How to add Floating Action Button with Icon and Text
The Floating Action Button (FAB) is one of the commonly used components in material design. Usually, the FAB has icons only. In this tutorial, let’s learn how to add Floating Action Button with icon and text in Flutter.
The FloatingActionButton class has a constructor – FloatingActionButton.extended that can be used to add icon and text as well. We use icon property for the Icon and label property for the Text.
See the code snippet given below.
FloatingActionButton.extended(
onPressed: () {},
icon: const Icon(Icons.edit),
label: const Text('Edit'),
),
You will get the following output of a FAB with icon and text.
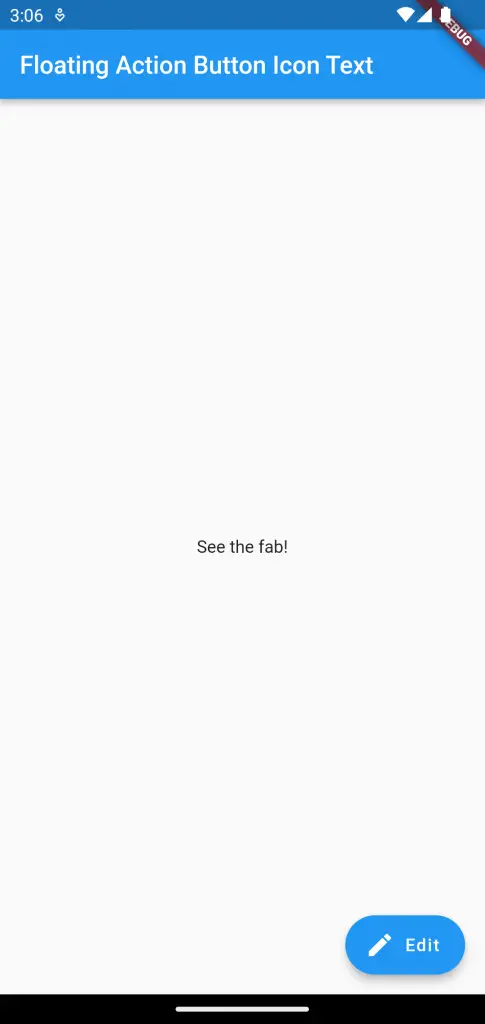
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Icon Text'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton.extended(
onPressed: () {},
icon: const Icon(Icons.edit),
label: const Text('Edit'),
),
);
}
}
That’s how you create Floating Action Button with icon and text in Flutter.