How to Make Images Load Faster in React Native
Most of the time, the react native image component is enough for showing images in your react native app. If your app has so many images to load then you may find issues such as flickering, cache miss, etc with the image component.
So, how to make your images optimized and load faster in react native?
React native fast image library provides you high performant image component with the following features.
- Aggressive caching
- Authorization headers
- GIF
- prioritize and preload images
You can install the library using any of the commands given below.
yarn add react-native-fast-image
or
npm install react-native-fast-image
For ios, execute the following command too.
cd ios && pod install
Following is the example which uses the FastImage component of react native fast image library.
import React from 'react';
import {StyleSheet, View} from 'react-native';
import FastImage from 'react-native-fast-image';
const App = () => {
return (
<View style={styles.container}>
<FastImage
style={styles.image}
source={{
uri: 'https://cdn.pixabay.com/photo/2014/05/26/13/32/butterfly-354528_1280.jpg',
headers: {Authorization: 'default'},
priority: FastImage.priority.normal,
}}
resizeMode={FastImage.resizeMode.contain}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
image: {
width: 350,
height: 200,
},
});
export default App;
Following is the output of the example.
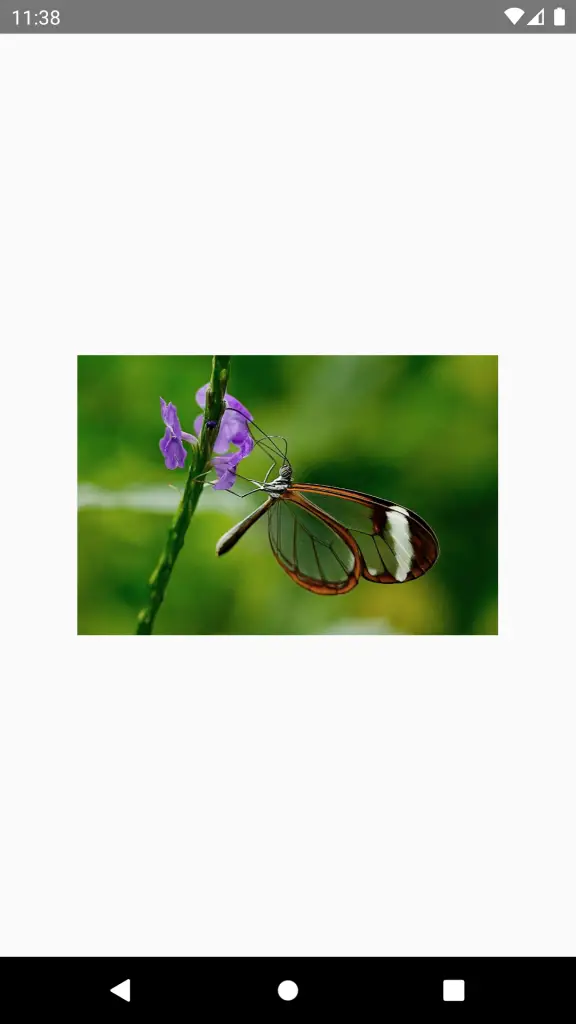
That’s how you make images load faster in react native. I hope this tutorial helped you.