How to set AlertDialog Background Color in Flutter
AlertDialog widget is one of the most useful widgets in Flutter. It helps developers to show important messages which may require acknowledgment. In this short Flutter tutorial, let’s learn how to change the AlertDialog background color.
Adding AlertDialog is simple. You can make use of the backgroundColor property of AlertDialog and change the color. See the code snippet given below.
AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
backgroundColor: Colors.yellow,
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
This gives a yellow color background to AlertDialog. The output is given below.
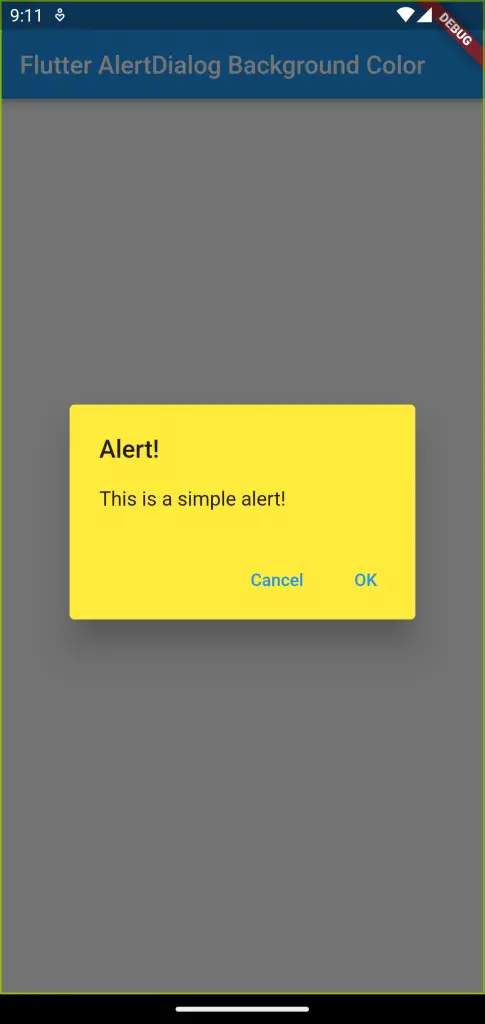
If you want more customizations then it’s better to use custom Dialog instead of AlertDialog.
Following is the complete AlertDialog code for reference. The alert appears only when the TextButton is pressed.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter AlertDialog Background Color'),
),
body: Center(
child: TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
backgroundColor: Colors.yellow,
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
),
child: const Text('Show Dialog'),
)));
}
}
That’s how you change background color of AlertDialog in Flutter.