How to Create Swipeable Screens in Flutter
Swipeable screens are capable of making your mobile app user interface very beautiful. They are mostly used in scenarios such as app introduction sliders. Let’s see how to add swipeable screens in Flutter.
You need PageView widget and PageController class to create swipeable screens in Flutter. The PageController defines which screen you want to show first. See the example code given below.
import 'package:flutter/material.dart';
class HorizontalSwipeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorials',
home: MainScreen()
);
}
}
/// This is the stateless widget that the main application instantiates.
class MainScreen extends StatelessWidget {
final controller = PageController(initialPage: 0);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Horizontal Swipe Example'),
),
body: Center(
child: PageView(controller: controller,
children: <Widget>[
Container(
color: Colors.teal,
),
Container(
color: Colors.yellow,
),
Container(
color: Colors.red,
),
],)
),
);
}
As you noted, there are three container widgets that are given as children to PageView widget. This means each container widget acts as a screen. You will get the following output.
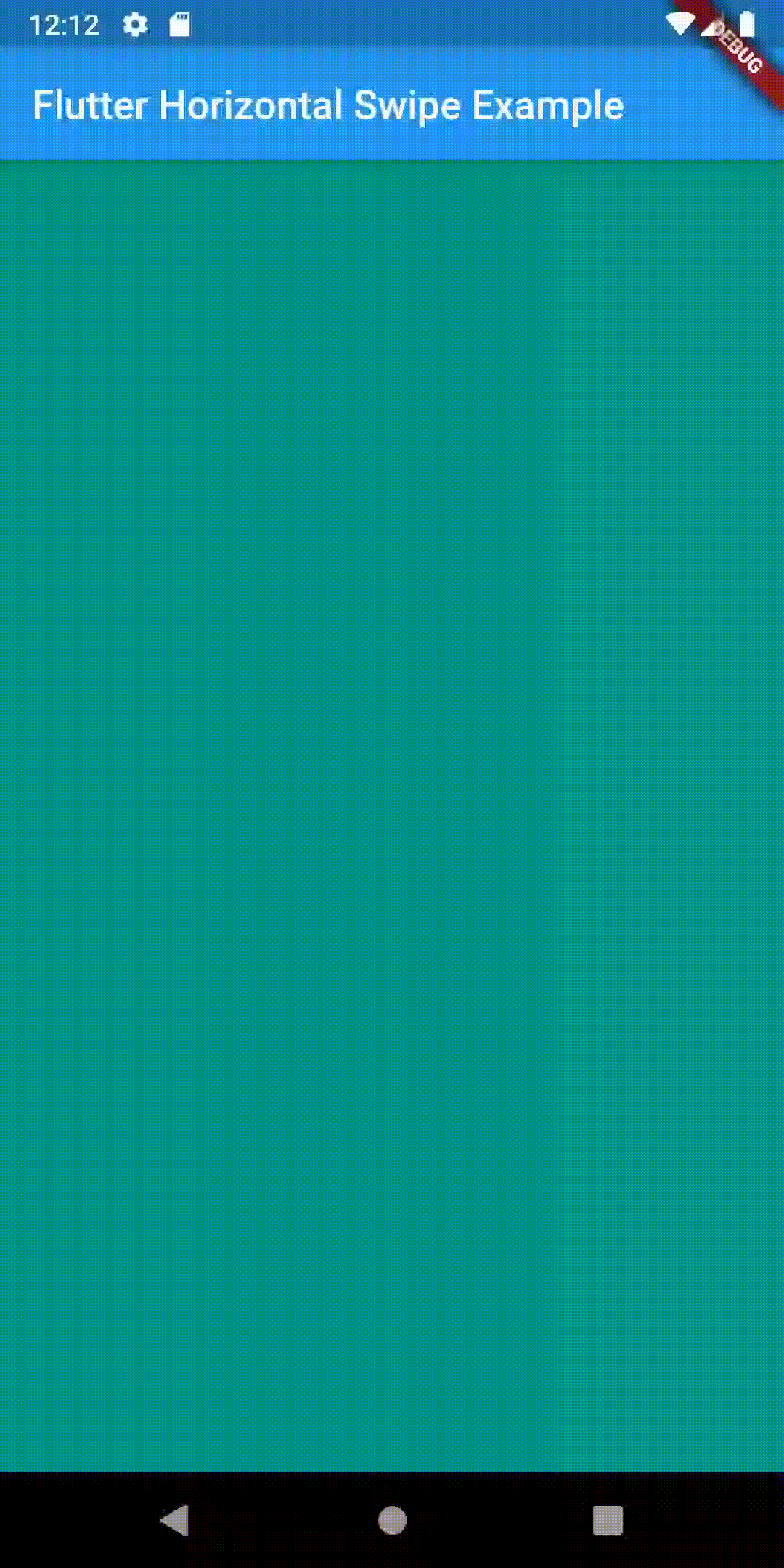
If you want to make the screens swipeable in a vertical direction then use scrollDirection property of PageView widget.
