How to Do VStack Bottom Alignment iOS SwiftUI
In SwiftUI, VStack provides an easy way to manage views vertically. But what if you want to align these views towards the bottom? In this blog post, we’ll dive into how you can achieve bottom alignment using VStack.
An Overview of VStack
VStack is a struct in SwiftUI that arranges views vertically. This layout comes in handy when you want to stack views on top of one another. However, by default, VStack aligns its contents in the center. To align it to the bottom, we need to understand Spacer.
Bottom Alignment in VStack using Spacer
The Spacer is another struct in SwiftUI that takes up as much space as it can. When you place it in a VStack, it pushes everything else towards the top, leaving the rest of the space at the bottom.
Step 1: Set Up VStack
Start by setting up a VStack with your views. Here’s a simple example:
VStack {
Text("Hello")
Text("SwiftUI")
}
Step 2: Introduce Spacer
Next, introduce a Spacer in your VStack. The Spacer will push all the other views upwards, aligning them at the top and leaving the space below empty.
VStack {
Spacer()
Text("Hello")
Text("SwiftUI")
}
In this VStack, “Hello” and “SwiftUI” are now aligned at the bottom.
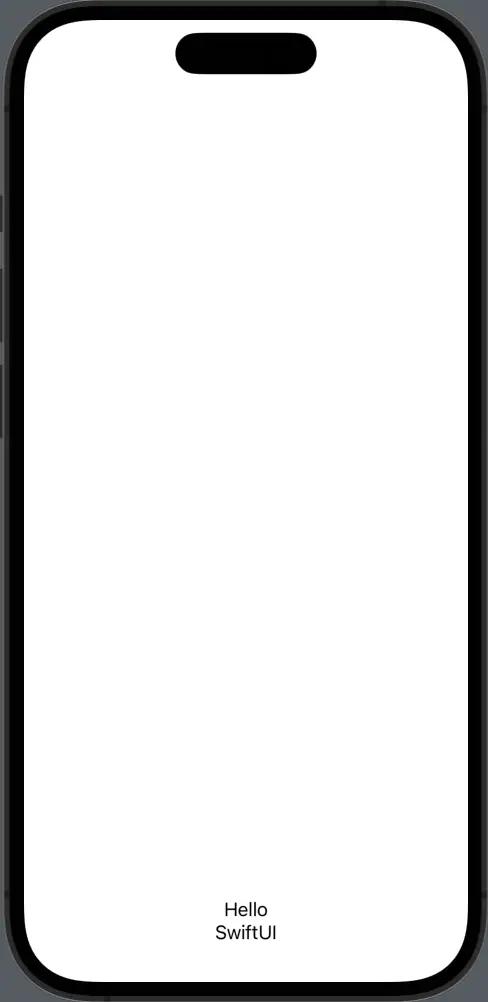
Bottom Alignment in VStack using Frame
In addition to Spacer, you can also use the .frame
modifier to align views to the bottom within a VStack. The frame modifier offers you control over the alignment and size of your views.
VStack(alignment: .center) {
Text("Hello")
Text("SwiftUI")
}.frame(maxHeight: .infinity, alignment: .bottom)
Now, you’ll find that “Hello” and “SwiftUI” are aligned at the bottom. The VStack occupies the full height of its parent view, and the Text views are at the bottom of that frame.
There you have it! Two different ways to align your views to the bottom using VStack in SwiftUI. Whether you choose Spacer or Frame depends on the specific needs of your layout. Each method has its benefits and use cases, so feel free to use what suits you best.