How to Add a Forward Button to WebView in Flutter
The flutter webview package has a WebView widget that provides an easy way to display web pages within your mobile application. Sometimes you may need to add navigation controls to the WebView, such as a forward button to allow the user to move forward in the web page’s history.
In this blog post, let’s check how to add a WebView with a forward button in Flutter. I expect you to install the flutter webview package into your project.
Using the webview controller goForward method, you can prompt the webview to go to the forward webpage. See the code snippet given below.
IconButton(
icon: const Icon(Icons.arrow_forward),
onPressed: () async {
if (await controller.canGoForward()) {
controller.goForward();
} else {
debugPrint('No history available');
return;
}
},
)
Similar way, you can create a back button using the goBack method. In the following example, we have both buttons added to the AppBar itself.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView example'),
actions: [
IconButton(
icon: const Icon(Icons.arrow_forward),
onPressed: () async {
if (await controller.canGoForward()) {
controller.goForward();
} else {
debugPrint('No history available');
return;
}
},
)
],
leading: IconButton(
icon: const Icon(Icons.arrow_back),
onPressed: () async {
if (await controller.canGoBack()) {
controller.goBack();
} else {
debugPrint('No history available');
return;
}
},
),
),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
],
));
}
}
And you will get the following output.
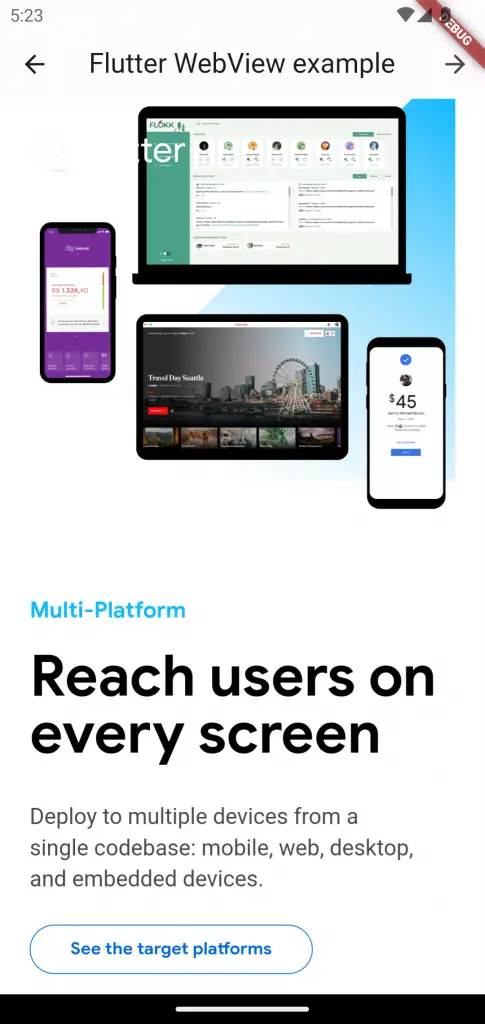
I hope this Flutter tutorial to add a forward button to the WebView is helpful for you. Thank you for reading!