How to Disable Icon Button in Flutter
IconButton is one of the important button widgets in Flutter. It helps you to give button actions to an icon in Flutter. In this blog post, let’s learn how to disable the icon button in Flutter.
An icon button can be added using the IconButton widget. You can disable the IconButton by passing null to the onPressed property. See the code snippet given below.
IconButton(
icon: Icon(Icons.favorite),
color: Colors.red,
iconSize: 50,
onPressed: null,
),
The IconButton color turns to gray and all the button actions will be disabled. See the output given below.
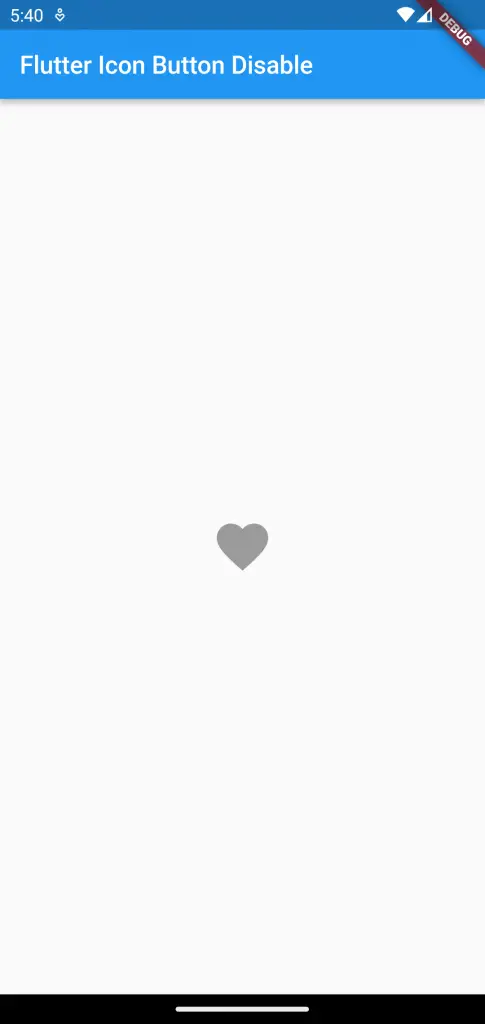
How to change IconButton Disable Color
By default, the icon changes to a gray color when the button is disabled. Sometimes, you may want to customize the disabled color of the IconButton. You should make use of the disabledColor property for this.
See the following code snippet.
IconButton(
icon: Icon(Icons.favorite),
color: Colors.red,
disabledColor: Colors.black,
iconSize: 50,
onPressed: null,
),
The IconButton turns to black color when it is disabled.
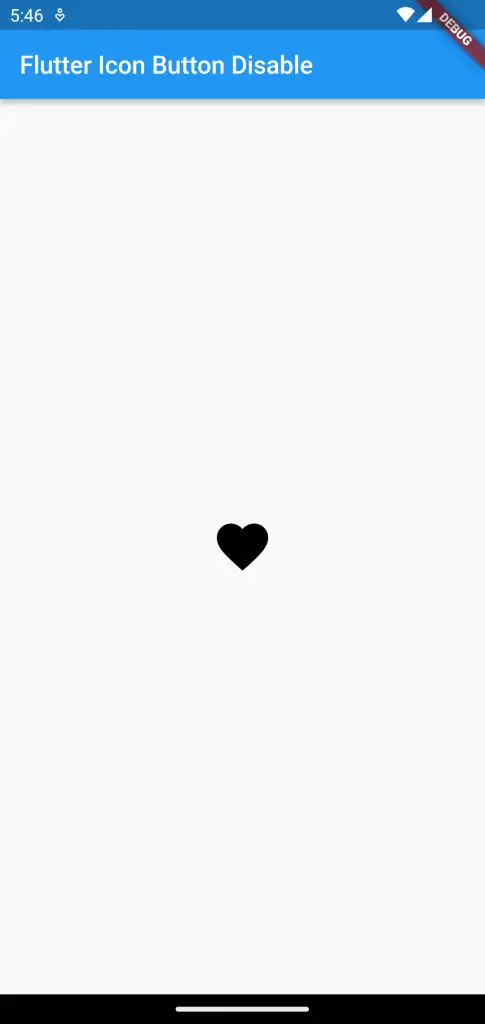
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Icon Button Disable'),
),
body: const Center(
child: IconButton(
icon: Icon(Icons.favorite),
color: Colors.red,
disabledColor: Colors.black,
iconSize: 50,
onPressed: null,
),
));
}
}
That’s how you disable an IconButton and change its disabled color in Flutter.