How to Make VStack Scrollable in SwiftUI
If you’ve ever been in a situation where your SwiftUI VStack’s content overflows beyond the viewable screen area, you know it’s time to make your VStack scrollable. This blog post explores how to do just that.
When to Make VStack Scrollable
In SwiftUI, VStack is a great tool for organizing views vertically. However, there might be times when your VStack’s contents exceed the device’s screen height. When this happens, parts of your content become inaccessible. Here’s where making your VStack scrollable comes in handy.
Create a Scrollable VStack
Creating a scrollable VStack in SwiftUI is quite simple and straightforward. The trick lies in the ScrollView component.
Step 1: Add a ScrollView
The first step in making your VStack scrollable is by wrapping it in a ScrollView. SwiftUI’s ScrollView component allows users to scroll through content that surpasses the bounds of the screen.
ScrollView {
VStack {
// Your VStack content here
}
}
Step 2: Add VStack Content
Add your VStack contents. For instance, we can add a series of text views:
ScrollView {
VStack {
ForEach(0..<100) { number in
Text("Row \(number)")
}
}
}
This SwiftUI code creates a VStack with 100 rows of text. Thanks to the ScrollView, you can now easily scroll through all the VStack content, no matter how long.
Step 3: Expand VStack
Now, here’s the crucial part. We need to add a Spacer at the end of our VStack and set the frame of the VStack to cover the full width of the ScrollView:
ScrollView {
VStack {
ForEach(0..<100) { number in
Text("Row \(number)")
}
Spacer(minLength: 0)
}
.frame(maxWidth: .infinity)
}
This will make the VStack span the entire width of the ScrollView, making the entire area of VStack scrollable, not just the text.
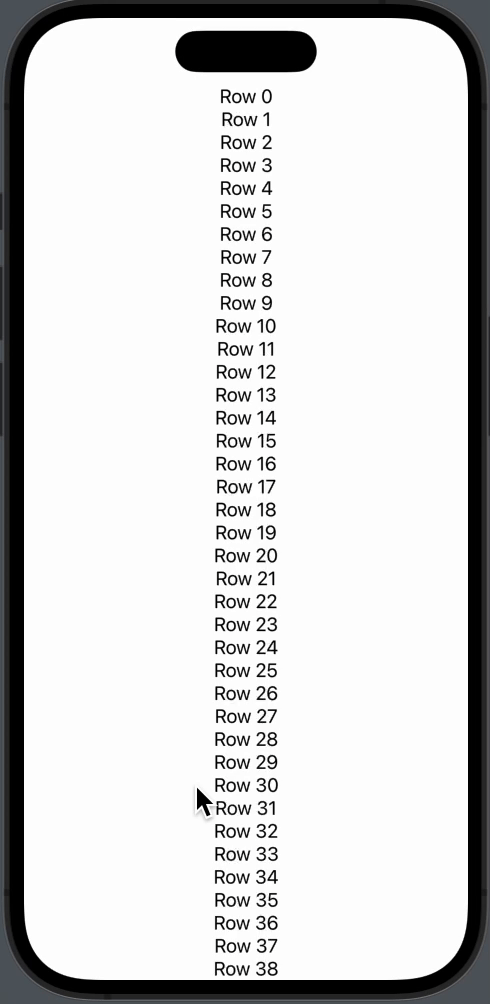
There you have it! With SwiftUI’s ScrollView component, you can easily make any VStack scrollable, solving the problem of content overflow. Embrace scrollable VStacks and ensure your app’s content is always accessible!