How to Request Multiple Permissions for Android in React Native
Android apps need to ask for permission while running. In this blog post, let’s learn how to request multiple permissions at once for Android in react native.
The PermissionsAndroid API is used to request permissions in react native. The API has the requestMultiple method that helps to ask for all permissions at once.
First of all, you have to add the required permissions in the Android Manifest file.
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_CONTACTS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
Then you can request permissions as given below.
const getAllPermissions = async () => {
PermissionsAndroid.requestMultiple([
PermissionsAndroid.PERMISSIONS.CAMERA,
PermissionsAndroid.PERMISSIONS.READ_CONTACTS,
PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE,
]).then(result => {
if (
result['android.permission.CAMERA'] &&
result['android.permission.READ_CONTACTS'] &&
result['android.permission.WRITE_EXTERNAL_STORAGE'] === 'granted'
) {
Alert.alert(
'All permissions granted!',
'Now you can download anything!',
);
} else {
Alert.alert('Permissions denied!', 'You need to give permissions');
}
});
};
You will get the following output.
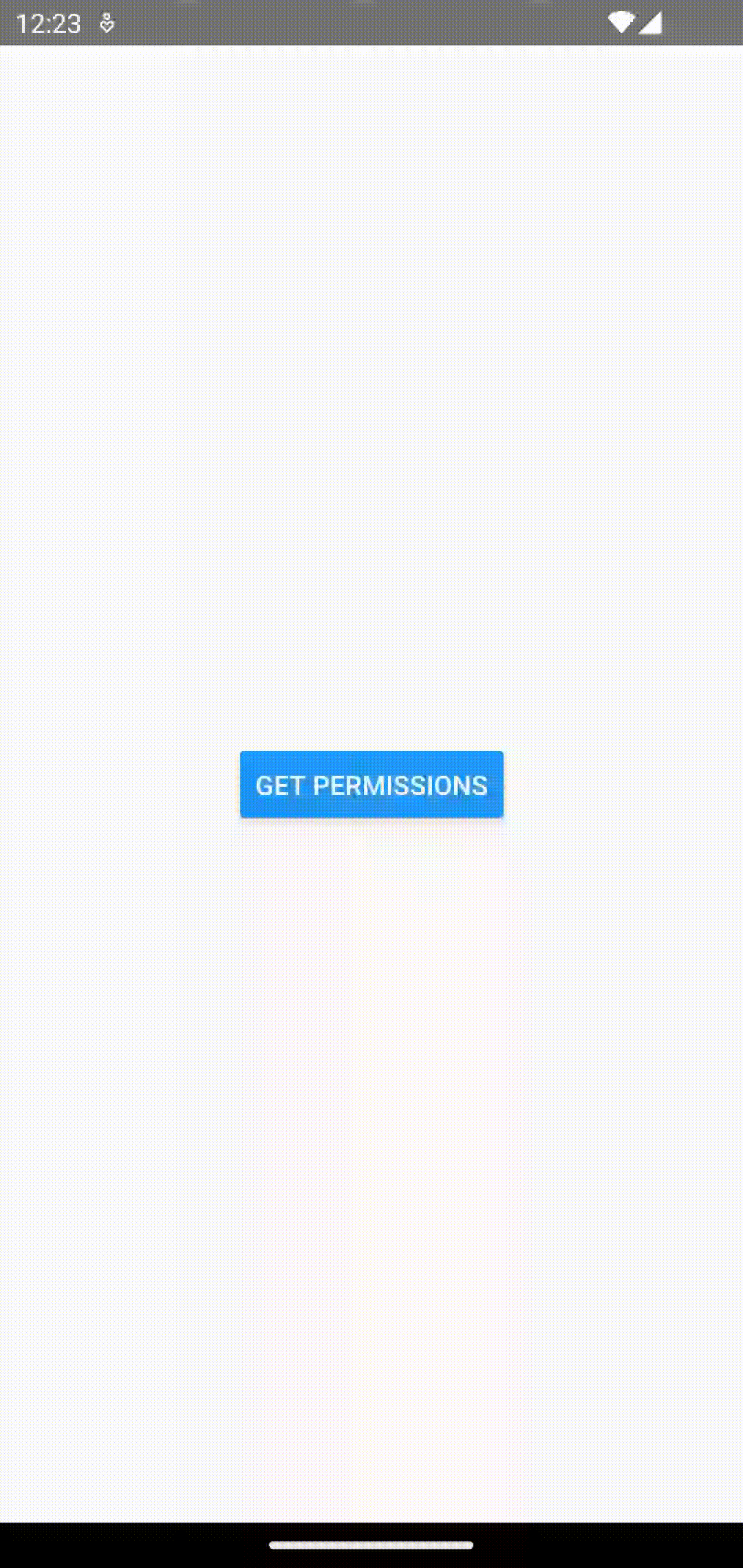
Following is the complete code for react native multiple permission requests.
import {
StyleSheet,
PermissionsAndroid,
Button,
View,
Alert,
} from 'react-native';
import React from 'react';
const App = () => {
const getAllPermissions = async () => {
PermissionsAndroid.requestMultiple([
PermissionsAndroid.PERMISSIONS.CAMERA,
PermissionsAndroid.PERMISSIONS.READ_CONTACTS,
PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE,
]).then(result => {
if (
result['android.permission.CAMERA'] &&
result['android.permission.READ_CONTACTS'] &&
result['android.permission.WRITE_EXTERNAL_STORAGE'] === 'granted'
) {
Alert.alert(
'All permissions granted!',
'Now you can download anything!',
);
} else {
Alert.alert('Permissions denied!', 'You need to give permissions');
}
});
};
return (
<View style={styles.container}>
<Button onPress={getAllPermissions} title="Get Permissions" />
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
That’s how you request multiple permissions at once in react native.