How to Darken an Image in Flutter
Images are an integral part of a mobile app user interface. Sometimes, you may want to show a darkened image in your app. In this blog post, let’s learn how to darken an image in Flutter.
The Image widget comes with many valuable properties. Using the color property and the colorBlendMode property you can easily darken an image.
See the code snippet given below.
Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2',
width: 300,
height: 500,
color: Colors.black45,
colorBlendMode: BlendMode.darken,
),
As you see, we used black45 color, and BlendMode.darken is chosen.
The output is given below.
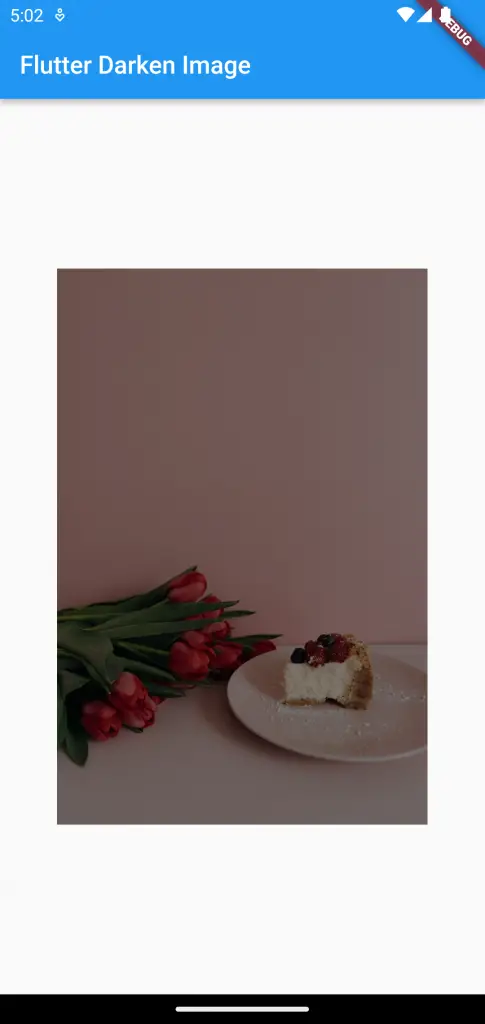
See the following complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Darken Image'),
),
body: Center(
child: Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2',
width: 300,
height: 500,
color: Colors.black45,
colorBlendMode: BlendMode.darken,
),
));
}
}
I hope this Flutter tutorial to darken images is helpful for you.
If you want to change the opacity then see this Flutter Image opacity tutorial.