How to create Full Screen Dialog Alert in Flutter
Sometimes, you may want to interrupt users to show important messages in a full-screen alert. This gains the full attention of the user to acknowledge the matter. Let’s learn how to create a full screen Dialog alert in Flutter.
You can create a custom dialog with the showDialog method but in order to create a full screen alert you should use the showGeneralDialog method.
Following is the complete code to create a full screen modal type alert in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter AlertDialog Background Color'),
),
body: Center(
child: TextButton(
onPressed: () => showGeneralDialog(
context: context,
barrierColor: Colors.cyan, // Background color
barrierDismissible: false,
barrierLabel: ' Full Screen Dialog',
transitionDuration: const Duration(milliseconds: 400),
pageBuilder: (_, __, ___) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const FlutterLogo(
size: 100,
),
const Text(
"Full Screen Dialog in Flutter",
style: TextStyle(fontSize: 18, color: Colors.white),
),
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text("Okay"))
],
);
},
),
child: const Text('Show Dialog'),
)));
}
}
Following is the output.
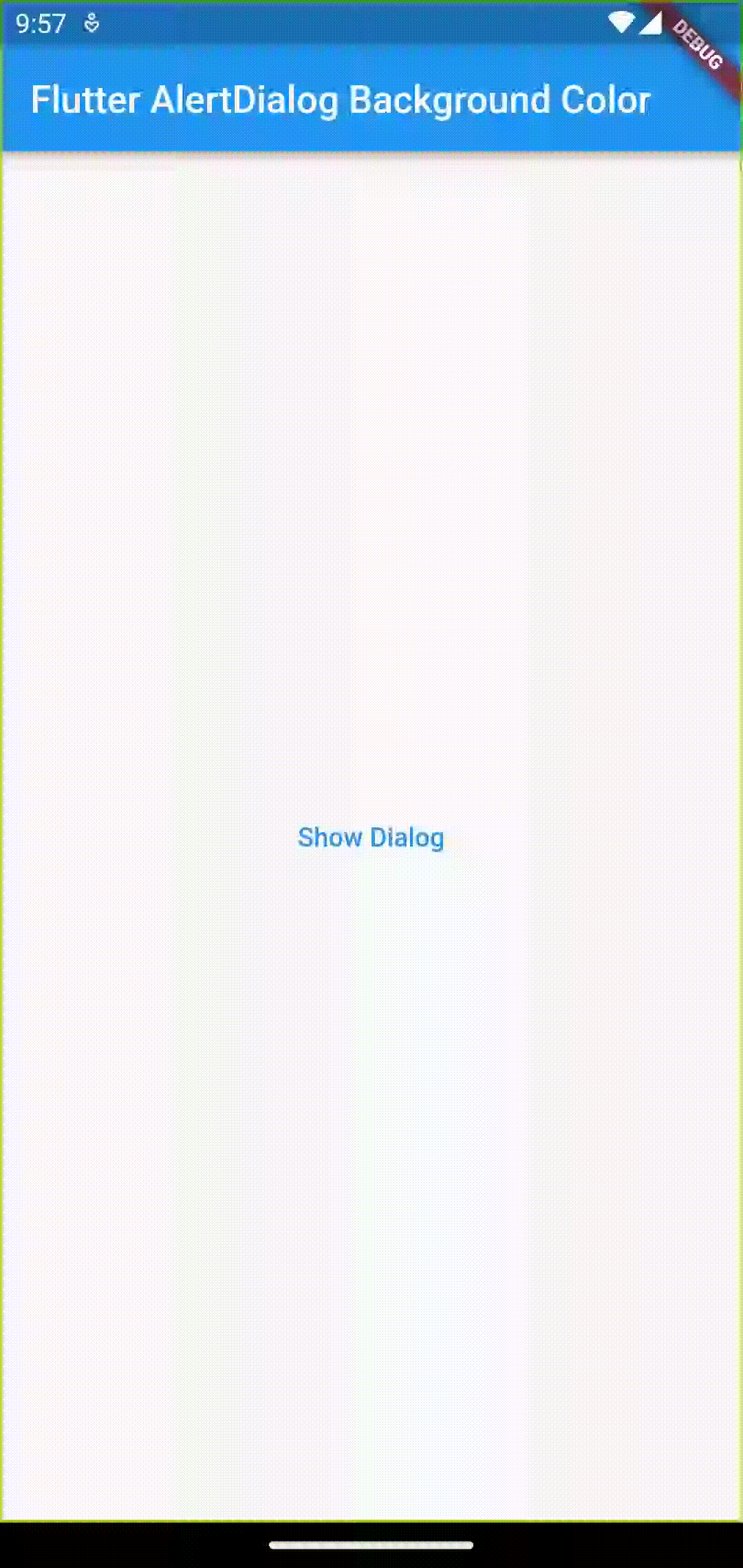
That’s how you add full width dialog in Flutter.