import 'package:flutter/material.dart';
class VerticalSwipeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorials',
home: MainScreen()
);
}
}
/// This is the stateless widget that the main application instantiates.
class MainScreen extends StatelessWidget {
final controller = PageController(initialPage: 0);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Vertical Swipe Example'),
),
body: Center(
child: PageView(controller: controller,
scrollDirection: Axis.vertical,
children: <Widget>[
Container(
color: Colors.teal,
),
Container(
color: Colors.yellow,
),
Container(
color: Colors.red,
),
],)
),
);
}
}
And here’s the output:
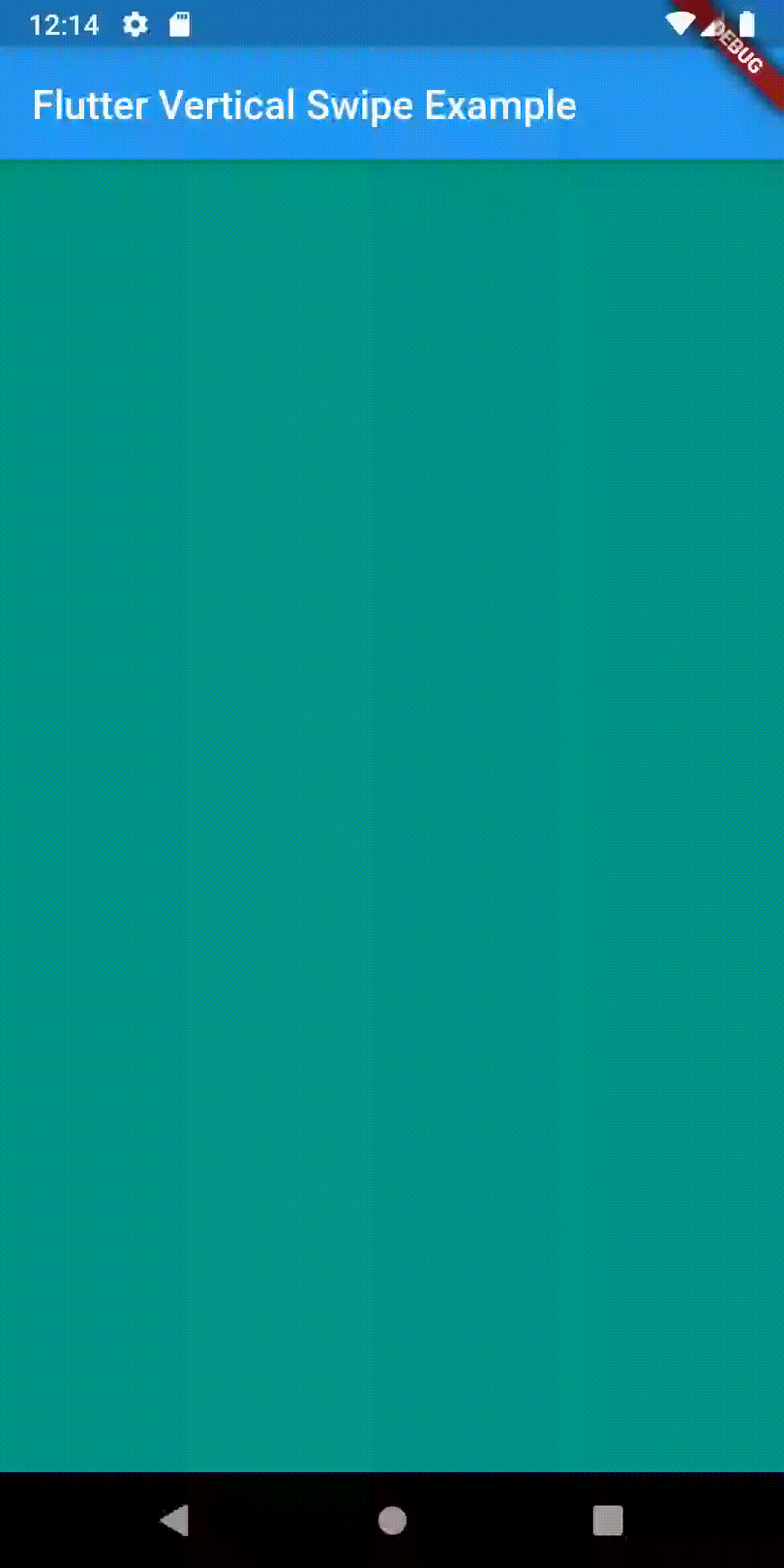