How to create Gradient Text in Flutter
Gradients can help you to create a visually appealing user interface. Let’s learn how to create Text with gradient colors in Flutter. I hope this Flutter tutorial will be useful for you.
You can make use of Paint and ui.Gradient.linear with TextStyle to create beautiful gradient texts. See the following code snippet.
import 'package:flutter/material.dart';
import 'dart:ui' as ui;
Text(
'codingwithrashid.com',
style: TextStyle(
fontSize: 40,
foreground: Paint()
..shader = ui.Gradient.linear(
const Offset(0, 25),
const Offset(100, 0),
<Color>[
Colors.green,
Colors.cyan,
],
)),
)
This is a Text with a linear gradient of two colors- green and cyan. You can set the Offset so that you can adjust two colors for the desired result. The output is given below.
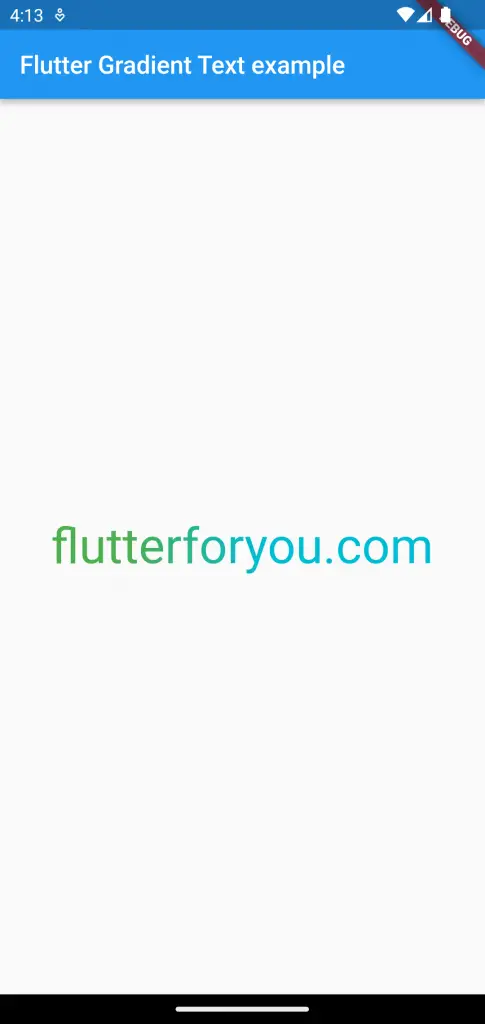
Following is the complete code.
import 'package:flutter/material.dart';
import 'dart:ui' as ui;
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Gradient Text example'),
),
body: Center(
child: Text(
'codingwithrashid.com',
style: TextStyle(
fontSize: 40,
foreground: Paint()
..shader = ui.Gradient.linear(
const Offset(0, 25),
const Offset(100, 0),
<Color>[
Colors.green,
Colors.cyan,
],
)),
)),
);
}
}
That’s how you add gradient text in Flutter.