How to Use Flex in Flutter Row
In this blog post, we’re going to tackle an essential concept when working with rows in Flutter—the flex
property. This guide aims to break down the basics and complexities of using flex
with Row
widgets.
Why Flex Matters
First off, why should you even care about the flex
property? The answer is simple. The flex
property allows you to distribute available space among child widgets dynamically, giving you greater control over your layouts. This property is your key to responsive design.
Basic Usage of Flex in Row
Let’s start with a straightforward example to show how flex
works with a Row
.
Row(
children: [
Expanded(
flex: 1,
child: Container(color: Colors.red),
),
Expanded(
flex: 2,
child: Container(color: Colors.green),
),
],
)
In this example, we use the Expanded
widget to wrap around Container
widgets. The flex
property is set to 1
for the first Container
and 2
for the second one. This means that the second Container
will take up twice the space compared to the first one.
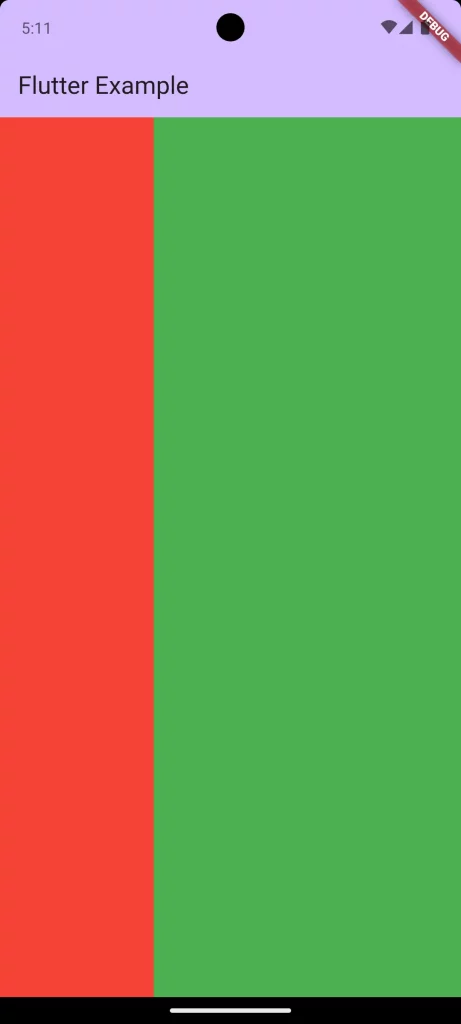
Advanced Flex Example
Now that you’ve got the basics down, let’s check a slightly more complex example.
Row(
children: [
Flexible(
flex: 1,
child: Container(color: Colors.blue),
),
const Spacer(flex: 1),
Flexible(
flex: 2,
child: Container(color: Colors.yellow),
),
],
)
Here, we use both Flexible
and Spacer
widgets in the row. While Flexible
allows the child to take up available space, Spacer
acts as an empty space that can also be adjusted using flex
.
In this example, the Spacer
with a flex
of 1
pushes the containers apart, while the containers themselves adjust their widths based on their flex
values.
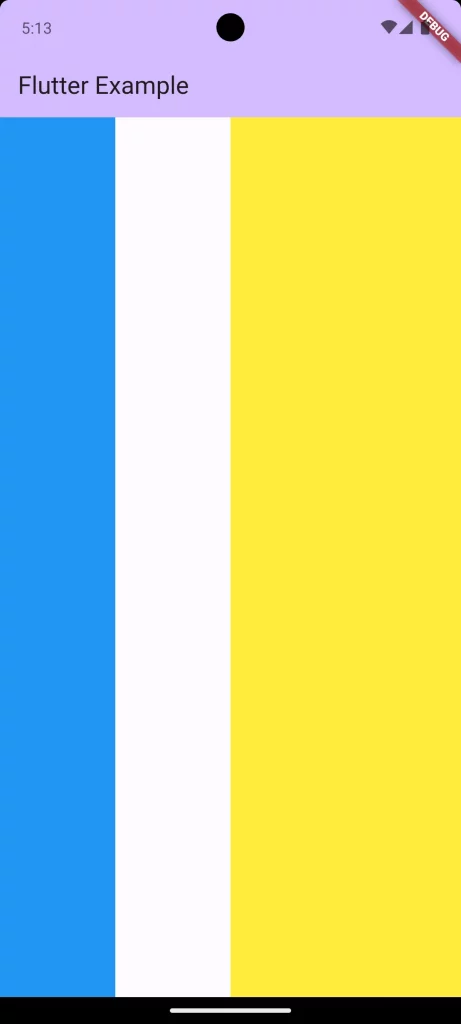
The flex
property in a Flutter Row
offers an array of possibilities for controlling space distribution among child widgets. Whether you are a beginner or an advanced Flutter developer, understanding how to effectively use flex
can significantly up your layout game.