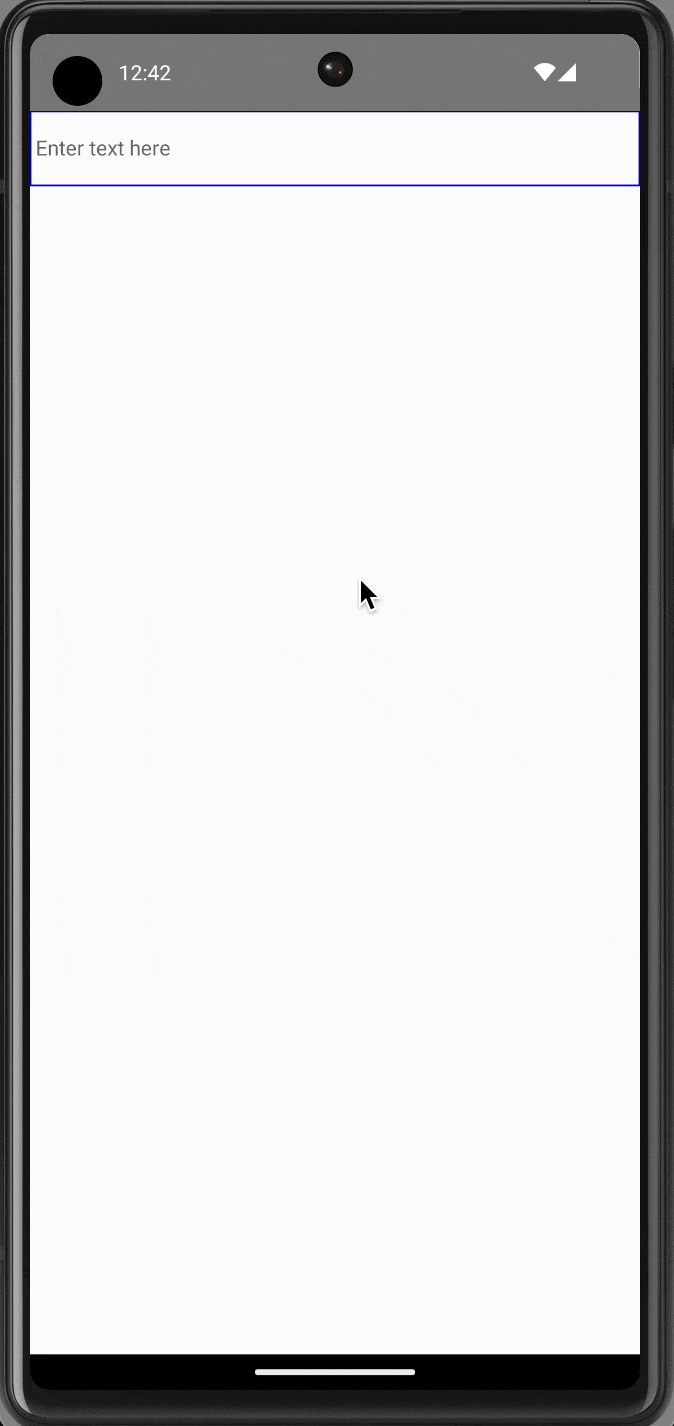
Changing the border color of a TextInput
when focused is a great way to improve user feedback in React Native apps. By using component state and event handlers like onFocus
and onBlur
, you can provide a more interactive and responsive experience.