How to change Selection Color of Text in Flutter
The Text in Flutter is not selectable by default. So you have to choose the SelectableText widget to make the text selectable. In this Flutter tutorial, let’s check how to change the selection color of the text.
The SelectableText widget can be used for single-style text whereas SelectableText.rich constructor should be used for text with multiple styles. In order to change the selection you have to tweak the ThemeData.
You can change cursorColor, selectionColor, and selectionHandleColor using the TextSelectionThemeData.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
textSelectionTheme: const TextSelectionThemeData(
cursorColor: Colors.red,
selectionColor: Colors.yellow,
selectionHandleColor: Colors.black,
)),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Selection Color'),
),
body: const Center(
child: SelectableText(
'Hello, How are you?',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 16),
)));
}
}
Following is the output.
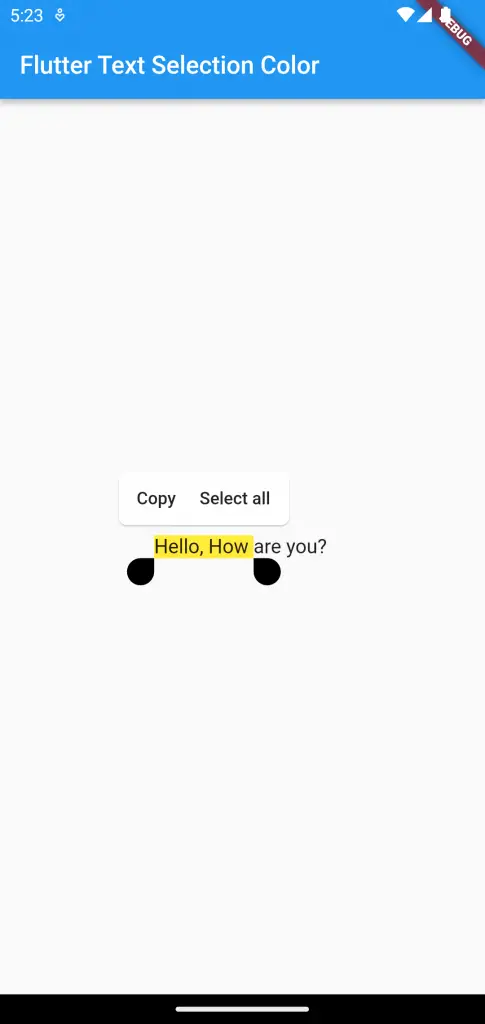
That’s how you change the selection color in Flutter.