How to Make Row Clickable in Flutter
In building interactive user interfaces with Flutter, there are instances when you want an entire row to be clickable. Whether it’s for a list item, a menu, or a card, making a Row
widget responsive to touches is a common requirement.
Flutter provides several ways to achieve this, ensuring that users can interact with the whole Row
as a singular actionable area. Let’s explore how to make a Row
clickable in Flutter.
GestureDetector
The GestureDetector
widget is a straightforward way to make not just a Row
, but any widget interactive. It offers various callbacks for handling different gestures, such as taps, double taps, and long presses.
Clickable Row with GestureDetector
GestureDetector(
onTap: () {
// Your click event code here
print('Row tapped!');
},
child: const Row(
children: <Widget>[
Icon(Icons.favorite),
Text('Tap me!'),
],
),
)
When the user taps anywhere within the Row
, the onTap
callback is executed, and in this case, it prints a message to the console.
InkWell
Another widget that’s commonly used to make elements clickable in Flutter is InkWell
. It provides the same tap callbacks as GestureDetector
, but also shows a ripple effect upon touch, providing visual feedback, which is standard in material design.
Clickable Row with InkWell
InkWell(
onTap: () {
// Your click event code here
print('Row tapped!');
},
child: const SizedBox(
width: double.infinity,
child: Row(
children: <Widget>[
Icon(Icons.star),
Text('Tap me for a ripple effect!'),
],
),
)
)
The InkWell
must be placed within a Material
widget to show the ripple effect correctly, and Container
ensures the InkWell
stretches to fill its parent.
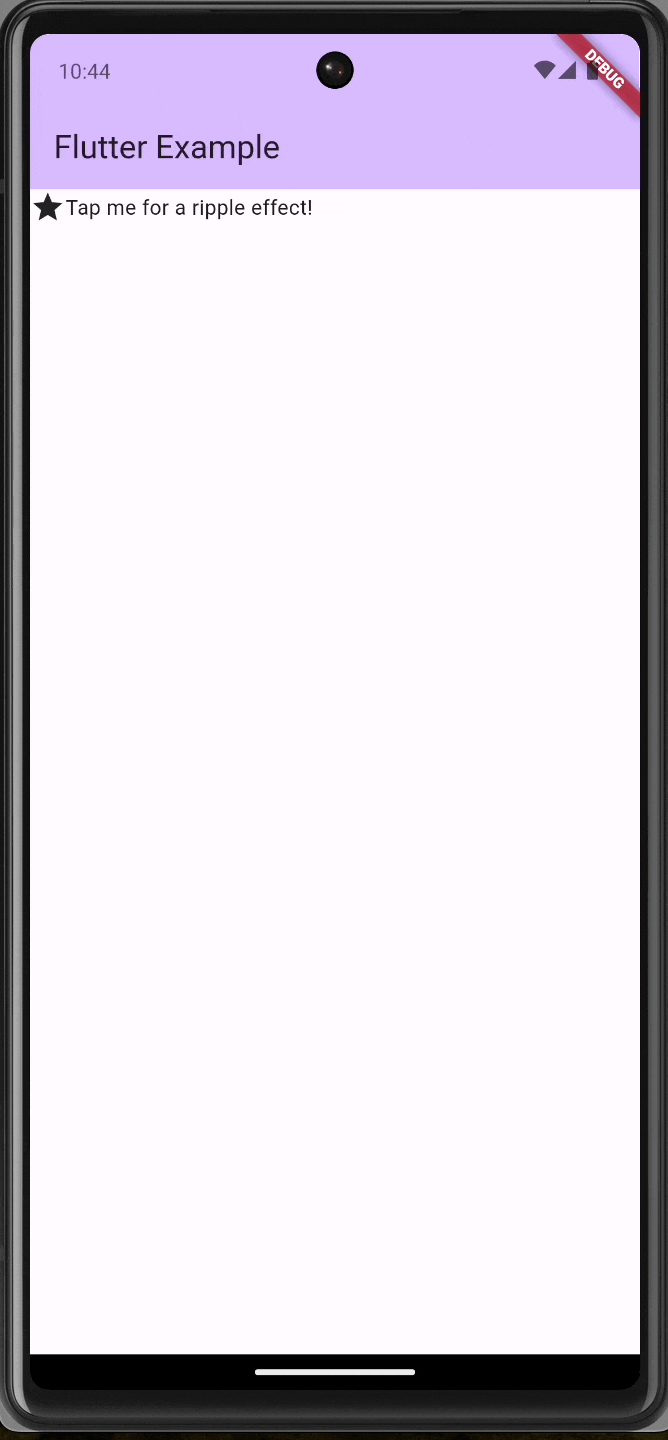
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: InkWell(
onTap: () {
// Your click event code here
print('Row tapped!');
},
child: const SizedBox(
width: double.infinity,
child: Row(
children: <Widget>[
Icon(Icons.star),
Text('Tap me for a ripple effect!'),
],
),
),
));
}
}
Making a Row
clickable in Flutter can be done in various ways, depending on the need for visual feedback and the context in which the row is used. Whether you opt for the simplicity of GestureDetector
or the material feedback of InkWell
, Flutter offers robust solutions to create an interactive and responsive UI.