React Native Text Wrap: How to Manage Long Text
React Native is a powerful framework for building mobile apps. A common challenge developers face is text wrapping. In this blog post, we’ll explore how to handle long text in React Native apps using text-wrapping techniques.
What is Text Wrapping?
Text wrapping is the process of breaking lines so that text fits within a certain space. In React Native, text wraps automatically. But sometimes, you might want to control how it wraps.
How Does React Native Handle Text Wrapping?
Default Wrapping
By default, React Native wraps text inside a <Text>
component. It’s a simple, out-of-the-box feature.
Ellipsis
If the text is too long to fit in one line, you can use the ellipsizeMode
prop. This will show an ellipsis (...
) at the end of the line.
import {Text, View} from 'react-native';
import React from 'react';
const App = () => {
return (
<View>
<Text numberOfLines={1} ellipsizeMode="tail">
This is a very long text. This text will be truncated after the first
line. okay?
</Text>
</View>
);
};
export default App;
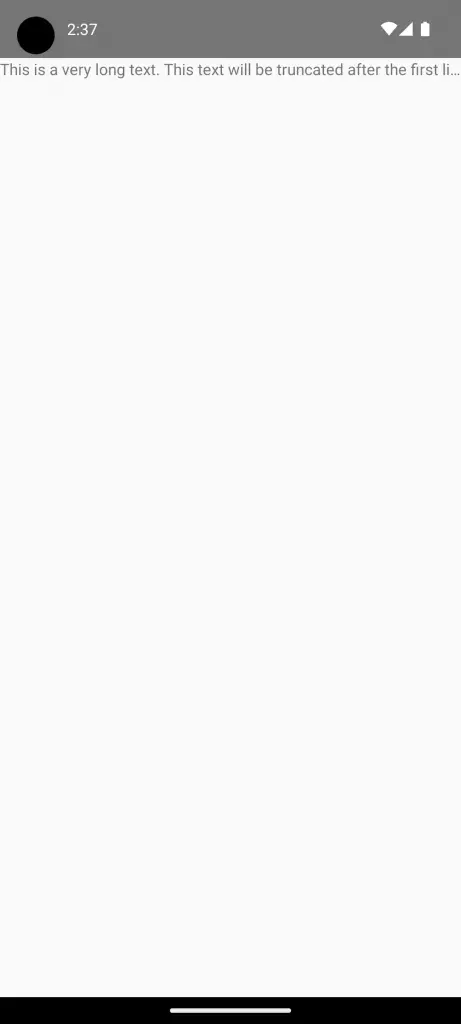
Number of Lines
You can also limit the number of lines using the numberOfLines
prop.
<Text numberOfLines={2}>This is a long text that will be truncated after two lines</Text>
Common Pitfalls
Text Overflow
If the text overflows the container, it might get cut off. So, make sure to size your containers well.
Parent-Child Components
Sometimes, parent components can affect the text wrap. Be mindful of how your <Text>
component is nested.
Text wrapping is an essential feature in React Native. Knowing how to customize it can help you build more versatile mobile apps. React Native offers several ways to manage text wrapping, and understanding these can solve many common UI challenges.