How to Remove Floating Action Button Shadow in Flutter
A Floating Action Button or FAB is a button that is intended for actions with high significance. As a UI element, the style of FAB is also important. In this Flutter tutorial, let’s check how to remove the shadow of the Floating Action Button.
By default, FAB has some elevation and that’s the reason for the shadows. You can see it in the image given below.
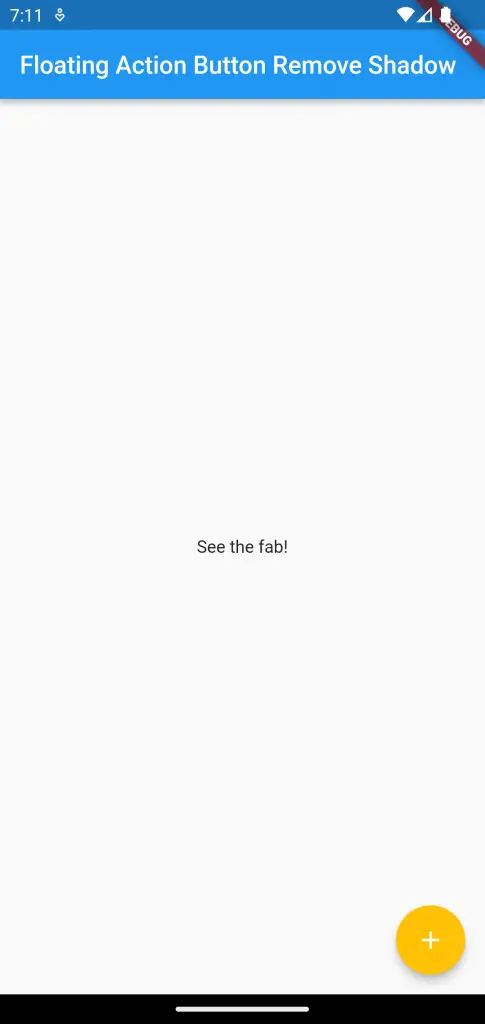
The FloatingActionButton widget has a property named elevation. All you need to do is to set the elevation to zero.
See the code snippet given below.
FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.amber,
elevation: 0,
child: const Icon(Icons.add),
),
Now, the shadows of the Floating Action Button will be removed. Following is the output.
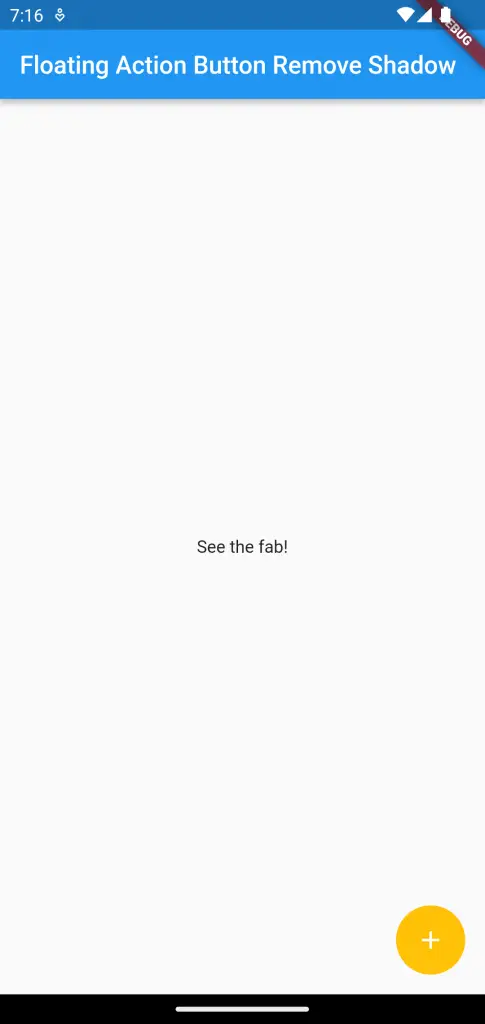
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Remove Shadow'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.amber,
elevation: 0,
child: const Icon(Icons.add),
),
);
}
}
That’s how you remove Floating Action Button shadows in Flutter.