How to change IconButton Splash Color in Flutter
The IconButton is one of the most used button widgets in Flutter. A splash effect occurs when the IconButton is pressed. In this blog post, let’s learn how to change the splash color of the IconButton in Flutter.
The IconButton has many properties for customization. One among them is splashColor. You can use the splashColor property to define a custom color for the splash effect.
See the code snippet given below.
IconButton(
icon: const Icon(Icons.favorite),
color: Colors.red,
splashColor: Colors.blue,
iconSize: 50,
onPressed: () {},
),
You will get the following output with blue color splash effect.
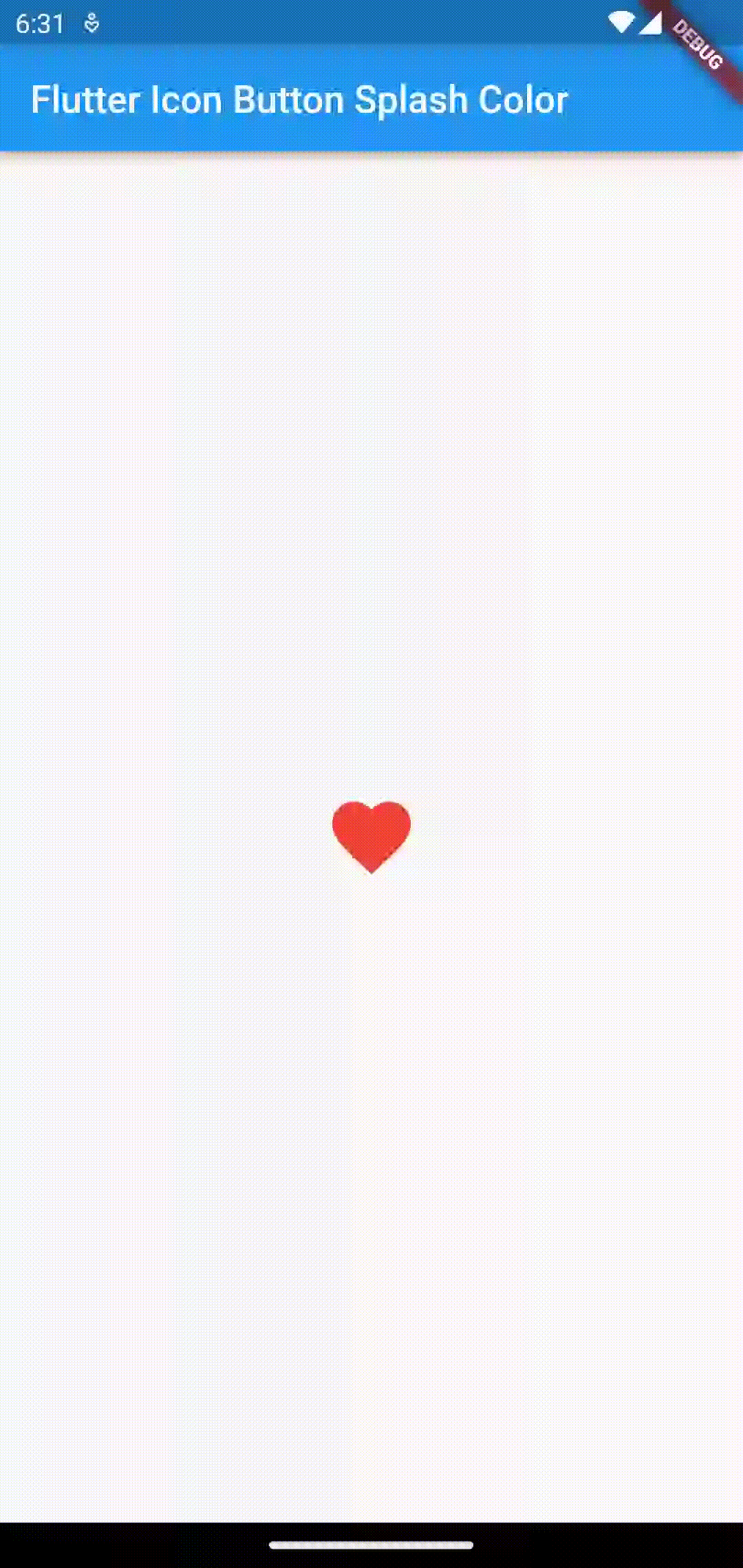
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Icon Button Splash Color'),
),
body: Center(
child: IconButton(
icon: const Icon(Icons.favorite),
color: Colors.red,
splashColor: Colors.blue,
iconSize: 50,
onPressed: () {},
),
));
}
}
That’s how you change the splash color of IconButton in Flutter.