How to Add Stepper Without Label in iOS SwiftUI
Steppers are useful UI components that allow users to increment or decrement a value. In SwiftUI, adding a stepper is a breeze. But what if you want to hide the label and only show the stepper buttons? In this blog post, we’ll learn how to add a stepper without a label in iOS using SwiftUI.
The Basic Stepper
Before diving into hiding labels, let’s look at how to create a basic stepper. The code is straightforward:
import SwiftUI
struct ContentView: View {
@State private var counter = 0
var body: some View {
Stepper("Counter: \(counter)", value: $counter)
}
}
This will display a stepper with a label showing the current value of counter
.
Hide the Label
To hide the label, you can use the labelsHidden()
modifier. Here’s the example you provided:
import SwiftUI
struct ContentView: View {
@State private var counter = 0
var body: some View {
Stepper("Counter: \(counter)", value: $counter).labelsHidden()
}
}
By adding .labelsHidden()
, the label will be hidden, and only the stepper buttons will be visible.
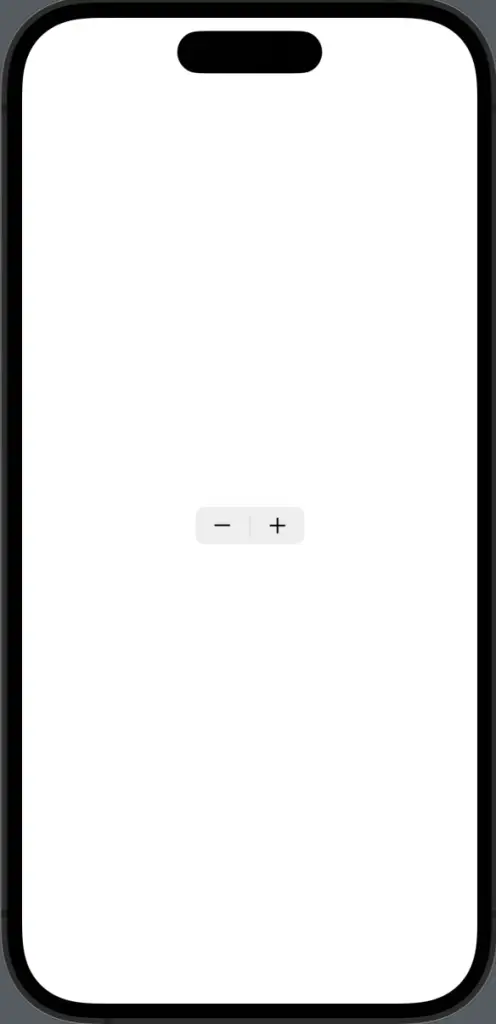
Why Hide Labels?
Hiding labels can be useful for several reasons:
- To save screen space.
- To focus user attention on the stepper buttons.
- To create a cleaner UI.
Hiding the label of a stepper in SwiftUI is simple. Just add the .labelsHidden()
modifier to your stepper, and you’re good to go. This can be useful for creating a cleaner UI or saving screen space.