How to add Icon with Text in Flutter
Icons have an important role in mobile app design. In this blog post, let’s check how to add an icon with text in Flutter.
The Icon widget helps you to add icons to your Flutter project. By default, there’s no property to add a label or text with the icon. Hence, you have to make use of layout widgets such as Column or Row.
See the code snippet given below.
Column(
children: const <Widget>[
Icon(Icons.favorite, color: Colors.red, size: 50),
Text('Favorite')
],
),
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Icon with Text'),
),
body: Center(
child: Column(
children: const <Widget>[
Icon(Icons.favorite, color: Colors.red, size: 50),
Text('Favorite')
],
),
));
}
}
And you will get the following output with icon and text.
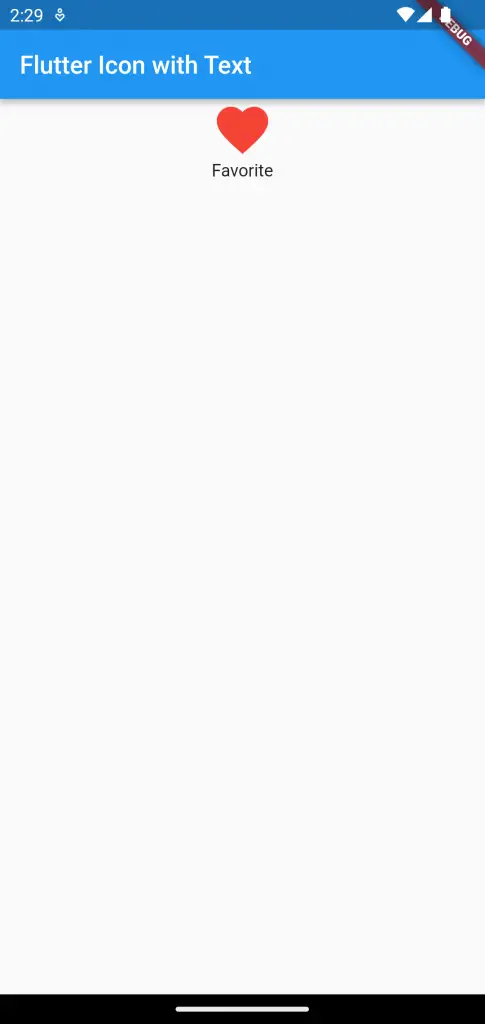
That’s how you add Icon with text in Flutter.