How to Change Text Background Color in React Native
Adding background colors to text components in React Native can enhance the user interface of your app. While it’s a simple task, knowing the different methods to do so is beneficial.
In this guide, we’ll walk through how to set background colors for text components in React Native and explore some use-cases.
Basic Method: Using Inline Style
Set Background Color
You can set a background color for a text component in React Native with inline styling, using the backgroundColor
property.
import React from 'react';
import {View, Text} from 'react-native';
const App = () => (
<View>
<Text style={{backgroundColor: 'yellow'}}>
This text has a yellow background.
</Text>
</View>
);
export default App;
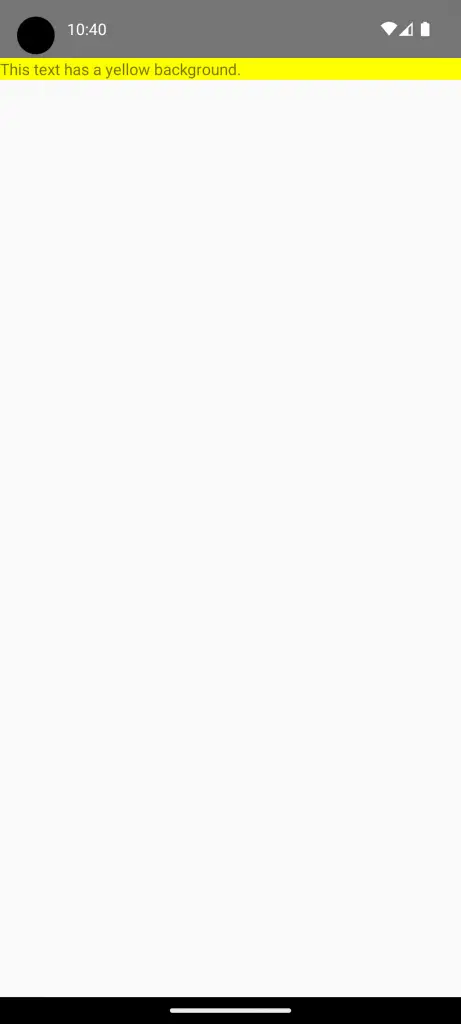
Advanced Techniques
Use RGBA Colors
The backgroundColor
property supports RGBA, allowing you to add transparency to your background color.
<Text style={{ backgroundColor: 'rgba(0, 0, 255, 0.3)' }}>
This text has a transparent blue background.
</Text>
Use Hexadecimal Codes
If you prefer using hexadecimal color codes, the backgroundColor
property supports that too.
<Text style={{ backgroundColor: '#0000FF' }}>
This text has a blue background.
</Text>
Use Global Styles
For repeated use of the same background color, it’s better to define a global stylesheet.
import { StyleSheet } from 'react-native';
const styles = StyleSheet.create({
blueBackground: {
backgroundColor: 'blue',
},
});
<Text style={styles.blueBackground}>
This text has a blue background.
</Text>
Setting background colors for text components in React Native is pretty straightforward. With the flexibility of inline styles and global stylesheets, you can easily add this visual element to enhance your app’s UI.
Experiment with different color combinations and transparency levels to make your app visually appealing.