How to Do Icon Animation in React Native
Creating visually appealing animations in React Native can greatly enhance the user experience in your mobile application. One great way to do this is by animating icons. In this blog post let’s do icon animation in react native.
We are using react native vector icons library for this tutorial. I recommend you to read how to use react native vector icons to install and set up the package.
We can create animation by combining the Icon component and the Animated API. You should create an animated component as given below.
import Icon from 'react-native-vector-icons/MaterialCommunityIcons';
const AnimatedIcon = Animated.createAnimatedComponent(Icon);
Now you can create any kind of animation using the Animated API.
Following is an example where I animate an icon that slightly rotates and gets back to its original position when the icon is pressed.
import {View, Animated} from 'react-native';
import React, {useState} from 'react';
import Icon from 'react-native-vector-icons/MaterialCommunityIcons';
const AnimatedIcon = Animated.createAnimatedComponent(Icon);
function App() {
const [spinAnim, setSpinAnim] = useState(new Animated.Value(0));
const [iconPressed, setIconPressed] = useState(false);
const spin = spinAnim.interpolate({
inputRange: [0, 0.5, 1],
outputRange: ['0deg', '15deg', '0deg'],
});
function rotate() {
Animated.spring(spinAnim, {
toValue: iconPressed ? 0 : 1,
bounciness: 0,
useNativeDriver: true,
}).start();
}
return (
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<AnimatedIcon
name="home"
size={50}
color="red"
style={{transform: [{rotate: spin}]}}
onPress={() => {
setIconPressed(!iconPressed);
rotate();
}}
/>
</View>
);
}
export default App;
The Animated.createAnimatedComponent function is used to create an AnimatedIcon component that can be animated.
We have two state variables: spinAnim and iconPressed. The spinAnim state is initialized with a new Animated.Value object, which will store the value used to control the animation.
The iconPressed state is initialized to false and will be used to keep track of whether the icon has been pressed or not.
The spin constant is defined using the interpolate method of spinAnim, which maps values in the range of [0, 0.5, 1] to the output range of [‘0deg’, ’15deg’, ‘0deg’].
This means that when spinAnim is 0, the spin constant will be ‘0deg’, when spinAnim is 0.5, the spin constant will be ’15deg’, and when spinAnim is 1, the spin constant will be ‘0deg’.
The rotate function is defined, which uses the Animated.spring method to animate the spinAnim state.
The toValue argument is set to either 0 or 1 depending on the value of iconPressed, which means that the animation will either rotate the icon back to its original position (when iconPressed is true) or rotate it by 15 degrees (when iconPressed is false).
The bounciness argument is set to 0, which means that the animation will not have any bouncing effect. The useNativeDriver argument is set to true, which means that the animation will be performed using the native driver for better performance.
You will get the following output.
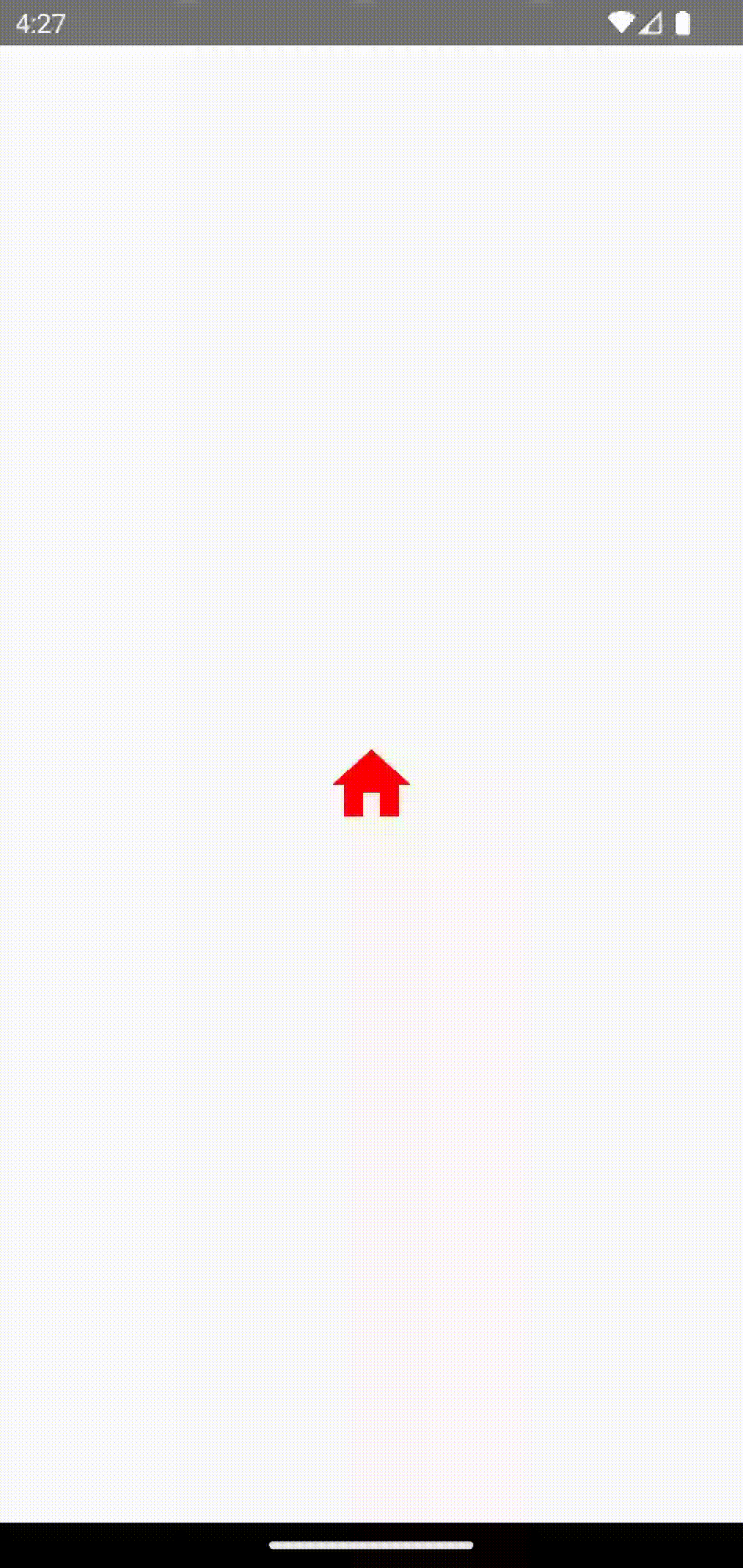
I hope this tutorial react native icon animation is helpful for you.