How to add ListView with Auto Scroll to Bottom
ListView is a very important widget in Flutter. It allows us to display lists from data efficiently. In this blog post, let’s learn how to implement the auto scroll to bottom feature in ListView.
The ScrollController class helps us to manage the scrolling of the ListView widget. With it, you can scroll to the bottom or top easily.
The following code snippet allows you to scroll to the bottom of the ListView.
WidgetsBinding.instance.addPostFrameCallback((_) {
if (_scrollController.hasClients) {
_scrollController.animateTo(_scrollController.position.maxScrollExtent,
duration: const Duration(milliseconds: 500),
curve: Curves.fastOutSlowIn);
}
});
But, the tricky part here is, we want to scroll automatically to the bottom of the ListView. Hence the placement of the code is important.
The solution is to call the snippet when the ListView widget is built. See the complete code given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyStatefulWidget(),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
var data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
final ScrollController _scrollController = ScrollController();
@override
Widget build(BuildContext context) {
WidgetsBinding.instance.addPostFrameCallback((_) {
if (_scrollController.hasClients) {
_scrollController.animateTo(_scrollController.position.maxScrollExtent,
duration: const Duration(milliseconds: 500),
curve: Curves.fastOutSlowIn);
}
});
return Scaffold(
appBar: AppBar(
title: const Text('Flutter ListView with Scroll to Bottom'),
),
body: ListView.builder(
controller: _scrollController,
itemCount: data.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 100,
color: Colors.white,
child: Center(
child: Text(
data[index],
)),
);
}),
);
}
}
Following is the output.
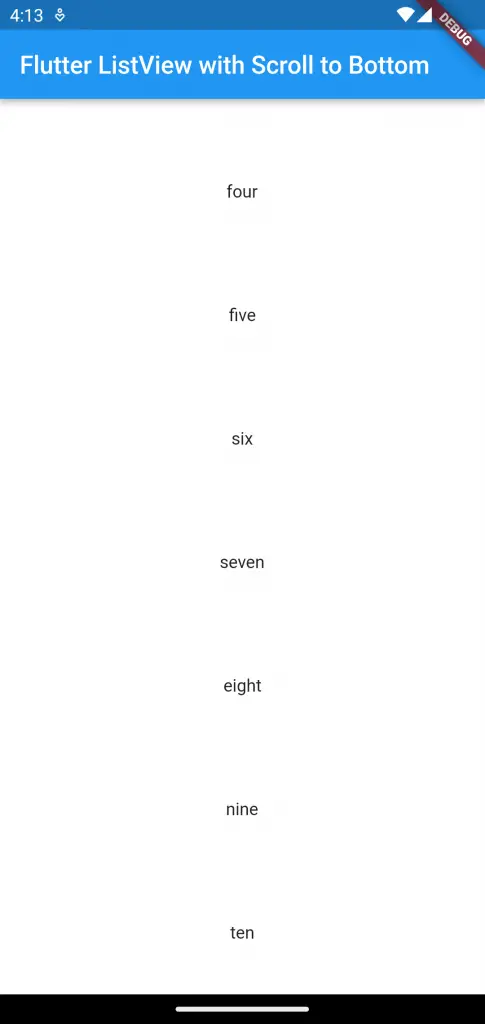
That’s how you add Flutter ListView with auto scroll to the bottom.