How to Create Horizontal Progress Bar in React Native (Android Only)
As you know, the ActivityIndicator component of React Native is round in shape and not horizontal. If you need a horizontal progress bar in your React Native Android app then you should use ProgressBarAndroid component.
The ProgressBarAndroid component should be installed from react native community using any of the following commands.
npm install @react-native-community/progress-bar-android --save
or
yarn add @react-native-community/progress-bar-android
For iOS, run the following command too.
npx pod-install
The following snippet creates a horizontal progress bar in red color.
<ProgressBar
styleAttr="Horizontal"
color="red"
indeterminate={false}
progress={0.5}
/>
Following is the complete example.
import React from 'react';
import {View} from 'react-native';
import {ProgressBar} from '@react-native-community/progress-bar-android';
const App = () => {
return (
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<ProgressBar
style={{width: 150}}
styleAttr="Horizontal"
color="#2196F3"
/>
</View>
);
};
export default App;
Following is the output of react native horizontal progress bar example.
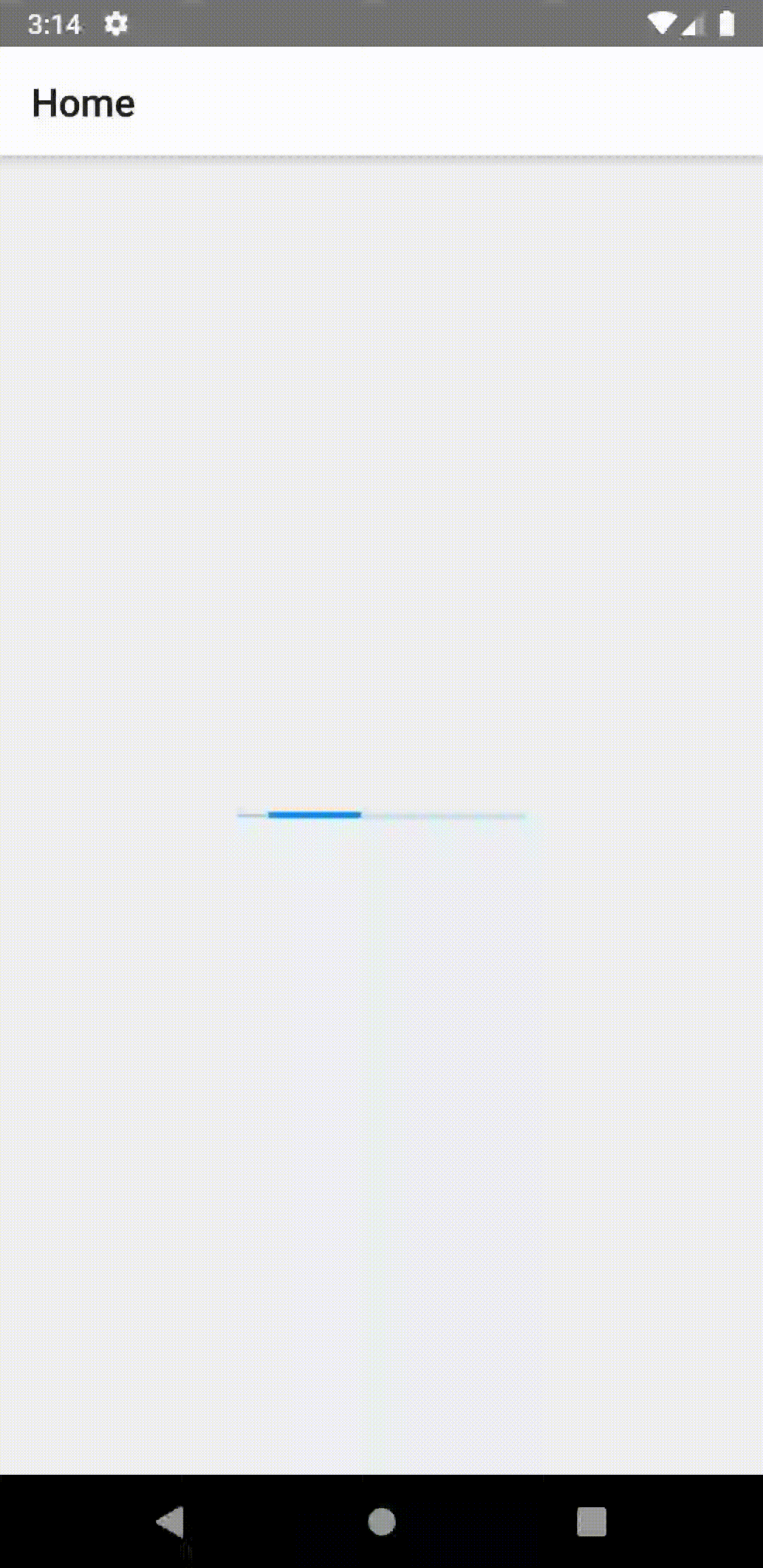
That’s how you show the horizontal progress bar in react native.